Serializing object with no namespaces using DataContractSerializer(使用 DataContractSerializer 序列化没有命名空间的对象)
问题描述
如何从使用 DataContractSerializer 序列化的对象的 XML 表示中删除 XML 命名空间?
How do I remove XML namespaces from an object's XML representation serialized using DataContractSerializer?
该对象需要序列化为一个非常简单的输出 XML.
That object needs to be serialized to a very simple output XML.
- 最新 &最好的 - 使用 .Net 4 beta 2
- 永远不需要反序列化对象.
- XML 不应有任何 xmlns:...命名空间引用
- 需要支持 Exception 和 ISubObject 的任何子类型.
- 更改原始对象将非常困难.
对象:
[Serializable]
class MyObj
{
string str;
Exception ex;
ISubObject subobj;
}
需要序列化成:
<xml>
<str>...</str>
<ex i:nil="true" />
<subobj i:type="Abc">
<AbcProp1>...</AbcProp1>
<AbcProp2>...</AbcProp2>
</subobj>
</xml>
我使用了这个代码:
private static string ObjectToXmlString(object obj)
{
if (obj == null) throw new ArgumentNullException("obj");
var serializer =
new DataContractSerializer(
obj.GetType(), null, Int32.MaxValue, false, false, null,
new AllowAllContractResolver());
var sb = new StringBuilder();
using (var xw = XmlWriter.Create(sb, new XmlWriterSettings
{
OmitXmlDeclaration = true,
NamespaceHandling = NamespaceHandling.OmitDuplicates,
Indent = true
}))
{
serializer.WriteObject(xw, obj);
xw.Flush();
return sb.ToString();
}
}
来自 这篇文章我采用了一个DataContractResolver,因此不必声明子类型:
From this article I adopted a DataContractResolver so that no subtypes have to be declared:
public class AllowAllContractResolver : DataContractResolver
{
public override bool TryResolveType(Type dataContractType, Type declaredType, DataContractResolver knownTypeResolver, out XmlDictionaryString typeName, out XmlDictionaryString typeNamespace)
{
if (!knownTypeResolver.TryResolveType(dataContractType, declaredType, null, out typeName, out typeNamespace))
{
var dictionary = new XmlDictionary();
typeName = dictionary.Add(dataContractType.FullName);
typeNamespace = dictionary.Add(dataContractType.Assembly.FullName);
}
return true;
}
public override Type ResolveName(string typeName, string typeNamespace, Type declaredType, DataContractResolver knownTypeResolver)
{
return knownTypeResolver.ResolveName(typeName, typeNamespace, declaredType, null) ?? Type.GetType(typeName + ", " + typeNamespace);
}
}
推荐答案
你需要标记你想要序列化的类:
You need to mark the classes you want to serialize with:
[DataContract(Namespace="")]
在这种情况下,数据协定序列化程序不会为您的序列化对象使用任何命名空间.
In that case, the data contract serializer will not use any namespace for your serialized objects.
马克
这篇关于使用 DataContractSerializer 序列化没有命名空间的对象的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:使用 DataContractSerializer 序列化没有命名空间的对象
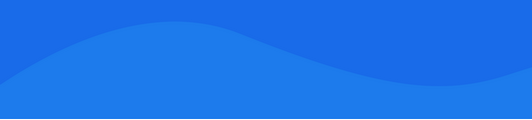
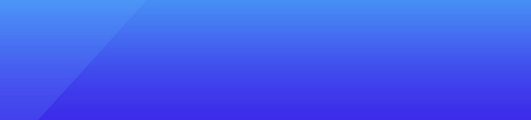
基础教程推荐
- 为什么Flurl.Http DownloadFileAsync/Http客户端GetAsync需要 2022-09-30
- 如何激活MC67中的红灯 2022-01-01
- 将 Office 安装到 Windows 容器 (servercore:ltsc2019) 失败,错误代码为 17002 2022-01-01
- SSE 浮点算术是否可重现? 2022-01-01
- 有没有办法忽略 2GB 文件上传的 maxRequestLength 限制? 2022-01-01
- 将 XML 转换为通用列表 2022-01-01
- 如何在 IDE 中获取 Xamarin Studio C# 输出? 2022-01-01
- MS Visual Studio .NET 的替代品 2022-01-01
- rabbitmq 的 REST API 2022-01-01
- c# Math.Sqrt 实现 2022-01-01