Read from xml files with or without a namespace using XmlDocument(使用 XmlDocument 从有或没有命名空间的 xml 文件中读取)
问题描述
我有一些代码使用 XmlDocument 从具有命名空间的 xml 文件中读取.我的挑战是我现在正在读取硬编码的文件的命名空间,我将它传递给 XmlNamespaceManager.我想为我的方法更灵活一点.从任何类型的 xml 文件中读取.如果它有命名空间,则使用命名空间管理器来读取元素,而无需对命名空间进行硬编码.如果文件没有命名空间,然后去前进,只需解析它.下面是我所做的.
I have some code that reads from xml files with a namespace using XmlDocument.My challenge is that i have the namespace of the file i'm reading hard coded for now and i pass that to the XmlNamespaceManager.I would like for my approach to be a little more flexible.To read from any kind of xml file.If it has a namespace,then use the namespace manager to read the elements without hard coding the namespace.If the file doesn't have a namespace,then go ahead and just parse it.Below is what I've done.
xmldoc = new XmlDocument ();
xmldoc.Load (fileLocation);
XmlNamespaceManager nameSpaceManager = new XmlNamespaceManager(xmldoc.NameTable);
nameSpaceManager.AddNamespace ("ns","http://schemas.sample.data.org/2005");
XmlNodeList nodeList = xmldoc.SelectNodes("/ns:Demo/ns:Items", nameSpaceManager);
if (nodeList != null)
{
foreach (XmlNode childNode in nodeList)
{
string first = childNode.SelectSingleNode ("ns:First", nameSpaceManager).InnerText;
string second= childNode.SelectSingleNode ("ns:Second", nameSpaceManager).InnerText;
string third = childNode.SelectSingleNode ("ns:Third", nameSpaceManager).InnerText;
}
}
这是我正在使用的示例 xml 文件
Here's the sample xml file i'm using
<Demo xmlns:i="http://www.justasample.com" xmlns="http://schemas.sample.data.org/2005">
<Items>
<First>first</First>
<Second>second</Second>
<Third>third</Third>
</Items>
</Demo>
推荐答案
您可以考虑以下选项:
- 确定文档是否包含命名空间,并根据它构造 xpath 查询
- 使用与命名空间无关的 xpath,例如
local-name()
,这将忽略命名空间
选项 1
var xmlDoc = new XmlDocument();
xmlDoc.Load(fileLocation);
//determine whether document contains namespace
var namespaceName = "ns";
var namespacePrefix = string.Empty;
XmlNamespaceManager nameSpaceManager = null;
if (xmlDoc.FirstChild.Attributes != null)
{
var xmlns = xmlDoc.FirstChild.Attributes["xmlns"];
if (xmlns != null)
{
nameSpaceManager = new XmlNamespaceManager(xmlDoc.NameTable);
nameSpaceManager.AddNamespace(namespaceName, xmlns.Value);
namespacePrefix = namespaceName + ":";
}
}
XmlNodeList nodeList = xmlDoc.SelectNodes(string.Format("/{0}Demo/{0}Items",namespacePrefix), nameSpaceManager);
if (nodeList != null)
{
foreach (XmlNode childNode in nodeList)
{
string first = childNode.SelectSingleNode(namespacePrefix + "First", nameSpaceManager).InnerText;
string second = childNode.SelectSingleNode(namespacePrefix + "Second", nameSpaceManager).InnerText;
string third = childNode.SelectSingleNode(namespacePrefix + "Third", nameSpaceManager).InnerText;
}
}
选项 2
XmlNodeList nodeList = xmlDoc.SelectNodes("/*[local-name() = 'Demo']/*[local-name() = 'Items']");
if (nodeList != null)
{
foreach (XmlNode childNode in nodeList)
{
string first = childNode.SelectSingleNode("*[local-name() = 'First']").InnerText;
string second = childNode.SelectSingleNode("*[local-name() = 'Second']").InnerText;
string third = childNode.SelectSingleNode("*[local-name() = 'Third']").InnerText;
}
}
这篇关于使用 XmlDocument 从有或没有命名空间的 xml 文件中读取的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:使用 XmlDocument 从有或没有命名空间的 xml 文件中读取
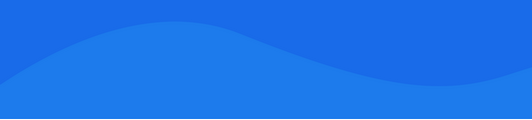
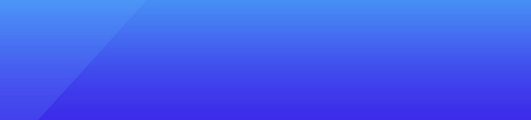
基础教程推荐
- rabbitmq 的 REST API 2022-01-01
- 将 Office 安装到 Windows 容器 (servercore:ltsc2019) 失败,错误代码为 17002 2022-01-01
- 有没有办法忽略 2GB 文件上传的 maxRequestLength 限制? 2022-01-01
- c# Math.Sqrt 实现 2022-01-01
- 为什么Flurl.Http DownloadFileAsync/Http客户端GetAsync需要 2022-09-30
- 如何激活MC67中的红灯 2022-01-01
- SSE 浮点算术是否可重现? 2022-01-01
- MS Visual Studio .NET 的替代品 2022-01-01
- 将 XML 转换为通用列表 2022-01-01
- 如何在 IDE 中获取 Xamarin Studio C# 输出? 2022-01-01