How do you put a Func in a C# attribute (annotation)?(如何将 Func 放入 C# 属性(注释)中?)
问题描述
I have a C# annotation which is :
[AttributeUsage(AttributeTargets.Method)]
public class OperationInfo : System.Attribute {
public enum VisibilityType {
GLOBAL,
LOCAL,
PRIVATE
}
public VisibilityType Visibility { get; set; }
public string Title { get; set; }
public Func<List<string>, List<string>> Func;
public OperationInfo(VisibilityType visibility, string title, Func<List<string>, List<string>> function) {
Visibility = visibility;
Title = title;
Func = function;
}
}
As you can see, there is a property which is a Func and I want to call it dynamically. Basically, I'd like to parse all the methods which have this annotation and then call the Func binded to the annotation.
I'd like to use it like this (this is a simple example of an echo function, which gets a string and return the same string):
[OperationInfo(OperationInfo.VisibilityType.GLOBAL, "echo", IDoEcho)]
public static string DoEcho(string a)
{
return a;
}
[OperationInfo(OperationInfo.VisibilityType.PRIVATE, null, null)]
public static List<string> IDoEcho(List<string> param) {
return new List<string>() { DoEcho(param[0]) };
}
I've got no error in my Annotation class but when it comes to regenerate the entire solution, each time I declare a method with the annotation I've got an error which tells me that I must use literals in an annotation.
I understand there is limitations, but is there any way I can avoid this problem? I know I can use a string instead of a Func, and look dynamically for the function whose name is the same as the string, but I would like not to do so.
Thanks for helping :)
I don't think it is possible, c# only allows you to use Attributes with constant values only.
One way of doing this would be to create a class instead of a func, and then you pass the type of that class into the attribute. You'd then need to instantiate it with reflection and execute a method in it.
So an example would be:
public interface IDoStuff
{
IList<string> Execute();
}
then your attribute would take a type instead of a Func
public OperationInfo(VisibilityType v, string title, Type type)
{
///...
}
you then would create a class that implements your interface and pass it into the attribute. Although this would mean that (at least initially, and without knowing your problem) you will need to create a new class every time you want to return different results.
这篇关于如何将 Func 放入 C# 属性(注释)中?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何将 Func 放入 C# 属性(注释)中?
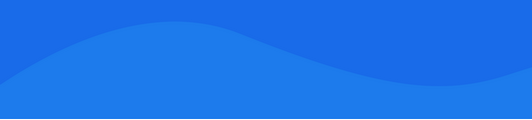
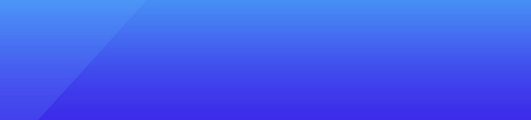
基础教程推荐
- 有没有办法忽略 2GB 文件上传的 maxRequestLength 限制? 2022-01-01
- 将 XML 转换为通用列表 2022-01-01
- c# Math.Sqrt 实现 2022-01-01
- 如何激活MC67中的红灯 2022-01-01
- 将 Office 安装到 Windows 容器 (servercore:ltsc2019) 失败,错误代码为 17002 2022-01-01
- 如何在 IDE 中获取 Xamarin Studio C# 输出? 2022-01-01
- 为什么Flurl.Http DownloadFileAsync/Http客户端GetAsync需要 2022-09-30
- MS Visual Studio .NET 的替代品 2022-01-01
- SSE 浮点算术是否可重现? 2022-01-01
- rabbitmq 的 REST API 2022-01-01