Customizing response serialization in ASP.NET Core MVC(在 ASP.NET Core MVC 中自定义响应序列化)
问题描述
是否可以自定义在 ASP.NET Core MVC 中将类型序列化为响应的方式?
Is it possible to customize the way types are serialized to the response in ASP.NET Core MVC?
在我的特定用例中,我有一个结构 AccountId
,它简单地包裹着一个 Guid
:
In my particular use case I've got a struct, AccountId
, that simply wraps around a Guid
:
public readonly struct AccountId
{
public Guid Value { get; }
// ...
}
当我从动作方法返回它时,不出所料,它会序列化为以下内容:
When I return it from an action method, unsurprisingly, it serializes to the following:
{ "value": "F6556C1D-1E8A-4D25-AB06-E8E244067D04" }
相反,我想自动解开 Value
以便它序列化为纯字符串:
Instead, I'd like to automatically unwrap the Value
so it serializes to a plain string:
"F6556C1D-1E8A-4D25-AB06-E8E244067D04"
可以配置 MVC 来实现这一点吗?
Can MVC be configured to achieve this?
推荐答案
您可以使用 自定义转换器.
在你的情况下,它看起来像这样:
In your case, it would look like this:
[JsonConverter(typeof(AccountIdConverter))]
public readonly struct AccountId
{
public Guid Value { get; }
// ...
}
public class AccountIdConverter : JsonConverter
{
public override bool CanConvert(Type objectType)
=> objectType == typeof(AccountId);
// this converter is only used for serialization, not to deserialize
public override bool CanRead => false;
// implement this if you need to read the string representation to create an AccountId
public override object ReadJson(JsonReader reader, Type objectType, object existingValue, JsonSerializer serializer)
=> throw new NotImplementedException();
public override void WriteJson(JsonWriter writer, object value, JsonSerializer serializer)
{
if (!(value is AccountId accountId))
throw new JsonSerializationException("Expected AccountId object value.");
// custom response
writer.WriteValue(accountId.Value);
}
}
如果您不想使用 JsonConverter
属性,可以在 ConfigureServices
中添加转换器(需要 Microsoft.AspNetCore.Mvc.Formatters.Json代码>):
If you prefer not to use the JsonConverter
attribute, it's possible to add converters in ConfigureServices
(requires Microsoft.AspNetCore.Mvc.Formatters.Json
):
public void ConfigureServices(IServiceCollection services)
{
services
.AddMvc()
.AddJsonOptions(options => {
options.SerializerSettings.Converters.Add(new AccountIdConverter());
});
}
这篇关于在 ASP.NET Core MVC 中自定义响应序列化的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:在 ASP.NET Core MVC 中自定义响应序列化
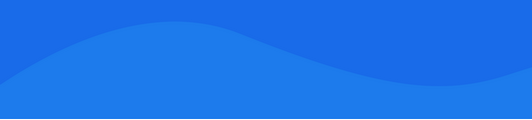
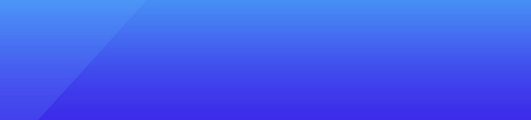
基础教程推荐
- 如何激活MC67中的红灯 2022-01-01
- 将 XML 转换为通用列表 2022-01-01
- 如何在 IDE 中获取 Xamarin Studio C# 输出? 2022-01-01
- SSE 浮点算术是否可重现? 2022-01-01
- rabbitmq 的 REST API 2022-01-01
- 将 Office 安装到 Windows 容器 (servercore:ltsc2019) 失败,错误代码为 17002 2022-01-01
- 有没有办法忽略 2GB 文件上传的 maxRequestLength 限制? 2022-01-01
- MS Visual Studio .NET 的替代品 2022-01-01
- 为什么Flurl.Http DownloadFileAsync/Http客户端GetAsync需要 2022-09-30
- c# Math.Sqrt 实现 2022-01-01