How to return JSon object(如何返回 JSON 对象)
问题描述
我正在使用一个 jQuery 插件,它需要一个具有以下结构的 JSON 对象(我将从数据库中检索值):
I am using a jQuery plugin that need a JSON object with following structure(I will be retrieving the values from database):
{ results: [
{ id: "1", value: "ABC", info: "ABC" },
{ id: "2", value: "JKL", info: "JKL" },
{ id: "3", value: "XYZ", info: "XYZ" }
] }
这是我的课:
public class results
{
int _id;
string _value;
string _info;
public int id
{
get
{
return _id;
}
set
{
_id = value;
}
}
public string value
{
get
{
return _value;
}
set
{
_value = value;
}
}
public string info
{
get
{
return _info;
}
set
{
_info = value;
}
}
}
这是我序列化的方式:
results result = new results();
result.id = 1;
result.value = "ABC";
result.info = "ABC";
string json = JsonConvert.SerializeObject(result);
但这只会返回一行.你能帮我返回一个以上的结果吗?我怎样才能得到上面指定格式的结果?
But this will return only one row. Can you please help me in returning more than one result? How can I get the result in the format specified above?
推荐答案
首先,有 没有 JSON 对象之类的东西.您的问题是 JavaScript 对象文字(请参阅 这里 对差异进行了很好的讨论).以下是您将如何将您拥有的内容序列化为 JSON 的方法:
First of all, there's no such thing as a JSON object. What you've got in your question is a JavaScript object literal (see here for a great discussion on the difference). Here's how you would go about serializing what you've got to JSON though:
我会使用一个匿名类型填充你的 results
类型:
I would use an anonymous type filled with your results
type:
string json = JsonConvert.SerializeObject(new
{
results = new List<Result>()
{
new Result { id = 1, value = "ABC", info = "ABC" },
new Result { id = 2, value = "JKL", info = "JKL" }
}
});
另外,请注意,生成的 JSON 的结果项的 id
类型为 Number
,而不是字符串.我怀疑这会是一个问题,但是在 C# 中将 id
的类型更改为 string
很容易.
Also, note that the generated JSON has result items with id
s of type Number
instead of strings. I doubt this will be a problem, but it would be easy enough to change the type of id
to string
in the C#.
我还会调整您的 results
类型并去掉支持字段:
I'd also tweak your results
type and get rid of the backing fields:
public class Result
{
public int id { get ;set; }
public string value { get; set; }
public string info { get; set; }
}
此外,类通常是 PascalCased
而不是 camelCased
.
Furthermore, classes conventionally are PascalCased
and not camelCased
.
这是从上面的代码生成的 JSON:
Here's the generated JSON from the code above:
{
"results": [
{
"id": 1,
"value": "ABC",
"info": "ABC"
},
{
"id": 2,
"value": "JKL",
"info": "JKL"
}
]
}
这篇关于如何返回 JSON 对象的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何返回 JSON 对象
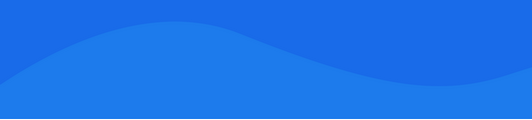
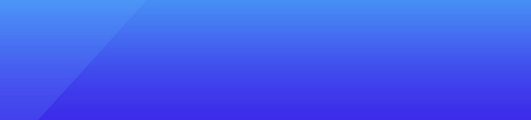
基础教程推荐
- 将 XML 转换为通用列表 2022-01-01
- SSE 浮点算术是否可重现? 2022-01-01
- 有没有办法忽略 2GB 文件上传的 maxRequestLength 限制? 2022-01-01
- c# Math.Sqrt 实现 2022-01-01
- 如何激活MC67中的红灯 2022-01-01
- rabbitmq 的 REST API 2022-01-01
- 为什么Flurl.Http DownloadFileAsync/Http客户端GetAsync需要 2022-09-30
- MS Visual Studio .NET 的替代品 2022-01-01
- 如何在 IDE 中获取 Xamarin Studio C# 输出? 2022-01-01
- 将 Office 安装到 Windows 容器 (servercore:ltsc2019) 失败,错误代码为 17002 2022-01-01