Deserialize nested JSON into C# objects(将嵌套的 JSON 反序列化为 C# 对象)
问题描述
我从如下所示的 API 获取 JSON:
I am getting JSON back from an API that looks like this:
{
"Items": {
"Item322A": [{
"prop1": "string",
"prop2": "string",
"prop3": 1,
"prop4": false
},{
"prop1": "string",
"prop2": "string",
"prop3": 0,
"prop4": false
}],
"Item2B": [{
"prop1": "string",
"prop2": "string",
"prop3": 14,
"prop4": true
}]
},
"Errors": ["String"]
}
我已经尝试了几种方法来在 c# 对象中表示这个 JSON(这里列出的太多了).我已经尝试过使用列表和字典,这是我最近尝试表示它的示例:
I have tried a few approaches to represent this JSON in c# objects (too many to list here). I've tried with lists and dictionaries, here is a recent example of how I've tried to represent it:
private class Response
{
public Item Items { get; set; }
public string[] Errors { get; set; }
}
private class Item
{
public List<SubItem> SubItems { get; set; }
}
private class SubItem
{
public List<Info> Infos { get; set; }
}
private class Info
{
public string Prop1 { get; set; }
public string Prop2 { get; set; }
public int Prop3 { get; set; }
public bool Prop4 { get; set; }
}
这是我用来反序列化 JSON 的方法:
And here is the method I am using to deserialize the JSON:
using (var sr = new StringReader(responseJSON))
using (var jr = new JsonTextReader(sr))
{
var serial = new JsonSerializer();
serial.Formatting = Formatting.Indented;
var obj = serial.Deserialize<Response>(jr);
}
obj
包含 Items
和 Errors
.Items
包含 SubItems
,但 SubItems
是 null
.因此,除了 Errors
实际上没有任何东西被反序列化.
obj
contains Items
and Errors
. And Items
contains SubItems
, but SubItems
is null
. So nothing except for Errors
is actually getting deserialized.
应该很简单,但由于某种原因我无法找出正确的对象表示
It should be simple, but for some reason I can't figure out the correct object representation
推荐答案
"Items"
使用Dictionary
,即:
class Response
{
public Dictionary<string, List<Info>> Items { get; set; }
public string[] Errors { get; set; }
}
class Info
{
public string Prop1 { get; set; }
public string Prop2 { get; set; }
public int Prop3 { get; set; }
public bool Prop4 { get; set; }
}
这假定项目名称 "Item322A"
和 "Item2B"
会因响应而异,并将这些名称作为字典键读取.
This assumes that the item names "Item322A"
and "Item2B"
will vary from response to response, and reads these names in as the dictionary keys.
示例小提琴.
这篇关于将嵌套的 JSON 反序列化为 C# 对象的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:将嵌套的 JSON 反序列化为 C# 对象
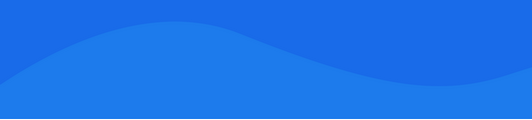
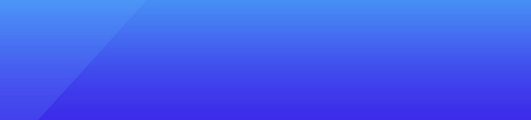
基础教程推荐
- 有没有办法忽略 2GB 文件上传的 maxRequestLength 限制? 2022-01-01
- c# Math.Sqrt 实现 2022-01-01
- 如何在 IDE 中获取 Xamarin Studio C# 输出? 2022-01-01
- 将 Office 安装到 Windows 容器 (servercore:ltsc2019) 失败,错误代码为 17002 2022-01-01
- 为什么Flurl.Http DownloadFileAsync/Http客户端GetAsync需要 2022-09-30
- MS Visual Studio .NET 的替代品 2022-01-01
- SSE 浮点算术是否可重现? 2022-01-01
- 如何激活MC67中的红灯 2022-01-01
- 将 XML 转换为通用列表 2022-01-01
- rabbitmq 的 REST API 2022-01-01