MongoDB and C# Find()(MongoDB 和 C# Find())
问题描述
我有以下代码,我是 mongodb 的新手,我需要帮助来查找集合中的特定元素.
I have the below code and I am new to mongodb, I need help in finding an specific element in the collection.
using MongoDB.Bson;
using MongoDB.Driver;
namespace mongo_console {
public class User {
public ObjectId Id { get; set; }
public string name { get; set; }
public string pwd { get; set; }
}
class Program {
static void Main(string[] args)
{
MongoClient client = new MongoClient();
MongoServer server = client.GetServer();
MongoDatabase db = server.GetDatabase("Users");
MongoCollection<User> collection = db.GetCollection<User>("users");
User user = new User
{
Id = ObjectId.GenerateNewId(),
name = "admin",
pwd = "admin"
};
User user2 = new User
{
Id = ObjectId.GenerateNewId(),
name = "system",
pwd = "system"
};
collection.Save(user);
collection.Save(user2);
/*
* How do I collection.Find() for example using the name
*/
}
}
}
一旦我找到我想要打印的用户,这是可能的还是只会找到返回位置?如果是这样,我该如何打印?
Once I find the user I will like to print it, is that posible or will find only return the position? if so, how do I print it?
我看过一些示例 collection.Find(x => x.something) 但我不知道 x 是什么或意味着什么
I have seen some examples collection.Find(x => x.something) but I do not know what that x is or mean
推荐答案
要查找记录,您可以在 find 中使用 Lambda,例如:
To find a record you could use Lambda in find, for example:
var results = collection.Find(x => x.name == "system").ToList();
或者,您可以使用支持强类型 Lambda 或文本的构建器:
Alternatively you can use Builders which work with strongly typed Lambda or text:
var filter = Builders<User>.Filter.Eq(x => x.name, "system")
或
var filter = Builders<User>.Filter.Eq("name", "system")
然后像上面一样使用find
And then use find as above
// results will be a collection of your documents matching your filter criteria
// Sync syntax
var results = collection.Find(filter).ToList();
// Async syntax
var results = await collection.Find(filter).ToListAsync();
这篇关于MongoDB 和 C# Find()的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:MongoDB 和 C# Find()
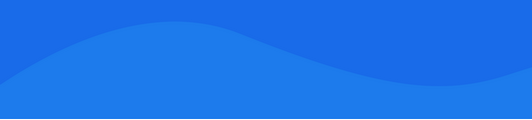
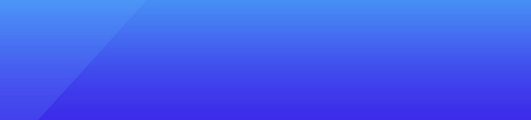
基础教程推荐
- Page.OnAppearing 中的 Xamarin.Forms Page.DisplayAlert 2022-01-01
- 使用 SED 在 XML 标签之间提取值 2022-01-01
- 从 VB6 迁移到 .NET/.NET Core 的最佳策略或工具 2022-01-01
- 当键值未知时反序列化 JSON 2022-01-01
- 我什么时候应该使用 GC.SuppressFinalize()? 2022-01-01
- C# - 将浮点数转换为整数...并根据余数更改整数 2022-01-01
- 创建属性设置器委托 2022-01-01
- 覆盖 Json.Net 中的默认原始类型处理 2022-01-01
- C# - 如何列出发布到 ASPX 页面的变量名称和值 2022-01-01
- 如何使用OpenXML SDK将Excel转换为CSV? 2022-01-01