How does appending to a null string work in C#?(追加到空字符串在 C# 中如何工作?)
问题描述
我很惊讶地看到一个字符串被初始化为 null 然后在生产环境中附加了一些东西的例子.只是闻起来不对.
I was surprised to see an example of a string being initialised to null and then having something appended to it in a production environment. It just smelt wrong.
我确信它会抛出一个空对象异常,但这个大大简化的例子也有效:
I was sure it would have thrown a null object exception but this greatly reduced example also works:
string sample = null;
sample += "test";
// sample equals "test"
*请注意,我发现的原始代码将字符串属性设置为 null 并将其附加到其他地方,因此涉及编译器在编译时优化 null 的答案无关紧要.
有人可以解释为什么这样做没有错误吗?
Can someone explain why this works without error?
根据 Leppie 的回答,我使用 Reflector 来查看 string.Concat 中的内容.现在很明显为什么会发生这种转换(根本没有魔法):
Based on Leppie's answer I used Reflector to see what is inside string.Concat. It is now really obvious why that conversion takes place (no magic at all):
public static string Concat(string str0, string str1)
{
if (IsNullOrEmpty(str0))
{
if (IsNullOrEmpty(str1))
{
return Empty;
}
return str1;
}
if (IsNullOrEmpty(str1))
{
return str0;
}
int length = str0.Length;
string dest = FastAllocateString(length + str1.Length);
FillStringChecked(dest, 0, str0);
FillStringChecked(dest, length, str1);
return dest;
}
**注意:我正在研究的特定实现(在 Microsoft 的 .Net 库中)不会像 C# 标准和大多数答案所建议的那样转换为空字符串,而是使用了一些测试来缩短流程.最终结果和它一样,但你去:)
推荐答案
字符串的 +
运算符只是 string.Concat
的简写,它只是将 null
在连接前将参数转换为空字符串.
the +
operator for strings are just shorthand for string.Concat
which simply turns null
arguments into empty strings before the concatenation.
更新:
string.Concat 的通用版本:
The generalized version of string.Concat:
public static string Concat(params string[] values)
{
int num = 0;
if (values == null)
{
throw new ArgumentNullException("values");
}
string[] array = new string[values.Length];
for (int i = 0; i < values.Length; i++)
{
string text = values[i];
array[i] = ((text == null) ? string.Empty : text);
num += array[i].Length;
if (num < 0)
{
throw new OutOfMemoryException();
}
}
return string.ConcatArray(array, num);
}
这篇关于追加到空字符串在 C# 中如何工作?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:追加到空字符串在 C# 中如何工作?
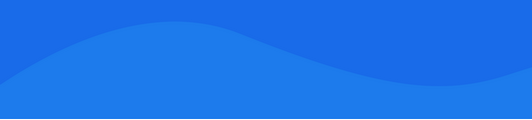
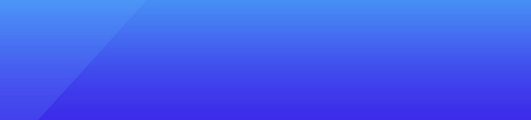
基础教程推荐
- 如何使用OpenXML SDK将Excel转换为CSV? 2022-01-01
- 使用 SED 在 XML 标签之间提取值 2022-01-01
- Page.OnAppearing 中的 Xamarin.Forms Page.DisplayAlert 2022-01-01
- C# - 将浮点数转换为整数...并根据余数更改整数 2022-01-01
- 创建属性设置器委托 2022-01-01
- 我什么时候应该使用 GC.SuppressFinalize()? 2022-01-01
- C# - 如何列出发布到 ASPX 页面的变量名称和值 2022-01-01
- 从 VB6 迁移到 .NET/.NET Core 的最佳策略或工具 2022-01-01
- 当键值未知时反序列化 JSON 2022-01-01
- 覆盖 Json.Net 中的默认原始类型处理 2022-01-01