How to configure dependency injection container with Funclt;T, Resultgt;?(如何使用Funclt;T、Resultgt;配置依赖注入容器?)
问题描述
BusinessAction用于表示用户可以执行的操作。每个操作都与特定实体相关,因此,例如,如果该实体是Order,则业务操作可以是CancelOrder、IssueRefund等。
public abstract class BusinessAction<T>
{
public Guid Id { get; init; }
public Func<T, bool> IsEnabledFor { get; init; }
}
public class CancelOrderAction : BusinessAction<Order>
{
public CancelOrderAction ()
{
Id = Guid.Parse("0e07d05c-6298-4c56-87d7-d2ca339fee1e");
IsEnabledFor = o => o.Status == OrderStatus.Active;
}
}
然后,我需要对与特定类型相关的所有操作进行分组。
public interface IActionRegistry
{
Task<IEnumerable<Guid>> GetEnabledActionIdsForAsync(Guid entityId);
}
public class ActionRegistry<T> : IActionRegistry
where T : BaseEntity
{
private readonly IEnumerable<BusinessAction<T>> _actions;
private readonly IRepository<T> _repository;
public ActionRegistry(IEnumerable<BusinessAction<T>> actions, IRepository<T> repository)
{
_actions = actions;
_repository = repository;
}
public async Task<IEnumerable<Guid>> GetEnabledActionIdsForAsync(Guid entityId)
{
var entity = await _repository.FindByIdAsync(entityId);
return entity == null
? Enumerable.Empty<Guid>()
: _actions.Where(a => a.IsEnabledFor(entity)).Select(a => a.Id);
}
}
最后,还有一个API端点,它接收实体类型(一些后来映射到真正的.NET类型的枚举)和实体的ID。API终结点负责返回为实体的当前状态启用的操作ID。
public class RequestHandler : IRequestHandler<Request, IEnumerable<Guid>>>
{
private readonly Func<Type, IActionRegistry> _registryFactory;
public RequestHandler(Func<Type, IActionRegistry> registryFactory)
{
_registryFactory = registryFactory;
}
public async Task<IEnumerable<Guid>> Handle(Request request, CancellationToken cancellationToken)
{
var type = request.EntityType.GetDotnetType();
var actionRegistry = _registryFactory(type);
var enabledActions = await actionRegistry.GetEnabledActionIdsForAsync(request.EntityId);
return enabledActions;
}
}
问题是:如何在ASP.NET中配置依赖项注入容器(使用默认选项或Autofac),以便可以解析Func<;Type、IActionRegistry&>?
对于ActionRegistry<T>
中的参数,我想我可以这样做:
builder.RegisterAssemblyTypes().AsClosedTypesOf(typeof(BusinessAction<>));
builder.RegisterGeneric(typeof(Repository<>))
.As(typeof(IRepository<>))
.InstancePerLifetimeScope();
但是,我如何配置Func<Type, IActionRegistry>
,以便能够自动将Order
的请求与ActionRegistry<Order>
连接?有没有办法做到这一点,或者我将需要通过编写一些基于类型的开关语句来手动配置工厂(这看起来会是什么样子)?
有没有更好的方法来实现我在这里需要的东西?最终目标是,一旦我有了运行时类型,我就可以获得与该类型相关的业务操作列表以及存储库(这样我就可以从数据库中获取实体)。
推荐答案
您尝试做的事情是可能的,但这不是一件常见的事情,也不是什么神奇的事情,您可以开箱即用。您必须编写代码来实现它。
在我讲到那个之前...从未来的角度来看,如果您的复制品更小,您可能会更快地获得帮助,并更多地关注您的问题。实际上并不需要整个BusinessAction<T>
;不需要RequestHandler
...老实说,你所要做的就是:
public interface IActionRegistry
{
}
public class ActionRegistry<T> : IActionRegistry
{
}
如果其他内容与问题相关,请务必将其包括在内。但在这种情况下,它不是,所以在这里添加它只会使问题更难阅读和回答。我知道,就我个人而言,有时会跳过有很多额外内容的问题,因为一天中只有这么多小时,你知道吗?
不管怎样,下面是您如何做的,以工作示例的形式:
var builder = new ContainerBuilder();
// Register the action registry generic but not AS the interface.
// You can't register an open generic as a non-generic interface.
builder.RegisterGeneric(typeof(ActionRegistry<>));
// Manually build the factory method. Going from reflection
// System.Type to a generic ActionRegistry<Type> is not common and
// not directly supported.
builder.Register((context, parameters) => {
// Capture the lifetime scope or you'll get an exception about
// the resolve operation already being over.
var scope = context.Resolve<ILifetimeScope>();
// Here's the factory method. You can add whatever additional
// enhancements you need, like better error handling.
return (Type type) => {
var closedGeneric = typeof(ActionRegistry<>).MakeGenericType(type);
return scope.Resolve(closedGeneric) as IActionRegistry;
};
});
var container = builder.Build();
// Now you can resolve it and use it.
var factory = container.Resolve<Func<Type, IActionRegistry>>();
var instance = factory(typeof(DivideByZeroException));
Assert.Equal("ActionRegistry`1", instance.GetType().Name);
Assert.Equal("DivideByZeroException", instance.GetType().GenericTypeArguments[0].Name);
这篇关于如何使用Func<;T、Result>;配置依赖注入容器?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何使用Func<;T、Result>;配置依赖注入容器?
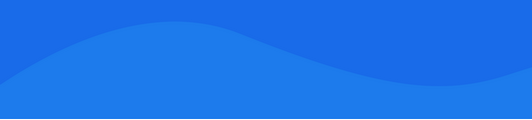
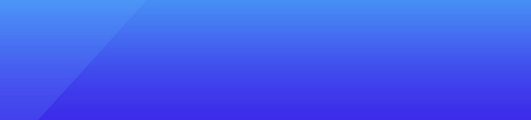
基础教程推荐
- 有没有办法忽略 2GB 文件上传的 maxRequestLength 限制? 2022-01-01
- 将 Office 安装到 Windows 容器 (servercore:ltsc2019) 失败,错误代码为 17002 2022-01-01
- 将 XML 转换为通用列表 2022-01-01
- MS Visual Studio .NET 的替代品 2022-01-01
- c# Math.Sqrt 实现 2022-01-01
- 为什么Flurl.Http DownloadFileAsync/Http客户端GetAsync需要 2022-09-30
- rabbitmq 的 REST API 2022-01-01
- 如何在 IDE 中获取 Xamarin Studio C# 输出? 2022-01-01
- 如何激活MC67中的红灯 2022-01-01
- SSE 浮点算术是否可重现? 2022-01-01