Parameterize SQL query(参数化 SQL 查询)
问题描述
许多关于 C# 中的 SQL 参数的帖子,但我仍然缺少一些东西.我没有收到错误消息,但没有插入数据.缺什么?我有名为 fname、lname、address、city、state 和 zip 的文本框.
Many posts about Parameters in SQL with C# but I am still missing something. I am not getting an error message but no data is inserted. What is missing? I have text boxes named fname, lname, address, city, state and zip.
private void enter_button_Click(object sender, EventArgs e)
{
string first, last, addy, city1, stat, zippy;
first = fname.Text;
SqlParameter firstparam;
firstparam = new SqlParameter();
firstparam.ParameterName = "@first";
firstparam.Value = first;
last = lname.Text;
SqlParameter lastparam;
lastparam = new SqlParameter();
lastparam.ParameterName = "@last";
lastparam.Value = last;
addy = address.Text;
SqlParameter addressparam;
addressparam = new SqlParameter();
addressparam.ParameterName = "@addy";
addressparam.Value = addy;
city1 = city.Text;
SqlParameter cityparam;
cityparam = new SqlParameter();
cityparam.ParameterName = "@city1";
cityparam.Value = city1;
stat = state.Text;
SqlParameter stateparam;
stateparam = new SqlParameter();
stateparam.ParameterName = "@stat";
stateparam.Value = stat;
zippy = zip.Text;
SqlParameter zipparam;
zipparam = new SqlParameter();
zipparam.ParameterName = "@zippy";
zipparam.Value = zippy;
try
{
Validate(fname);
Validate(lname);
Validate(city);
Validate(state);
}
catch (Exception ex)
{
throw new Exception(ex.ToString(), ex);
}
try
{
exValidate(address);
}
catch (Exception ex1)
{
throw new Exception(ex1.ToString(), ex1);
}
try
{
numValidate(zip);
}
catch (Exception ex2)
{
throw new Exception(ex2.ToString(), ex2);
}
string connection = "Data Source=TX-MANAGER;Initial Catalog=Contacts;Integrated Security=True";
var sqlstring = string.Format("INSERT INTO Contacts ([First] ,[Last] ,[Address] ,[City] ,[State],[ZIP]) VALUES {0}, {1}, {2}, {3}, {4}, {5})", @first, @last, @addy, @city1, @stat, @zippy);
SqlConnection conn = new SqlConnection(connection);
SqlCommand comm = new SqlCommand();
comm.CommandText = sqlstring;
try
{
conn.Open();
//SqlTransaction trans = conn.BeginTransaction();
//comm.Transaction = trans;
comm.Parameters.Add("@first", SqlDbType.Text);
comm.Parameters.Add("@last", SqlDbType.Text);
comm.Parameters.Add("@addy", SqlDbType.Text);
comm.Parameters.Add("@city1", SqlDbType.Text);
comm.Parameters.Add("@stat", SqlDbType.Text);
comm.Parameters.Add("@zippy", SqlDbType.SmallInt);
}
catch (Exception commex)
{
throw new Exception(commex.ToString(), commex);
}
conn.Close();
}
所以我改成了这个,但仍然没有任何反应.
So I changed to this and still nothing happens.
string connection = "Data Source=TX-MANAGER;Initial Catalog=Contacts;Integrated Security=True";
var sqlstring = string.Format("INSERT INTO Contacts ([First] ,[Last] ,[Address] ,[City] ,[State],[ZIP]) VALUES {0}, {1}, {2}, {3}, {4}, {5})", @first, @last, @addy, @city1, @stat, @zippy);
SqlConnection conn = new SqlConnection(connection);
SqlCommand comm = conn.CreateCommand();
comm.CommandText = sqlstring;
try
{
conn.Open();
//SqlTransaction trans = conn.BeginTransaction();
//comm.Transaction = trans;
comm.Parameters.AddWithValue("@first", first);
comm.Parameters.AddWithValue("@last", last);
comm.Parameters.AddWithValue("@addy", addy);
comm.Parameters.AddWithValue("@city1", city1);
comm.Parameters.AddWithValue("@stat", stat);
comm.Parameters.AddWithValue("@zippy", zippy);
comm.ExecuteNonQuery();
推荐答案
你忘了执行命令 ;)
您也没有使用在方法开始时创建的参数.
you also didn't use the parameters that you created at the beginning of the method.
...
try
{
conn.Open();
//SqlTransaction trans = conn.BeginTransaction();
//comm.Transaction = trans;
comm.Parameters.Add(firstparam);
comm.Parameters.Add(lastparam);
comm.Parameters.Add(addressparam);
comm.Parameters.Add(cityparam);
comm.Parameters.Add(stateparam);
comm.Parameters.Add(zipparam);
// This is what you forgot:
comm.ExecuteNonQuery();
}
...
顺便说一句,不要这样做:
BTW, don't do things like that:
catch (Exception ex1)
{
throw new Exception(ex1.ToString(), ex1);
}
没用,它只是增加了一个新级别的异常,没有添加任何有用的东西.让异常在堆栈中冒泡,直到它到达一个真正有用的 catch 块.
It's useless, it just adds a new level of exception without adding anything useful. Just let the exception bubble up the stack until it reaches a catch block that actually does something useful.
这篇关于参数化 SQL 查询的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:参数化 SQL 查询
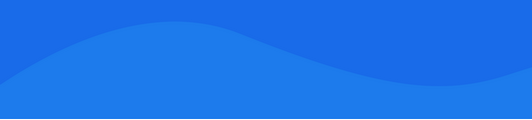
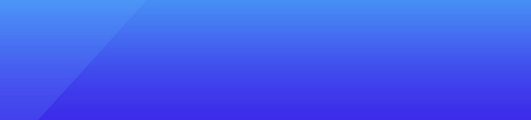
基础教程推荐
- 如何在 IDE 中获取 Xamarin Studio C# 输出? 2022-01-01
- MS Visual Studio .NET 的替代品 2022-01-01
- 将 XML 转换为通用列表 2022-01-01
- 将 Office 安装到 Windows 容器 (servercore:ltsc2019) 失败,错误代码为 17002 2022-01-01
- c# Math.Sqrt 实现 2022-01-01
- 如何激活MC67中的红灯 2022-01-01
- rabbitmq 的 REST API 2022-01-01
- 有没有办法忽略 2GB 文件上传的 maxRequestLength 限制? 2022-01-01
- SSE 浮点算术是否可重现? 2022-01-01
- 为什么Flurl.Http DownloadFileAsync/Http客户端GetAsync需要 2022-09-30