How to update a record using sequelize for node?(如何使用节点的 sequelize 更新记录?)
问题描述
我正在使用 NodeJS、express、express-resource 和 Sequelize 创建一个 RESTful API,用于管理存储在 MySQL 数据库中的数据集.
I'm creating a RESTful API with NodeJS, express, express-resource, and Sequelize that is used to manage datasets stored in a MySQL database.
我正在尝试弄清楚如何使用 Sequelize 正确更新记录.
I'm trying to figure out how to properly update a record using Sequelize.
我创建了一个模型:
module.exports = function (sequelize, DataTypes) {
return sequelize.define('Locale', {
id: {
type: DataTypes.INTEGER,
autoIncrement: true,
primaryKey: true
},
locale: {
type: DataTypes.STRING,
allowNull: false,
unique: true,
validate: {
len: 2
}
},
visible: {
type: DataTypes.BOOLEAN,
defaultValue: 1
}
})
}
然后,在我的资源控制器中,我定义了一个更新操作.
Then, in my resource controller, I define an update action.
在这里,我希望能够更新 id 与 req.params
变量匹配的记录.
In here I want to be able to update the record where the id matches a req.params
variable.
首先我建立一个模型,然后我使用 updateAttributes
方法来更新记录.
First I build a model and then I use the updateAttributes
method to update the record.
const Sequelize = require('sequelize')
const { dbconfig } = require('../config.js')
// Initialize database connection
const sequelize = new Sequelize(dbconfig.database, dbconfig.username, dbconfig.password)
// Locale model
const Locales = sequelize.import(__dirname + './models/Locale')
// Create schema if necessary
Locales.sync()
/**
* PUT /locale/:id
*/
exports.update = function (req, res) {
if (req.body.name) {
const loc = Locales.build()
loc.updateAttributes({
locale: req.body.name
})
.on('success', id => {
res.json({
success: true
}, 200)
})
.on('failure', error => {
throw new Error(error)
})
}
else
throw new Error('Data not provided')
}
现在,这实际上并没有像我期望的那样产生更新查询.
Now, this does not actually produce an update query as I would expect.
而是执行插入查询:
INSERT INTO `Locales`(`id`, `locale`, `createdAt`, `updatedAt`, `visible`)
VALUES ('1', 'us', '2011-11-16 05:26:09', '2011-11-16 05:26:15', 1)
所以我的问题是:使用 Sequelize ORM 更新记录的正确方法是什么?
So my question is: What is the proper way to update a record using Sequelize ORM?
推荐答案
我没有用过 Sequelize,但是在阅读它的文档,很明显你正在实例化一个新对象,这就是为什么 Sequelize在数据库中插入一条新记录.
I have not used Sequelize, but after reading its documentation, it's obvious that you are instantiating a new object, that's why Sequelize inserts a new record into the db.
首先您需要搜索该记录,获取它,然后才更改其属性和 更新,例如:
First you need to search for that record, fetch it and only after that change its properties and update it, for example:
Project.find({ where: { title: 'aProject' } })
.on('success', function (project) {
// Check if record exists in db
if (project) {
project.update({
title: 'a very different title now'
})
.success(function () {})
}
})
这篇关于如何使用节点的 sequelize 更新记录?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何使用节点的 sequelize 更新记录?
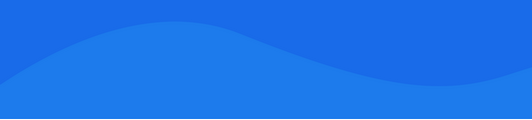
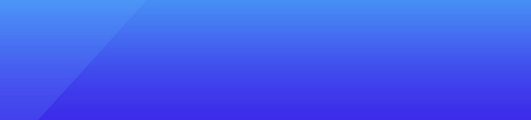
基础教程推荐
- 如何在 SQL Server 的嵌套过程中处理事务? 2021-01-01
- Sql Server 字符串到日期的转换 2021-01-01
- ERROR 2006 (HY000): MySQL 服务器已经消失 2021-01-01
- 将数据从 MS SQL 迁移到 PostgreSQL? 2022-01-01
- 使用pyodbc“不安全"的Python多处理和数据库访问? 2022-01-01
- SQL Server 2016更改对象所有者 2022-01-01
- SQL Server 中单行 MERGE/upsert 的语法 2021-01-01
- 在 VB.NET 中更新 SQL Server DateTime 列 2021-01-01
- SQL Server:只有 GROUP BY 中的最后一个条目 2021-01-01
- 无法在 ubuntu 中启动 mysql 服务器 2021-01-01