LibGDX - how do I make my menu screen switch to the Game screen?(LibGDX - 如何让我的菜单屏幕切换到游戏屏幕?)
问题描述
当我运行应用程序时,我的菜单屏幕显示,但是当我单击屏幕开始玩游戏时,游戏开始玩,但菜单屏幕仍然覆盖游戏.我知道这一点是因为游戏中有音乐播放.我将来还会添加一个启动画面,但我现在并不担心.我是新来的,所以请尽可能地解释事情.以下是用于实现此目的的 3 个类.
When I run the application my menu screen shows, but when I click the screen to begin playing the game the game begins playing but the menu screen is still their overlaying the game. I know this because the game has music playing. I'm also going to add a splash screen in the future but I'm not concerned about that right now. I'm new at this so please explain things as best as you can. Below are the 3 classes used to make this happen.
public class SlingshotSteve extends Game {
public SpriteBatch batch;
public BitmapFont font;
public void create() {
batch = new SpriteBatch();
//Use LibGDX's default Arial font.
font = new BitmapFont();
this.setScreen(new Menu(this));
}
public void render() {
super.render(); //important!
}
public void dispose() {
batch.dispose();
font.dispose();
}
}
这里是主菜单屏幕
public class Menu implements Screen {
final SlingshotSteve game;
OrthographicCamera camera;
public Menu(final SlingshotSteve gam) {
game = gam;
camera = new OrthographicCamera();
camera.setToOrtho(false, 800, 480);
}
@Override
public void render(float delta) {
Gdx.gl.glClearColor(0, 0, 0.2f, 1);
Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT);
camera.update();
game.batch.setProjectionMatrix(camera.combined);
game.batch.begin();
game.font.draw(game.batch, "Welcome to Slingshot Steve!!! ", 100, 150);
game.font.draw(game.batch, "Tap anywhere to begin!", 100, 100);
game.batch.end();
if (Gdx.input.isTouched()) {
game.setScreen((Screen) new GameScreen(game));
dispose();
}
}
这是游戏画面
public class GameScreen implements Screen {
final SlingshotSteve game;
OrthographicCamera camera;
// Creates our 2D images
SpriteBatch batch;
TextureRegion backgroundTexture;
Texture texture;
GameScreen(final SlingshotSteve gam) {
this.game = gam;
camera = new OrthographicCamera(1280, 720);
batch = new SpriteBatch();
Texture texture = new Texture(Gdx.files.internal("background.jpg"));
backgroundTexture = new TextureRegion(texture, 0, 0, 500, 500);
Music mp3Sound = Gdx.audio.newMusic(Gdx.files.internal("rain.mp3"));
mp3Sound.setLooping(true);
mp3Sound.play();
}
public void render() {
Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT);
camera.update();
batch.setProjectionMatrix(camera.combined);
batch.begin();
batch.draw(backgroundTexture, 0, 0);
batch.end();
}
@Override
public void resize(int width, int height) {
}
@Override
public void pause() {
}
@Override
public void resume() {
}
@Override
public void dispose() {
batch.dispose();
texture.dispose();
}
推荐答案
不确定,但我认为您在 GameScreen
上的 render()
方法没有被调用.您必须实现方法 render(float delta)
使用 delta time 作为参数.
Not sure but I think your render()
method on GameScreen
not called. you must implement method render(float delta)
that use delta time for parameter.
替换
public void render() {
// your code
}
与
public void render(float delta) {
// your code
}
这篇关于LibGDX - 如何让我的菜单屏幕切换到游戏屏幕?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:LibGDX - 如何让我的菜单屏幕切换到游戏屏幕?
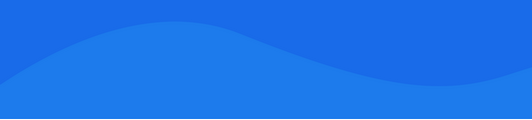
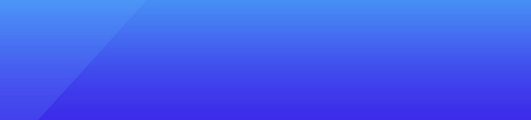
基础教程推荐
- 如何在不安装整个 WTP 包的情况下将 Tomcat 8 添加到 Eclipse Kepler 2022-01-01
- Spring Boot Freemarker从2.2.0升级失败 2022-01-01
- 如何对 HashSet 进行排序? 2022-01-01
- 如何强制对超级方法进行多态调用? 2022-01-01
- 如何使用 Stream 在集合中拆分奇数和偶数以及两者的总和 2022-01-01
- Java 中保存最后 N 个元素的大小受限队列 2022-01-01
- 在螺旋中写一个字符串 2022-01-01
- 由于对所需库 rt.jar 的限制,对类的访问限制? 2022-01-01
- 如何使用 Eclipse 检查调试符号状态? 2022-01-01
- 首次使用 Hadoop,MapReduce Job 不运行 Reduce Phase 2022-01-01