How do I calculate someone#39;s age in Java?(如何在 Java 中计算某人的年龄?)
问题描述
我想在 Java 方法中以 int 形式返回年龄.我现在拥有的是以下内容,其中 getBirthDate() 返回一个 Date 对象(带有出生日期;-)):
I want to return an age in years as an int in a Java method. What I have now is the following where getBirthDate() returns a Date object (with the birth date ;-)):
public int getAge() {
long ageInMillis = new Date().getTime() - getBirthDate().getTime();
Date age = new Date(ageInMillis);
return age.getYear();
}
但是由于 getYear() 已被弃用,我想知道是否有更好的方法来做到这一点?我什至不确定这是否能正常工作,因为我还没有进行单元测试.
But since getYear() is deprecated I'm wondering if there is a better way to do this? I'm not even sure this works correctly, since I have no unit tests in place (yet).
推荐答案
JDK 8 让这一切变得简单而优雅:
JDK 8 makes this easy and elegant:
public class AgeCalculator {
public static int calculateAge(LocalDate birthDate, LocalDate currentDate) {
if ((birthDate != null) && (currentDate != null)) {
return Period.between(birthDate, currentDate).getYears();
} else {
return 0;
}
}
}
一个 JUnit 测试来演示它的使用:
A JUnit test to demonstrate its use:
public class AgeCalculatorTest {
@Test
public void testCalculateAge_Success() {
// setup
LocalDate birthDate = LocalDate.of(1961, 5, 17);
// exercise
int actual = AgeCalculator.calculateAge(birthDate, LocalDate.of(2016, 7, 12));
// assert
Assert.assertEquals(55, actual);
}
}
现在每个人都应该使用 JDK 8.所有早期版本均已结束其支持生命周期.
Everyone should be using JDK 8 by now. All earlier versions have passed the end of their support lives.
这篇关于如何在 Java 中计算某人的年龄?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何在 Java 中计算某人的年龄?
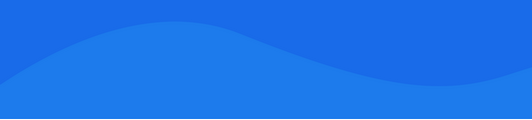
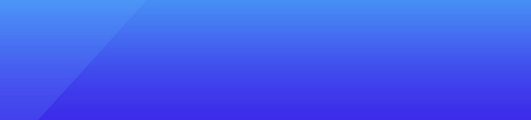
基础教程推荐
- 如何强制对超级方法进行多态调用? 2022-01-01
- Spring Boot Freemarker从2.2.0升级失败 2022-01-01
- 如何使用 Eclipse 检查调试符号状态? 2022-01-01
- 由于对所需库 rt.jar 的限制,对类的访问限制? 2022-01-01
- Java 中保存最后 N 个元素的大小受限队列 2022-01-01
- 如何使用 Stream 在集合中拆分奇数和偶数以及两者的总和 2022-01-01
- 如何对 HashSet 进行排序? 2022-01-01
- 如何在不安装整个 WTP 包的情况下将 Tomcat 8 添加到 Eclipse Kepler 2022-01-01
- 在螺旋中写一个字符串 2022-01-01
- 首次使用 Hadoop,MapReduce Job 不运行 Reduce Phase 2022-01-01