Java, Using Iterator to search an ArrayList and delete matching objects(Java,使用迭代器搜索 ArrayList 并删除匹配的对象)
问题描述
基本上,用户提交一个字符串,迭代器在 ArrayList 中搜索该字符串.找到后,迭代器将删除包含该字符串的对象.
Basically, the user submits a String which the Iterator searches an ArrayList for. When found the Iterator will delete the object containing the String.
因为这些对象中的每一个都包含两个字符串,所以我很难将这些行写成一个.
Because each of these objects contain two Strings, I am finding trouble writing these lines as one.
Friend current = it.next();
String currently = current.getFriendCaption();
感谢您的帮助!
推荐答案
你不需要它们在一行,只要在匹配时使用 remove
删除一个项目:
You don't need them on one line, just use remove
to remove an item when it matches:
Iterator<Friend> it = list.iterator();
while (it.hasNext()) {
if (it.next().getFriendCaption().equals(targetCaption)) {
it.remove();
// If you know it's unique, you could `break;` here
}
}
完整演示:
import java.util.*;
public class ListExample {
public static final void main(String[] args) {
List<Friend> list = new ArrayList<Friend>(5);
String targetCaption = "match";
list.add(new Friend("match"));
list.add(new Friend("non-match"));
list.add(new Friend("match"));
list.add(new Friend("non-match"));
list.add(new Friend("match"));
System.out.println("Before:");
for (Friend f : list) {
System.out.println(f.getFriendCaption());
}
Iterator<Friend> it = list.iterator();
while (it.hasNext()) {
if (it.next().getFriendCaption().equals(targetCaption)) {
it.remove();
// If you know it's unique, you could `break;` here
}
}
System.out.println();
System.out.println("After:");
for (Friend f : list) {
System.out.println(f.getFriendCaption());
}
System.exit(0);
}
private static class Friend {
private String friendCaption;
public Friend(String fc) {
this.friendCaption = fc;
}
public String getFriendCaption() {
return this.friendCaption;
}
}
}
输出:
$ java ListExample
Before:
match
non-match
match
non-match
match
After:
non-match
non-match
这篇关于Java,使用迭代器搜索 ArrayList 并删除匹配的对象的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:Java,使用迭代器搜索 ArrayList 并删除匹配的对象
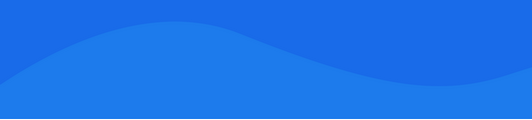
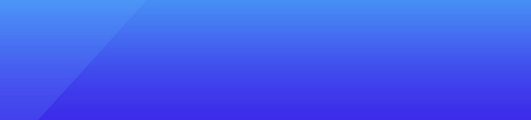
基础教程推荐
- 如何对 HashSet 进行排序? 2022-01-01
- Spring Boot Freemarker从2.2.0升级失败 2022-01-01
- 在螺旋中写一个字符串 2022-01-01
- 如何强制对超级方法进行多态调用? 2022-01-01
- 如何使用 Eclipse 检查调试符号状态? 2022-01-01
- 如何在不安装整个 WTP 包的情况下将 Tomcat 8 添加到 Eclipse Kepler 2022-01-01
- 如何使用 Stream 在集合中拆分奇数和偶数以及两者的总和 2022-01-01
- 由于对所需库 rt.jar 的限制,对类的访问限制? 2022-01-01
- 首次使用 Hadoop,MapReduce Job 不运行 Reduce Phase 2022-01-01
- Java 中保存最后 N 个元素的大小受限队列 2022-01-01