[SpringBoot]: Simple component cannot autowire String class([SpringBoot]:简单组件无法自动连接字符串类)
问题描述
我有这个简单的组件类:
package jason;
import org.springframework.stereotype.Component;
@Component
public class Messenger {
private String message;
public Messenger(String message) {
this.message = message;
}
public void setMessage(String message){
this.message = message;
}
public void getMessage(){
System.out.println("Your Message : " + message);
}
}
在构造函数的参数中,IntelliJ报告:Could not autowire. No beans of 'String' type found.
这个小玩具项目中还有两个类:Config
:
package jason;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
@Configuration
@ComponentScan(basePackageClasses = Messenger.class)
public class Config {
@Bean
public Messenger helloWorld(){
return new Messenger("Hello World!");
}
}
和MainApp
:
package jason;
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class MainApp {
public static void main(String[] args) {
ApplicationContext context = new AnnotationConfigApplicationContext(Config.class);
Messenger obj = context.getBean("helloWorld", Messenger.class);
obj.getMessage();
}
}
奇怪的是,除了表面上的编译时错误之外,项目还会构建,但在运行时会失败,并显示以下信息:
Exception in thread "main" org.springframework.beans.factory.UnsatisfiedDependencyException: Error creating bean with name 'messenger' defined in file [C:Usersjasonfilcodegreen-taxi argetclassesjasonMessenger.class]: Unsatisfied dependency expressed through constructor parameter 0; nested exception is org.springframework.beans.factory.NoSuchBeanDefinitionException: No qualifying bean of type 'java.lang.String' available: expected at least 1 bean which qualifies as autowire candidate. Dependency annotations: {}
我在这里做错了什么?SpringBoot新手。可能存在IOC误解。
推荐答案
您使用Messenger
类混合了两种Bean注入方式,使用@Component
混合了基于注释的注入,并且在Configuration类中使用@Bean
将其声明为Bean。
尝试通过激活的组件扫描使用AnnotationConfigApplicationContext
注入Messenger
时,Spring将首先使用基于注释的注入,因此@Component
。
当然,如果没有基于构造函数的自动装配(这就是您的情况),那么Spring将调用默认构造函数来注入bean,因此您需要将默认构造函数添加到Messenger
。如果没有默认构造函数,Spring将使用可用的构造函数,因此将得到上面的错误。当然,您需要删除@Bean
配置,因为您没有使用它:
打包Jason;
import org.springframework.stereotype.Component;
@Component
public class Messenger {
private String message;
public Messenger() {
}
public Messenger(String message) {
this.message = message;
}
public void setMessage(String message){
this.message = message;
}
public void getMessage(){
System.out.println("Your Message : " + message);
}
}
或者,如果要使用Bean配置,可以从Messenger
中删除@Component
,也可以删除@ComponentScan
:
package jason;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
@Configuration
public class Config {
@Bean
public Messenger helloWorld(){
return new Messenger("Hello World!");
}
}
这篇关于[SpringBoot]:简单组件无法自动连接字符串类的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:[SpringBoot]:简单组件无法自动连接字符串类
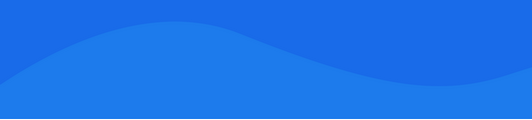
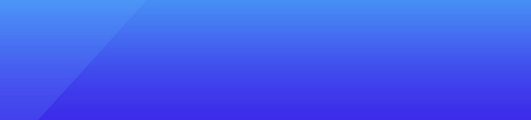
基础教程推荐
- 如何在不安装整个 WTP 包的情况下将 Tomcat 8 添加到 Eclipse Kepler 2022-01-01
- 首次使用 Hadoop,MapReduce Job 不运行 Reduce Phase 2022-01-01
- 在螺旋中写一个字符串 2022-01-01
- 由于对所需库 rt.jar 的限制,对类的访问限制? 2022-01-01
- 如何对 HashSet 进行排序? 2022-01-01
- 如何强制对超级方法进行多态调用? 2022-01-01
- Java 中保存最后 N 个元素的大小受限队列 2022-01-01
- 如何使用 Eclipse 检查调试符号状态? 2022-01-01
- Spring Boot Freemarker从2.2.0升级失败 2022-01-01
- 如何使用 Stream 在集合中拆分奇数和偶数以及两者的总和 2022-01-01