Ignore fields from Java object dynamically while sending as JSON from Spring MVC(在从 Spring MVC 作为 JSON 发送时动态忽略 Java 对象中的字段)
问题描述
I have model class like this, for hibernate
@Entity
@Table(name = "user", catalog = "userdb")
@JsonIgnoreProperties(ignoreUnknown = true)
public class User implements java.io.Serializable {
private Integer userId;
private String userName;
private String emailId;
private String encryptedPwd;
private String createdBy;
private String updatedBy;
@Id
@GeneratedValue(strategy = IDENTITY)
@Column(name = "UserId", unique = true, nullable = false)
public Integer getUserId() {
return this.userId;
}
public void setUserId(Integer userId) {
this.userId = userId;
}
@Column(name = "UserName", length = 100)
public String getUserName() {
return this.userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
@Column(name = "EmailId", nullable = false, length = 45)
public String getEmailId() {
return this.emailId;
}
public void setEmailId(String emailId) {
this.emailId = emailId;
}
@Column(name = "EncryptedPwd", length = 100)
public String getEncryptedPwd() {
return this.encryptedPwd;
}
public void setEncryptedPwd(String encryptedPwd) {
this.encryptedPwd = encryptedPwd;
}
public void setCreatedBy(String createdBy) {
this.createdBy = createdBy;
}
@Column(name = "UpdatedBy", length = 100)
public String getUpdatedBy() {
return this.updatedBy;
}
public void setUpdatedBy(String updatedBy) {
this.updatedBy = updatedBy;
}
}
In Spring MVC controller, using DAO, I am able to get the object. and returning as JSON Object.
@Controller
public class UserController {
@Autowired
private UserService userService;
@RequestMapping(value = "/getUser/{userId}", method = RequestMethod.GET)
@ResponseBody
public User getUser(@PathVariable Integer userId) throws Exception {
User user = userService.get(userId);
user.setCreatedBy(null);
user.setUpdatedBy(null);
return user;
}
}
View part is done using AngularJS, so it will get JSON like this
{
"userId" :2,
"userName" : "john",
"emailId" : "john@gmail.com",
"encryptedPwd" : "Co7Fwd1fXYk=",
"createdBy" : null,
"updatedBy" : null
}
If I don't want to set encrypted Password, I will set that field also as null.
But I don't want like this, I dont want to send all fields to client side. If I dont want password, updatedby, createdby fields to send, My result JSON should be like
{
"userId" :2,
"userName" : "john",
"emailId" : "john@gmail.com"
}
The list of fields which I don't want to send to client coming from other database table. So it will change based on the user who is logged in. How can I do that?
I hope You got my question.
Add the @JsonIgnoreProperties("fieldname")
annotation to your POJO.
Or you can use @JsonIgnore
before the name of the field you want to ignore while deserializing JSON. Example:
@JsonIgnore
@JsonProperty(value = "user_password")
public String getUserPassword() {
return userPassword;
}
GitHub example
这篇关于在从 Spring MVC 作为 JSON 发送时动态忽略 Java 对象中的字段的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:在从 Spring MVC 作为 JSON 发送时动态忽略 Java 对象中的字段
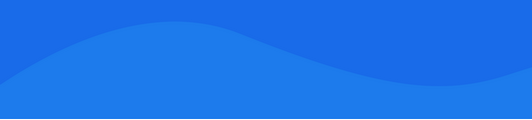
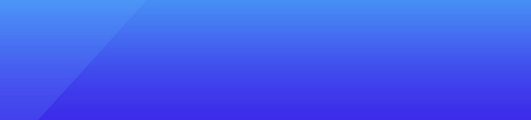
基础教程推荐
- 由于对所需库 rt.jar 的限制,对类的访问限制? 2022-01-01
- Java 中保存最后 N 个元素的大小受限队列 2022-01-01
- 如何对 HashSet 进行排序? 2022-01-01
- 首次使用 Hadoop,MapReduce Job 不运行 Reduce Phase 2022-01-01
- 如何强制对超级方法进行多态调用? 2022-01-01
- 在螺旋中写一个字符串 2022-01-01
- 如何在不安装整个 WTP 包的情况下将 Tomcat 8 添加到 Eclipse Kepler 2022-01-01
- Spring Boot Freemarker从2.2.0升级失败 2022-01-01
- 如何使用 Eclipse 检查调试符号状态? 2022-01-01
- 如何使用 Stream 在集合中拆分奇数和偶数以及两者的总和 2022-01-01