Get java array from c++ via JNI(通过 JNI 从 c++ 获取 java 数组)
问题描述
So, I want to obtain resultNative from c++ but resultNative is in Java. can anybody please show me how to do that via JNI? I am not so familiar with c++ and have searched google for a long time but didn't find any answer. thank you so much. the nativeResult is here in Java.
public String[] searchDatabase()
{
String result[]=new String[6];
String nativeResult[]=new String[2];
tName=this.getTargetName();
result=da.SearchKorea(tName,ctx);
tType=result[2];
tTrans=result[3];
tImage=result[4];
tFave=result[5];
nativeResult[1]= tImage+" - "+tName;
nativeResult[2]= tTrans+" ["+tType+"]";
return nativeResult;
}
First you have to get a reference to the class providing the method. Let's say your class is called MyClass and it is in package p. You get the reference to the class like this:
// You get the JNIEnv* pointer when calling a native function.
jclass myClass = env->FindClass("p/MyClass");
Or if you have a reference to the java object then you can also use GetObjectClass
:
jclass myClass = env->GetObjectClass(javaObject);
Then you have to get the ID of the method you'd like to call by providing the name of the method and a string describing the signature of the method.
"()[java/lang/String;" describes a method expecting no arguments and returning a string array.
jmethodID methodID = env->GetMethodID(myClass , "searchDatabase", "()[java/lang/String;");
Then you have to call the method with JNIEnv::CallObjectMethod
, and here you have to pass the referenc to the java object.
jobjectarray strings = env->CallObjectMethod(javaObject, methodID);
Then you can get an element of the array with GetObjectArrayElement
.
int index = 0;
jstring string = env->GetObjectArrayElement(strings, index);
And then you can get the native string from it in various ways.
const char* nativeChars = env->GetStringUTFChars(string, nullptr);
You can find more information about JNI here, and details about JNI type signatures here.
这篇关于通过 JNI 从 c++ 获取 java 数组的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:通过 JNI 从 c++ 获取 java 数组
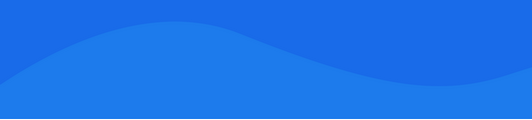
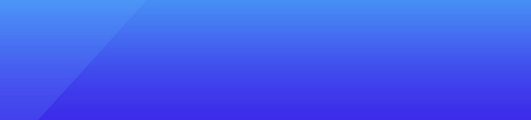
基础教程推荐
- 如何使用 Stream 在集合中拆分奇数和偶数以及两者的总和 2022-01-01
- 由于对所需库 rt.jar 的限制,对类的访问限制? 2022-01-01
- 如何强制对超级方法进行多态调用? 2022-01-01
- Java 中保存最后 N 个元素的大小受限队列 2022-01-01
- 如何使用 Eclipse 检查调试符号状态? 2022-01-01
- 首次使用 Hadoop,MapReduce Job 不运行 Reduce Phase 2022-01-01
- 在螺旋中写一个字符串 2022-01-01
- Spring Boot Freemarker从2.2.0升级失败 2022-01-01
- 如何在不安装整个 WTP 包的情况下将 Tomcat 8 添加到 Eclipse Kepler 2022-01-01
- 如何对 HashSet 进行排序? 2022-01-01