Providing Credentials to Google Cloud Storage API(向 Google Cloud Storage API 提供凭据)
问题描述
我正在尝试编写一个 hello world 程序来测试 Google Cloud Storage.我的目标是拥有最简单的程序,只需将硬编码文件上传到 Cloud Storage.
I'm trying to put together a hello world program for testing out Google Cloud Storage. My goal is to have the simplest possible program that just uploads a hard-coded file to Cloud Storage.
我一直在互联网上搜索基本教程,但我能找到的最接近的是 本指南 用于从 App Engine 使用 Cloud Storage.我整理了这个程序:
I've been scouring the internet for a basic tutorial, but the closest I could find is this guide for using Cloud Storage from App Engine. I've put together this program:
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.util.ArrayList;
import java.util.Arrays;
import com.google.cloud.storage.Acl;
import com.google.cloud.storage.Acl.Role;
import com.google.cloud.storage.Acl.User;
import com.google.cloud.storage.BlobInfo;
import com.google.cloud.storage.Storage;
import com.google.cloud.storage.StorageOptions;
public class Main {
public static void main(String[] args) throws FileNotFoundException{
FileInputStream fileInputStream = new FileInputStream("my-file.txt");
Storage storage = StorageOptions.getDefaultInstance().getService();
BlobInfo blobInfo =
storage.create(
BlobInfo
.newBuilder("my-cloud-storage-bucket", "my-file.txt")
.setAcl(new ArrayList<>(Arrays.asList(Acl.of(User.ofAllUsers(), Role.READER))))
.build(),
fileInputStream);
String fileUrl = blobInfo.getMediaLink();
System.out.println("Uploaded URL: " + fileUrl);
}
}
当我运行该代码时,我在调用 storage.create()
时收到 401 Unauthorized
错误,这并不奇怪,因为我没有提供用户名或密码.我认为这是因为该示例在 App Engine 中运行,它以这种方式提供凭据.
When I run that code, I get a 401 Unauthorized
error when I call storage.create()
, and that's not surprising because I'm not providing a username or password. I gather that's because the example is running in App Engine, which provides the credentials that way.
我尝试通过属性文件传递我的凭据:
I've tried passing in my credentials via a properties file:
Credential credential = new GoogleCredential.Builder()
.setTransport(httpTransport)
.setJsonFactory(jsonFactory)
.setServiceAccountId("accountId")
.setServiceAccountPrivateKeyFromP12File(
new File("PRIVATE_KEY_PATH"))
.setServiceAccountScopes(scopes).build();
Storage storage = new StorageOptions.DefaultStorageFactory().create(StorageOptions.newBuilder().setCredentials(credential).build());
但这不会编译,因为 setCredentials()
函数需要一个 Credentials
实例,而不是 Credentials
实例.
But that doesn't compile because the setCredentials()
function needs a Credentials
instance, not a Credential
instance.
我一直在谷歌搜索和阅读 云Storage API,但我仍然无法弄清楚我应该如何在不经过复杂设置过程的情况下传递凭据.
I've been Googling and reading through the Cloud Storage API, but I still can't figure out how I'm supposed to pass in the credentials without going through a convoluted setup process.
那么,我的问题是:向 Google Cloud 存储提供凭据以上传文件的最简单方法是什么?
So, my question is: what is the simplest way to provide credentials to Google Cloud storage to upload a file?
对于一些背景知识:我正在尝试比较和对比 Google Cloud Storage 和 Amazon S3.我可以在 S3 中创建这样的程序没问题,但由于某种原因,我在使用 Cloud Storage 时遇到了障碍.绝对感谢任何人可以向我提出的任何见解或基本教程.
For some background: I'm trying to compare and contrast Google Cloud Storage and Amazon S3. I can create a program like this in S3 no problem, but for some reason I'm hitting a brick wall with Cloud Storage. Definitely appreciate any insights or basic tutorials anybody can throw my way.
推荐答案
使用新的 Google Cloud 客户端库从 PKCS #12 文件创建凭据似乎不像以前使用旧的 云存储 JSON API.
It seems it's not so easy to create credentials from a PKCS #12 file with new Google Cloud Client Library as it used to be with the old Cloud Storage JSON API.
最简单的方法是使用 JSON 格式,如此处所述,然后使用 GoogleCredentials#fromStream
方法来加载它:
The easiest way would be to use JSON format instead as described here, and then use GoogleCredentials#fromStream
method to load it:
Credentials credentials = GoogleCredentials.fromStream(new FileInputStream("credentials.json"));
Storage storage = StorageOptions.newBuilder().setCredentials(credentials).build().getService();
如果您仍想使用 PKCS #12,我相信这会起作用:
If you still want to use PKCS #12, I believe this would work:
PrivateKey privateKey = SecurityUtils.loadPrivateKeyFromKeyStore(
SecurityUtils.getPkcs12KeyStore(), new FileInputStream("credentials.p12"), "notasecret", "privatekey", "notasecret");
Credentials credentials = new ServiceAccountCredentials(null, "accountId", privateKey, null, null);
Storage storage = StorageOptions.newBuilder().setCredentials(credentials).build().getService();
这篇关于向 Google Cloud Storage API 提供凭据的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:向 Google Cloud Storage API 提供凭据
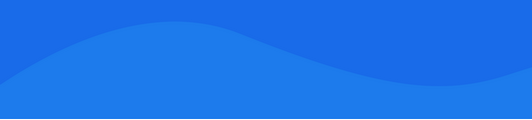
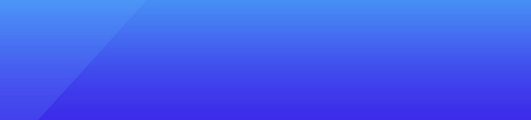
基础教程推荐
- 首次使用 Hadoop,MapReduce Job 不运行 Reduce Phase 2022-01-01
- 如何强制对超级方法进行多态调用? 2022-01-01
- 如何在不安装整个 WTP 包的情况下将 Tomcat 8 添加到 Eclipse Kepler 2022-01-01
- 如何使用 Eclipse 检查调试符号状态? 2022-01-01
- Java 中保存最后 N 个元素的大小受限队列 2022-01-01
- 如何对 HashSet 进行排序? 2022-01-01
- 在螺旋中写一个字符串 2022-01-01
- 由于对所需库 rt.jar 的限制,对类的访问限制? 2022-01-01
- 如何使用 Stream 在集合中拆分奇数和偶数以及两者的总和 2022-01-01
- Spring Boot Freemarker从2.2.0升级失败 2022-01-01