Shared memory between C++ and Java processes(C++ 和 Java 进程之间的共享内存)
问题描述
My aim is to pass data from a C++ process to a Java process and then to receive a result back.
I have achieved this via a named pipe but I would prefer to share the data rather than passing or copying it, assuming the access would be faster.
Initially, I thought of creating a shared segment in C++ that I could write to and read with Java, but I'm not sure if this is possible via JNI, let alone safe.
I believe it's possible in Java to allocate the memory using ByteBuffer.allocateDirect and then use GetDirectBufferAddress to access the address in C++, but if I'm correct this is for native calls within JNI and I can't get this address in my C++ process?
Lost.
Many thanks in advance.
If you have shared memory, for example using CreateFileMapping
(Windows) or shmget
(Unix), all you need is a native method on the Java side. Then you can create a ByteBuffer
that directly accesses the shared memory using NewDirectByteBuffer
like this:
JNIEXPORT jobject JNICALL Java_getSharedBuffer(JNIEnv* env, jobject caller) {
void* myBuffer;
int bufferLength;
Now you have to get a pointer to the shared memory. On Windows you would use something like this:
bufferLength = 1024; // assuming your buffer is 1024 bytes big
HANDLE mem = OpenFileMapping(FILE_MAP_READ, // assuming you only want to read
false, "MyBuffer"); // assuming your file mapping is called "MyBuffer"
myBuffer = MapViewOfFile(mem, FILE_MAP_READ, 0, 0, 0);
// don't forget to do UnmapViewOfFile when you're finished
Now you can just create a ByteBuffer
that is backed by this shared memory:
// put it into a ByteBuffer so the java code can use it
return env->NewDirectByteBuffer(myBuffer, bufferLength);
}
这篇关于C++ 和 Java 进程之间的共享内存的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:C++ 和 Java 进程之间的共享内存
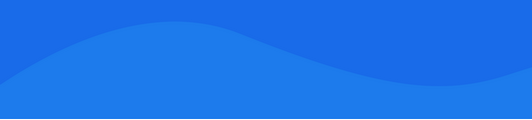
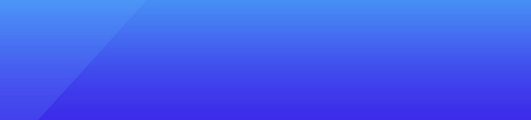
基础教程推荐
- 如何使用 Eclipse 检查调试符号状态? 2022-01-01
- Spring Boot Freemarker从2.2.0升级失败 2022-01-01
- Java 中保存最后 N 个元素的大小受限队列 2022-01-01
- 在螺旋中写一个字符串 2022-01-01
- 由于对所需库 rt.jar 的限制,对类的访问限制? 2022-01-01
- 如何对 HashSet 进行排序? 2022-01-01
- 如何强制对超级方法进行多态调用? 2022-01-01
- 如何在不安装整个 WTP 包的情况下将 Tomcat 8 添加到 Eclipse Kepler 2022-01-01
- 首次使用 Hadoop,MapReduce Job 不运行 Reduce Phase 2022-01-01
- 如何使用 Stream 在集合中拆分奇数和偶数以及两者的总和 2022-01-01