Java collections -- polymorphic access to elements(Java 集合——对元素的多态访问)
问题描述
I have a LinkedHashSet of values of ThisType. ThisType is implementing the interface ThatType.
I need the polymorphic use of this collection-- be able to point to it as LinkedHashSet< ThatType >.
How is this done?
i know this is a naive question, but several things i tried didn't work, and i wanna do it the right way.
Thanks in advance.
//==============================
UPDATE: more details:
In the following code, ThisType implements ThatType-- ThatType is an interface.
//LinkedHashMap<Integer, ? extends ThatType> theMap = new LinkedHashMap<>();
LinkedHashMap<Integer, ThisType> theMap = new LinkedHashMap<>();
for (Integer key:intArray) {
ThisType a = new ThisType();
if (theMap.containsKey(key)) {
a = theMap.get(key);
a.doStuff();
} else {
a.doOtherStuff();
}
theMap.put(key, a);
}
In the end, i wanna return the theMap as a collection of ThatType **, not **ThisType.
this is where the chain is broken. using the commented line (first line) for declaration is giving type mismatch error on put() & get() methods of the hash.
not sure whether this is significant-- but, i'm returning the result as LinkedHashSet< ThatType >. i'm doing the conversion from LinkedHashMap to LinkedHashSet. However, nothing worked nowhere for the polymorphic reference to collection values in the map or set. All and only type i used in all these operations so far is ThisType. ThatType gave me some error just about anywhere i tried it.
I think what you want is to use wildcards.
LinkedHashSet<? extends ThatType> someFunction(LinkedHashSet<? extends ThatType> set) {
return set;
}
As has been explained elsewhere LinkedHashSet<ThisType>
is not a subclass of LinkedHashSet<ThatType>
, since LinkedHashSet<ThisType>
can't accept ThatType
objects.
The wildcard in LinkedHashSet<? extends ThatType>
means a LinkedHastSet
of some class (not sure what) that extends ThatType
.
Though you may want to consider using this:
Set<? extends ThatType> someFunction(Set<? extends ThatType> set) {
return set;
}
这篇关于Java 集合——对元素的多态访问的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:Java 集合——对元素的多态访问
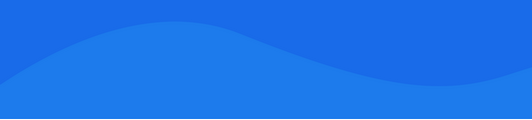
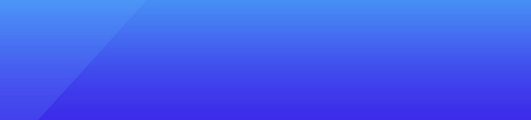
基础教程推荐
- 如何使用 Eclipse 检查调试符号状态? 2022-01-01
- 由于对所需库 rt.jar 的限制,对类的访问限制? 2022-01-01
- 如何使用 Stream 在集合中拆分奇数和偶数以及两者的总和 2022-01-01
- Spring Boot Freemarker从2.2.0升级失败 2022-01-01
- 如何在不安装整个 WTP 包的情况下将 Tomcat 8 添加到 Eclipse Kepler 2022-01-01
- Java 中保存最后 N 个元素的大小受限队列 2022-01-01
- 首次使用 Hadoop,MapReduce Job 不运行 Reduce Phase 2022-01-01
- 如何对 HashSet 进行排序? 2022-01-01
- 在螺旋中写一个字符串 2022-01-01
- 如何强制对超级方法进行多态调用? 2022-01-01