What is polymorphic method in java?(java中的多态方法是什么?)
问题描述
请在 Java 的上下文中解释什么是多态方法".
In the context of Java, please explain what a "polymorphic method" is.
推荐答案
多态"的意思是多种形状".在 Java 中,您可以拥有一个超类,其子类使用相同的名称来做不同的事情.传统的例子是超类 Shape
,子类 Circle
、Square
和 Rectangle
,以及方法 area()
.
"Polymorphic" means "many shapes." In Java, you can have a superclass with subclasses that do different things, using the same name. The traditional example is superclass Shape
, with subclasses Circle
, Square
, and Rectangle
, and method area()
.
所以,例如
// note code is abbreviated, this is just for explanation
class Shape {
public int area(); // no implementation, this is abstract
}
class Circle {
private int radius;
public Circle(int r){ radius = r ; }
public int area(){ return Math.PI*radius*radius ; }
}
class Square {
private int wid;
Public Square(int w){ wid=w; }
public int area() { return wid*wid; }
}
现在考虑一个例子
Shape s[] = new Shape[2];
s[0] = new Circle(10);
s[1] = new Square(10);
System.out.println("Area of s[0] "+s[0].area());
System.out.println("Area of s[1] "+s[1].area());
s[0].area()
调用Circle.area()
,s[1].area()
调用Square.area()
-- 因此我们说 Shape
及其子类利用对方法区域的多态调用.
s[0].area()
calls Circle.area()
, s[1].area()
calls Square.area()
-- and thus we say that Shape
and its subclasses exploit polymorphic calls to the method area.
这篇关于java中的多态方法是什么?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:java中的多态方法是什么?
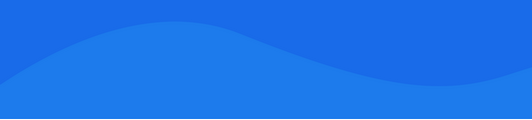
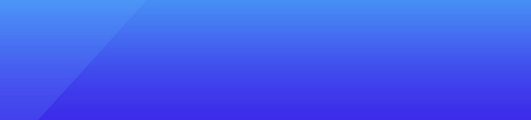
基础教程推荐
- 如何强制对超级方法进行多态调用? 2022-01-01
- 如何使用 Stream 在集合中拆分奇数和偶数以及两者的总和 2022-01-01
- 如何使用 Eclipse 检查调试符号状态? 2022-01-01
- 首次使用 Hadoop,MapReduce Job 不运行 Reduce Phase 2022-01-01
- Spring Boot Freemarker从2.2.0升级失败 2022-01-01
- 如何对 HashSet 进行排序? 2022-01-01
- 在螺旋中写一个字符串 2022-01-01
- 由于对所需库 rt.jar 的限制,对类的访问限制? 2022-01-01
- Java 中保存最后 N 个元素的大小受限队列 2022-01-01
- 如何在不安装整个 WTP 包的情况下将 Tomcat 8 添加到 Eclipse Kepler 2022-01-01