how to make a frame display 50 times for 1 second and disappear each time it#39;s displayed(如何让一个框架在1秒内显示50次并在每次显示时消失)
问题描述
I need my JFrame to display itself within the dimensions of my screen and it should display the frame with the message then disappear for 1 second then reappear 50 times here's my code so far:
import javax.swing.*;
import java.awt.*;
import java.util.*;
public class OurMain {
public static void main(String[] args) {
Dimension sSize = Toolkit.getDefaultToolkit().getScreenSize();
int w = sSize.width;
int h = sSize.height;
Random rand = new Random();
int z = rand.nextInt(sSize.width);
int c = rand.nextInt(sSize.height);
int x = (int)((Math.random()* w) - z);
int y = (int)((Math.random()* h) - 100);
for(int g = 0; g < 51; g++){
JFrame f = new MyFrame(z, 100, x, y);
f.pack();
f.setVisible(true);
f.setVisible(false);
}
}
}
and here's my JFrame:
import java.awt.*;
import javax.swing.*;
import java.util.*;
public class MyFrame extends JFrame{
MyFrame(int width, int height, int x, int y){
super();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setTitle("R and T's Main Frame");
setSize(width, height);
setLocation(x, y);
int v;
v = 5 + (int)(Math.random() * (1 - 5) + 1);
JLabel label = new JLabel("Software Engineering");
label.setFont(new Font("Impact", Font.BOLD, height/v));
label.setForeground(Color.BLUE);
getContentPane().add(label);
setVisible(true);
}
}
So yeah I have two problems 1. the frame seems to go outside of the screen sometimes when it should be displayed within the dimensions of my screen resolution and 2. The second is the iteration of the loop 50 times and for 1 second it should be displayed and then disappear for 1 second and continue on like that for 50 frames appearing.
First, I feel I have to tell you:
You just wrote 5 lines since your previous post.
Here we expect people to put more effort, try things and then ask, explining where they are stuck. We are not here to do your homework, we are here to help, so first, try it out by yourself. It doesn't look like you did any kind of research to me. If you want to get help, this must change because people notice that and don't like it.
Even if you did not get results, post what you tried, what were the results, what you expected them to be etc.
Code
This could be done in several ways, since you did not seem to care about it, neither added any preffered way to do it, I did it on my own.
Some possibilities are:
Create one JFrame and move it's location whenever you want to set it visible
Create a JFrame on every iteration of the loop (likely to be slower than the previous one?)
Many more...
I liked the first approach more, so I did that one. This is what it does:
Appear and dissappear 50 times
When it goes visible and invisible waits 1 second
Everytime it's set as visible the location and size of the frame changes
It will always be inside the screen
OurMain.java
public class OurMain {
public static void main(String[] args) {
Dimension screenSize = Toolkit.getDefaultToolkit().getScreenSize();
int screenWidth = screenSize.width;
int screenHeight = screenSize.height;
Random rand = new Random();
MyFrame frame = new MyFrame();
for (int g = 0; g < 50; g++) {
int frameWidth = rand.nextInt(screenSize.width);
int frameHeight = rand.nextInt(screenSize.height);
int frameLocationX = rand.nextInt(screenWidth - frameWidth);
int frameLocationY = rand.nextInt(screenHeight - frameHeight);
frame.setSize(frameWidth, frameHeight);
frame.setLocation(frameLocationX, frameLocationY);
frame.setVisible(true);
try {
Thread.sleep(1000); // Waits 1000 miliseconds = 1 second
} catch (InterruptedException e) { }
frame.setVisible(false);
try {
Thread.sleep(1000); // Waits 1000 miliseconds = 1 second
} catch (InterruptedException e) { }
}
}
}
MyFrame.java
public class MyFrame extends JFrame {
private static final long serialVersionUID = 1L;
private JLabel label;
public MyFrame() {
super();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setTitle("R and T's Main Frame");
label = new JLabel("Software Engineering");
label.setForeground(Color.BLUE);
getContentPane().add(label);
}
}
I did not comment the code, it's pretty simple. Anyway, if you need some explanation feel free to ask in the comments.
EDIT: Answers to questions on comments
If I didn't want to change the height of it and keep it 100, frameLocationY = 100 ?
No, that would change its location, you need to change frameHeight
to 100
interruptedException is empty cause nothing would go wrong
In this case, that's true, however, a Thread
can be stopped (see interrupt()) and therefore it would execute what it's inside the catch
brakets
an object called thread.sleep
public static void Thread.sleep(long milis) throws InterruptedException
is a function
inside the Thread
class. Since it's static, it doesn't need an instance of Thread
to be created
the f.pack(); so that within the frame the text is proportionate to the random frame size
If I am not mistaken, what pack()
does is give all the space they need to each component of the frame, however, since the text is so little and the frame so big, giving the label it's preffered size would eventually wrap it, resizing the size we set before
Everytime it's set as visible the location and size of the frame changes'' as does the content in it is proportionate to its size
That's more tricky, nevertheless, since you only have a JLabel, we can calculate the value of the font it needs to take all the space of the frame. Look this link and try to add that to the code, tell us if you can't
这篇关于如何让一个框架在1秒内显示50次并在每次显示时消失的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何让一个框架在1秒内显示50次并在每次显示时消失
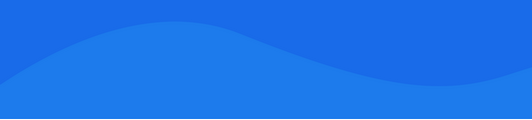
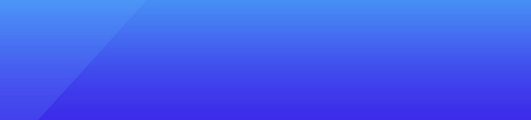
基础教程推荐
- Java 中保存最后 N 个元素的大小受限队列 2022-01-01
- 如何使用 Stream 在集合中拆分奇数和偶数以及两者的总和 2022-01-01
- 在螺旋中写一个字符串 2022-01-01
- 由于对所需库 rt.jar 的限制,对类的访问限制? 2022-01-01
- Spring Boot Freemarker从2.2.0升级失败 2022-01-01
- 首次使用 Hadoop,MapReduce Job 不运行 Reduce Phase 2022-01-01
- 如何强制对超级方法进行多态调用? 2022-01-01
- 如何在不安装整个 WTP 包的情况下将 Tomcat 8 添加到 Eclipse Kepler 2022-01-01
- 如何对 HashSet 进行排序? 2022-01-01
- 如何使用 Eclipse 检查调试符号状态? 2022-01-01