JComponents not showing up with picture background?(JComponents没有显示图片背景?)
问题描述
我的组件没有显示出来.我该如何解决这个问题?
My components are not showing up. How do I fix this?
代码:
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
import javax.swing.*;
public class login implements ActionListener{
JTextField gusername;
JTextField gpassword;
static String username;
static String password;
void logini() throws IOException {
JFrame window = new JFrame("Login");
window.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
window.setSize(300, 250);
window.setResizable(false);
window.setVisible(true);
JPanel mainp = new JPanel(new GridBagLayout());
GridBagConstraints c = new GridBagConstraints();
window.add(mainp);
BufferedImage myPicture = ImageIO.read(new File("c:\bgd.png"));
JLabel picLabel = new JLabel(new ImageIcon( myPicture ));
mainp.add(picLabel, c);
c.gridx = 0;
c.gridy = 1;
gusername = new JTextField();
gusername.setText("Username");
mainp.add(gusername, c);
c.gridx = 0;
c.gridy = 2;
gpassword = new JTextField();
gpassword.setText(" password ");
mainp.add(gpassword, c);
c.gridx = 0;
c.gridy = 3;
JButton login = new JButton("Login");
mainp.add(login, c);
login.addActionListener(this);
login.setActionCommand("ok");
}
public void actionPerformed(ActionEvent e) {
if (e.getActionCommand().equalsIgnoreCase("ok")){
try {
this.username = (gusername.getText());
this.password = (gpassword.getText());
System.out.println("0");
}
catch(NumberFormatException ex){
System.out.println("ERROR: Could not preform function: 7424");
}
}
}
}
结果:
推荐答案
先看这个小例子,如果你明白这里发生了什么,请告诉我.那么只有我们会更进一步,慢慢地慢慢地.尝试通过这个示例,我将向您展示如何在 JPanel
Here first watch this small example, do let me know if you understood what is going on in here. Then only we will go a step further, slowly slowly. Try to go through this example, in which I am showing you how to draw on a JPanel
import java.awt.*;
import java.awt.image.BufferedImage;
import java.io.IOException;
import java.net.URL;
import javax.imageio.ImageIO;
import javax.swing.*;
public class PaintingExample {
private CustomPanel contentPane;
private void displayGUI() {
JFrame frame = new JFrame("Painting Example");
frame.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
contentPane = new CustomPanel();
frame.setContentPane(contentPane);
frame.pack();
frame.setLocationByPlatform(true);
frame.setVisible(true);
}
public static void main(String... args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
new PaintingExample().displayGUI();
}
});
}
}
class CustomPanel extends JPanel {
private BufferedImage image;
public CustomPanel() {
setOpaque(true);
setBorder(BorderFactory.createLineBorder(Color.BLACK, 5));
try {
/*
* Since Images are Application Resources,
* it's always best to access them in the
* form of a URL, instead of File, as you are doing.
* Uncomment this below line and watch this answer
* of mine, as to HOW TO ADD IMAGES TO THE PROJECT
* http://stackoverflow.com/a/9866659/1057230
* In order to access images with getClass().getResource(path)
* here your Directory structure has to be like this
* Project
* |
* ------------------------
* | |
* bin src
* | |
* --------- .java files
* | |
* package image(folder)
* ( or |
* .class 404error.jpg
* files, if
* no package
* exists.)
*/
//image = ImageIO.read(
// getClass().getResource(
// "/image/404error.jpg"));
image = ImageIO.read(new URL(
"http://i.imgur.com/8zgHpH8.jpg"));
} catch(IOException ioe) {
System.out.println("Unable to fetch image.");
ioe.printStackTrace();
}
}
/*
* Make this one customary habbit,
* of overriding this method, when
* you extends a JPanel/JComponent,
* to define it's Preferred Size.
* Now in this case we want it to be
* as big as the Image itself.
*/
@Override
public Dimension getPreferredSize() {
return (new Dimension(image.getWidth(), image.getHeight()));
}
/*
* This is where the actual Painting
* Code for the JPanel/JComponent
* goes. Here we will draw the image.
* Here the first line super.paintComponent(...),
* means we want the JPanel to be drawn the usual
* Java way first (this usually depends on the opaque
* property of the said JComponent, if it's true, then
* it becomes the responsibility on the part of the
* programmer to fill the content area with a fully
* opaque color. If it is false, then the programmer
* is free to leave it untouched. So in order to
* overcome the hassle assoicated with this contract,
* super.paintComponent(g) is used, since it adheres
* to the rules, and performs the same task, depending
* upon whether the opaque property is true or false),
* then later on we will add our image to it, by
* writing the other line, g.drawImage(...).
*/
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
g.drawImage(image, 0, 0, this);
}
}
编译运行:
- 启动命令提示符/终端/cmd.并移动到指定的位置到 Project 文件夹
现在使用以下代码编译代码:
- Start command prompt/terminal/cmd. And move to the location referred to by the Project folder
Now compile the code by using:
C:Project>javac -d bin src*.java
C:Project>javac -d bin src*.java
现在移动到 bin 文件夹,通过发出命令:
Now move to bin folder, by issuing command:
C:Project>cd bin
C:Project>cd bin
进入 bin 文件夹后,发出以下命令运行:
Once inside bin folder, issue the following command to run:
C:Projectin>java PaintingExample
C:Projectin>java PaintingExample
这是使用 JLabel
作为图像基础时的代码:
Here is the code when using JLabel
as the base for the image :
import java.awt.*;
import java.net.MalformedURLException;
import java.net.URL;
import javax.swing.*;
public class LabelExample {
private JPanel contentPane;
private JLabel imageLabel;
private void displayGUI() {
JFrame frame = new JFrame("Label Example");
frame.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
contentPane = new JPanel();
contentPane.setOpaque(true);
contentPane.setBorder(
BorderFactory.createLineBorder(Color.BLACK, 5));
//imageLabel = new JLabel(
// new ImageIcon(
// getClass().getResource(
// "/image/404error.jpg")));
try {
imageLabel = new JLabel(new ImageIcon(
new URL("http://i.imgur.com/8zgHpH8.jpg")));
} catch(MalformedURLException mue) {
System.out.println(
"Unable to get Image from"
+ "the Resource specified.");
mue.printStackTrace();
}
contentPane.add(imageLabel);
frame.setContentPane(contentPane);
frame.pack();
frame.setLocationByPlatform(true);
frame.setVisible(true);
}
public static void main(String... args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
new LabelExample().displayGUI();
}
});
}
}
这是上面两个代码的输出:
Here is the output of both the above codes :
这篇关于JComponents没有显示图片背景?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:JComponents没有显示图片背景?
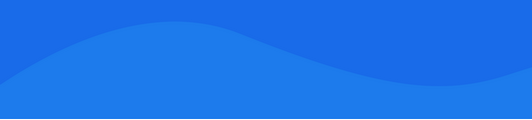
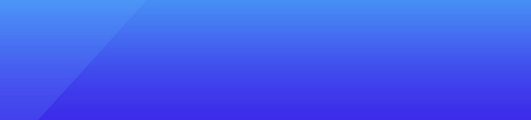
基础教程推荐
- 如何使用 Eclipse 检查调试符号状态? 2022-01-01
- 首次使用 Hadoop,MapReduce Job 不运行 Reduce Phase 2022-01-01
- 如何强制对超级方法进行多态调用? 2022-01-01
- 如何对 HashSet 进行排序? 2022-01-01
- 在螺旋中写一个字符串 2022-01-01
- 如何在不安装整个 WTP 包的情况下将 Tomcat 8 添加到 Eclipse Kepler 2022-01-01
- 如何使用 Stream 在集合中拆分奇数和偶数以及两者的总和 2022-01-01
- Java 中保存最后 N 个元素的大小受限队列 2022-01-01
- 由于对所需库 rt.jar 的限制,对类的访问限制? 2022-01-01
- Spring Boot Freemarker从2.2.0升级失败 2022-01-01