Find all the combination of substrings that add up to the given string(查找加起来为给定字符串的所有子字符串组合)
问题描述
我正在尝试创建一个数据结构,它包含所有可能的子字符串组合,这些组合加起来是原始字符串.例如,如果字符串是 "java"
,则有效结果将是 "j", "ava"
, "ja", "v", "a"
,无效结果将是 "ja", "a"
或 "a", "jav"
I'm trying to create a data structure that holds all the possible substring combinations that add up to the original string. For example, if the string is "java"
the valid results would be "j", "ava"
, "ja", "v", "a"
, an invalid result would be "ja", "a"
or "a", "jav"
我很容易找到所有可能的子字符串
I had it very easy in finding all the possible substrings
String string = "java";
List<String> substrings = new ArrayList<>();
for( int c = 0 ; c < string.length() ; c++ )
{
for( int i = 1 ; i <= string.length() - c ; i++ )
{
String sub = string.substring(c, c+i);
substrings.add(sub);
}
}
System.out.println(substrings);
现在我正在尝试构建一个仅包含有效子字符串的结构.但它几乎没有那么容易.我陷入了一个非常丑陋的代码的迷雾中,摆弄着索引,而且还没有完成,很可能完全走错了路.有什么提示吗?
and now I'm trying to construct a structure that holds only the valid substrings. But its not nearly as easy. I'm in the mist of a very ugly code, fiddling around with the indexes, and no where near of finishing, most likely on a wrong path completely. Any hints?
推荐答案
这是一种方法:
static List<List<String>> substrings(String input) {
// Base case: There's only one way to split up a single character
// string, and that is ["x"] where x is the character.
if (input.length() == 1)
return Collections.singletonList(Collections.singletonList(input));
// To hold the result
List<List<String>> result = new ArrayList<>();
// Recurse (since you tagged the question with recursion ;)
for (List<String> subresult : substrings(input.substring(1))) {
// Case: Don't split
List<String> l2 = new ArrayList<>(subresult);
l2.set(0, input.charAt(0) + l2.get(0));
result.add(l2);
// Case: Split
List<String> l = new ArrayList<>(subresult);
l.add(0, input.substring(0, 1));
result.add(l);
}
return result;
}
输出:
[java]
[j, ava]
[ja, va]
[j, a, va]
[jav, a]
[j, av, a]
[ja, v, a]
[j, a, v, a]
这篇关于查找加起来为给定字符串的所有子字符串组合的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:查找加起来为给定字符串的所有子字符串组合
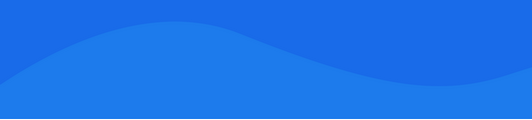
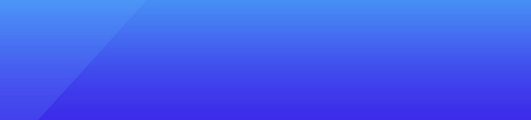
基础教程推荐
- 如何对 HashSet 进行排序? 2022-01-01
- 如何使用 Eclipse 检查调试符号状态? 2022-01-01
- Java 中保存最后 N 个元素的大小受限队列 2022-01-01
- 如何使用 Stream 在集合中拆分奇数和偶数以及两者的总和 2022-01-01
- 如何在不安装整个 WTP 包的情况下将 Tomcat 8 添加到 Eclipse Kepler 2022-01-01
- 在螺旋中写一个字符串 2022-01-01
- 由于对所需库 rt.jar 的限制,对类的访问限制? 2022-01-01
- 首次使用 Hadoop,MapReduce Job 不运行 Reduce Phase 2022-01-01
- Spring Boot Freemarker从2.2.0升级失败 2022-01-01
- 如何强制对超级方法进行多态调用? 2022-01-01