Sending Objects Across Network using UDP in Java(在 Java 中使用 UDP 跨网络发送对象)
问题描述
I haven't found an exact answer to the problem that I'm having, so I'm going to ask this anyway, and if I did, in fact, repost a question that's already been asked, I apologize.
I'm doing another lab assignment for my Java class, and for this week's lab, I have to make a UDP server to send out a Message object across the network, then I have to make a UDP client to read in that Message. The Message itself is just an Object that has a String message and a String username; fairly arbitrary. Message is Serializable.
Now, what I'm having an issue with is how to actually go about sending that Message Object across the network within a DatagramPacket? The actual constructor has me put in a byte array, the size of the array, the InetAddress, and the port number. My final question is: how to I find out the size of the array, and how to I turn my Message into bytes to be sent out?
You need to serialize your message class into a byte array. This will be the byte array you send (and it will be easy to get the size at that point).
On the client, you will want to deserialize the byte array back into a Message object.
Java provides a set of classes to handle serialization/deserialization, and the object you want to serialize must implement the "Serializable" interface.
Something like this would work:
// Serialize to a byte array
ByteArrayOutputStream bStream = new ByteArrayOutputStream();
ObjectOutput oo = new ObjectOutputStream(bStream);
oo.writeObject(messageClass);
oo.close();
byte[] serializedMessage = bStream.toByteArray();
And on the receiving end:
ObjectInputStream iStream = new ObjectInputStream(new ByteArrayInputStream(recBytes));
Message messageClass = (Message) iStream.readObject();
iStream.close();
Note, you should create a common interface that is shared between the client and the server, this will allow for easy serialization/deserialization of the payload.
这篇关于在 Java 中使用 UDP 跨网络发送对象的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:在 Java 中使用 UDP 跨网络发送对象
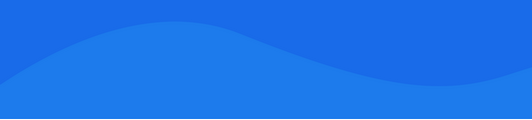
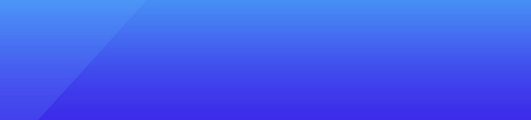
基础教程推荐
- 如何使用 Eclipse 检查调试符号状态? 2022-01-01
- 在螺旋中写一个字符串 2022-01-01
- Java 中保存最后 N 个元素的大小受限队列 2022-01-01
- 首次使用 Hadoop,MapReduce Job 不运行 Reduce Phase 2022-01-01
- 如何在不安装整个 WTP 包的情况下将 Tomcat 8 添加到 Eclipse Kepler 2022-01-01
- 由于对所需库 rt.jar 的限制,对类的访问限制? 2022-01-01
- Spring Boot Freemarker从2.2.0升级失败 2022-01-01
- 如何强制对超级方法进行多态调用? 2022-01-01
- 如何使用 Stream 在集合中拆分奇数和偶数以及两者的总和 2022-01-01
- 如何对 HashSet 进行排序? 2022-01-01