Correctly configure spring security oauth2(正确配置Spring安全OAuth2)
问题描述
我正在尝试使用Spring-Security-OAuth2和JWT配置授权服务器。
我的Main:
@SpringBootApplication
@EnableResourceServer
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
我的安全配置:
@Configuration
@EnableGlobalMethodSecurity(prePostEnabled = true)
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
@Bean
public AuthenticationManager authenticationManagerBean() throws Exception {
return super.authenticationManagerBean();
}
@Override
protected void configure(HttpSecurity http) throws Exception {
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth
.inMemoryAuthentication()
.withUser("john").password("123").roles("USER").authorities("FOO_READ");
}
}
我的身份验证服务器配置:
@Configuration
@EnableAuthorizationServer
public class AuthServerConfig extends AuthorizationServerConfigurerAdapter {
@Autowired
@Qualifier("authenticationManagerBean")
private AuthenticationManager authenticationManager;
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients.inMemory()
.withClient("gateway")
.secret("secret")
.authorizedGrantTypes("refresh_token", "password")
.scopes("GATEWAY")
.autoApprove(true) ;
}
@Override
public void configure(AuthorizationServerEndpointsConfigurer endpoints) throws Exception {
endpoints.tokenEnhancer(jwtTokenEnhancer()).authenticationManager(authenticationManager);
}
}
和用于访问当前用户的Web服务:
@RestController
public class UserController {
@GetMapping("/users/me")
public Principal user(Principal principal) {
return principal;
}
}
现在,如果我这样做POST请求:
gateway:secret@localhost:10101/oauth/token
grant_type : password
username : john
password : 123
我得到的回复如下:
{
"access_token": "...access token...",
"token_type": "bearer",
"refresh_token": "...refresh token...",
"expires_in": 43199,
"scope": "GATEWAY",
"jti": "...jti..."
}
如果我使用访问令牌呼叫我的用户WS:
GET localhost:10101/users/me
Authorization : Bearer ...access token...
我获得空响应。
但如果我的安全配置中有这几行:
@Override
protected void configure(HttpSecurity http) throws Exception {
http.requestMatchers().antMatchers("/wtf");
}
然后我获得相应的响应。
有人能给我解释一下这是怎么工作的吗?如果没有这一附加行,为什么无法识别我的用户?
谢谢。
推荐答案
实际上是Spring安全问题。 解决方案在此帖子中:
https://github.com/spring-projects/spring-security-oauth/issues/980
应添加此批注才能正确调用适配器:
@Configuration
@Order(SecurityProperties.ACCESS_OVERRIDE_ORDER)
class WebSecurityConfiguration extends WebSecurityConfigurerAdapter {
// ...
}
另一个解决方案是实现ResourceServerConfigurerAdapter而不是WebSecurityConfigurerAdapter。
这篇关于正确配置Spring安全OAuth2的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:正确配置Spring安全OAuth2
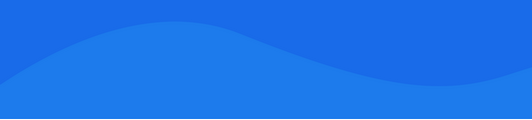
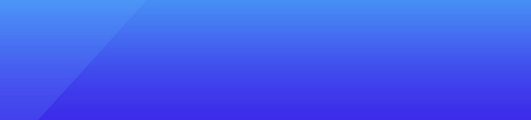
基础教程推荐
- 如何在不安装整个 WTP 包的情况下将 Tomcat 8 添加到 Eclipse Kepler 2022-01-01
- 如何强制对超级方法进行多态调用? 2022-01-01
- Java 中保存最后 N 个元素的大小受限队列 2022-01-01
- 由于对所需库 rt.jar 的限制,对类的访问限制? 2022-01-01
- 如何使用 Stream 在集合中拆分奇数和偶数以及两者的总和 2022-01-01
- Spring Boot Freemarker从2.2.0升级失败 2022-01-01
- 在螺旋中写一个字符串 2022-01-01
- 如何对 HashSet 进行排序? 2022-01-01
- 如何使用 Eclipse 检查调试符号状态? 2022-01-01
- 首次使用 Hadoop,MapReduce Job 不运行 Reduce Phase 2022-01-01