Testcase for creating zip file in java junit(在Java junit中创建压缩文件的测试用例)
问题描述
我被编写了一个方法,该方法接受一个文件,然后在它创建一个压缩文件之后。一旦压缩文件创建完成,它就会尝试将其存储在GCS存储桶中,并从临时目录中删除这些文件。 有人能帮我写一个测试用例吗?
private void createZip(File file) throws IOException {
File zipFile = new File(System.getProperty("java.io.tmpdir"), Constants.ZIP_FILE_NAME);
if (!file.exists()) {
logger.info("File Not Found For To Do Zip File! Please Provide A File..");
throw new FileNotFoundException("File Not Found For To Do Zip File! Please Provide A File..");
}
try (ZipOutputStream zos = new ZipOutputStream(new FileOutputStream(zipFile));
FileInputStream fis = new FileInputStream(file);) {
ZipEntry zipEntry = new ZipEntry(file.getName());
zos.putNextEntry(zipEntry);
byte[] buffer = new byte[1024];
int len;
while ((len = fis.read(buffer)) > 0) {
zos.write(buffer, 0, len);
}
zos.closeEntry();
}
try {
// store to GCS bucket
GcpCloudStorageUtil.uploadFileToGcsBucket(zipFile, zipFile.getName());
file.delete();
zipFile.delete();
} catch (Exception e) {
logger.error("Exception" + e);
throw new FxRuntimeException("Exception " + e.getMessage());
}
}
推荐答案
作为设计测试用例的一般经验法则,您希望同时考虑一切正常时的预期行为和可能出错的每种情况的预期行为。
对于您的特定问题,当一切正常时,您应该希望您的方法压缩作为参数给定的文件,上载它并从临时目录中删除该文件。 您可以通过监视静态GcpCloudStorageUtil方法来做到这一点。间谍是类的实例,除非指定以及您可以监视哪些方法调用,否则将保留其通常的行为。您可以阅读主题here和here(用于静态方法间谍)的更多信息。
在没有访问其余代码的情况下,很难给出完整的答案,但我建议:
- 创建非空文件
- 对此文件调用createZip方法
- 验证是否调用了GcpCloudStorageUtil.ploadFileToGcsBucket方法
- 验证上载的文件内容是否与您创建的文件匹配
- 正在验证临时目录现在是否为空
也有很多方面可能会出错。例如,作为参数提供的文件可能不存在。您想要编写一个测试用例,以确保在这种情况下抛出FileNotFoundException。本article介绍了使用JUnit4或JUnit5执行此操作的不同方法。
类似地,您可以设计一个测试用例,在其中模拟GcpCloudStorageUtil.ploadFileToGcsBucket并强制它抛出异常,然后验证是否抛出了预期的异常。模仿方法意味着强制它以某种方式运行(在这里,抛出异常)。同样,您可以阅读主题here的更多内容。编辑:这是一个可能的测试类。
@SpringBootTest
public class ZipperTest {
MockedStatic<GcpCloudStorageUtil> mockGcpCloudStorageUtil;
@Before
public void init(){
mockGcpCloudStorageUtil = Mockito.mockStatic(GcpCloudStorageUtil.class);
}
@After
public void clean(){
mockGcpCloudStorageUtil.close();
}
@Test
public void testFileIsUploadedAndDeleted() throws IOException, FxRuntimeException {
//mocks the GcpCloudStorageUtil class and ensures it behaves like it normally would
mockGcpCloudStorageUtil.when(() -> GcpCloudStorageUtil.uploadFileToGcsBucket(Mockito.any(File.class), Mockito.anyString())).thenCallRealMethod();
Zipper zipper = new Zipper();
//creates a file
String content = "My file content";
Files.write(Paths.get("example.txt"),
List.of(content),
StandardCharsets.UTF_8,
StandardOpenOption.CREATE,
StandardOpenOption.APPEND);
zipper.createZip(new File("example.txt"));
//verifies that the uploadFileToGcsBucket method was called once
mockGcpCloudStorageUtil.verify(()->GcpCloudStorageUtil.uploadFileToGcsBucket(Mockito.any(File.class), Mockito.anyString()), times(1));
//you can insert code here to verify the content of the uploaded file matches the provided file content
//verifies that the file in the temp directory has been deleted
Assert.assertFalse(Files.exists(Paths.get(System.getProperty("java.io.tmpdir")+"example.txt")));
}
@Test(expected = FileNotFoundException.class)
public void testExceptionThrownWhenFileDoesNotExist() throws FxRuntimeException, IOException {
mockGcpCloudStorageUtil.when(() -> GcpCloudStorageUtil.uploadFileToGcsBucket(Mockito.any(File.class), Mockito.anyString())).thenCallRealMethod();
Zipper zipper = new Zipper();
zipper.createZip(new File("doesnt_exist.txt"));
//verifies that the uploadFileToGcsBucket method was not called
mockGcpCloudStorageUtil.verifyNoInteractions();
}
@Test(expected = FxRuntimeException.class)
public void testExceptionThrownWhenIssueGcpUpload() throws IOException, FxRuntimeException {
//this time we force the uploadFileToGcsBucket method to throw an exception
mockGcpCloudStorageUtil.when(() -> GcpCloudStorageUtil.uploadFileToGcsBucket(Mockito.any(File.class), Mockito.anyString())).thenThrow(RuntimeException.class);
Zipper zipper = new Zipper();
//creates a file
String content = "My file content";
Files.write(Paths.get("example.txt"),
List.of(content),
StandardCharsets.UTF_8,
StandardOpenOption.CREATE,
StandardOpenOption.APPEND);
zipper.createZip(new File("example.txt"));
mockGcpCloudStorageUtil.verify(()->GcpCloudStorageUtil.uploadFileToGcsBucket(Mockito.any(File.class), Mockito.anyString()), times(1));
}
}
这篇关于在Java junit中创建压缩文件的测试用例的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:在Java junit中创建压缩文件的测试用例
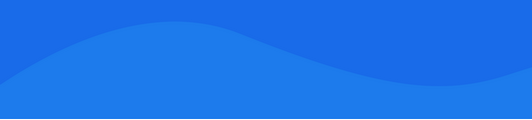
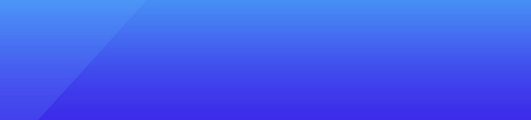
基础教程推荐
- Java 中保存最后 N 个元素的大小受限队列 2022-01-01
- 如何对 HashSet 进行排序? 2022-01-01
- 如何在不安装整个 WTP 包的情况下将 Tomcat 8 添加到 Eclipse Kepler 2022-01-01
- 首次使用 Hadoop,MapReduce Job 不运行 Reduce Phase 2022-01-01
- 在螺旋中写一个字符串 2022-01-01
- 由于对所需库 rt.jar 的限制,对类的访问限制? 2022-01-01
- 如何使用 Stream 在集合中拆分奇数和偶数以及两者的总和 2022-01-01
- 如何使用 Eclipse 检查调试符号状态? 2022-01-01
- Spring Boot Freemarker从2.2.0升级失败 2022-01-01
- 如何强制对超级方法进行多态调用? 2022-01-01