How to place the dataTables#39; horizontal scrollbar on top of the table?(如何将 dataTables 的水平滚动条放在表格顶部?)
问题描述
I have a really large datatable and I want to put the horizontal scrollbar on top as well as on the bottom of the table so it would be easier for the user to scroll (the datatable has a lot of columns). Is there an easy and proper way to achieve this?
You can use a container that has a maximum width and put the table inside.
<div class="large-table-container-1">
<table>...</table>
</div>
Depending on how much reliability you want, you can make:
A. Bottom scrollbar, just set a maximum width and overflow x to scroll:
.large-table-container-1 {
max-width: 200px;
overflow-x: scroll;
overflow-y: auto;
}
Demo:
.large-table-container-1 {
max-width: 200px;
overflow-x: scroll;
overflow-y: auto;
}
.large-table-container-1 table {
}
/*misc*/
td {
border: 1px solid gray;
}
th {
text-align: left;
}
<div class="large-table-container-1">
<table>
<thead>
<tr>
<th colspan="20">
Bottom scrollbar:
</th>
</tr>
</thead>
<tbody>
<tr>
<td>00</td>
<td>01</td>
<td>02</td>
<td>03</td>
<td>04</td>
<td>05</td>
<td>06</td>
<td>07</td>
<td>08</td>
<td>09</td>
<td>00</td>
<td>01</td>
<td>02</td>
<td>03</td>
<td>04</td>
<td>05</td>
<td>06</td>
<td>07</td>
<td>08</td>
<td>09</td>
</tr>
</tbody>
</table>
</div>
B. Top scrollbar, a little hacky, use a 180 degree transform to rotate the scrollbar to the top, then again another 180 degree to put back the content.
.large-table-container-2 {
max-width: 200px;
overflow-x: scroll;
overflow-y: auto;
transform:rotateX(180deg);
}
.large-table-container-2 table {
transform:rotateX(180deg);
}
You may want to use broswser specific prefixes for transform, like -webkit-transform:rotateX(180deg);
.
Demo:
.large-table-container-2 {
max-width: 200px;
overflow-x: scroll;
overflow-y: auto;
transform:rotateX(180deg);
}
.large-table-container-2 table {
transform:rotateX(180deg);
}
/*misc*/
td {
border: 1px solid gray;
}
th {
text-align: left;
}
<div class="large-table-container-2">
<table>
<thead>
<tr>
<th colspan="20">
Top scrollbar:
</th>
</tr>
</thead>
<tbody>
<tr>
<td>00</td>
<td>01</td>
<td>02</td>
<td>03</td>
<td>04</td>
<td>05</td>
<td>06</td>
<td>07</td>
<td>08</td>
<td>09</td>
<td>00</td>
<td>01</td>
<td>02</td>
<td>03</td>
<td>04</td>
<td>05</td>
<td>06</td>
<td>07</td>
<td>08</td>
<td>09</td>
</tr>
</tbody>
</table>
</div>
C. Top and bottom scrollbar, a little more hacky. Needs some javascript. Use the solution form A above and add a 'fake' div for top scrollbar:
<div class="large-table-fake-top-scroll-container-3">
<div> </div>
</div>
<div class="large-table-container-3">
<table>...</table>
</div>
.large-table-container-3 {
max-width: 200px;
overflow-x: scroll;
overflow-y: auto;
}
.large-table-container-3 table {
}
.large-table-fake-top-scroll-container-3 {
max-width: 200px;
overflow-x: scroll;
overflow-y: auto;
}
.large-table-fake-top-scroll-container-3 div {
background-color: red;/*Just for test, to see the 'fake' div*/
font-size:1px;
line-height:1px;
}
And a little javascript to catch the scroll on the top fake div and then scroll the table container (and vice-versa), with jQuery:
$(function() {
var tableContainer = $(".large-table-container-3");
var table = $(".large-table-container-3 table");
var fakeContainer = $(".large-table-fake-top-scroll-container-3");
var fakeDiv = $(".large-table-fake-top-scroll-container-3 div");
var tableWidth = table.width();
fakeDiv.width(tableWidth);
fakeContainer.scroll(function() {
tableContainer.scrollLeft(fakeContainer.scrollLeft());
});
})
Demo:
$(function() {
var tableContainer = $(".large-table-container-3");
var table = $(".large-table-container-3 table");
var fakeContainer = $(".large-table-fake-top-scroll-container-3");
var fakeDiv = $(".large-table-fake-top-scroll-container-3 div");
var tableWidth = table.width();
fakeDiv.width(tableWidth);
fakeContainer.scroll(function() {
tableContainer.scrollLeft(fakeContainer.scrollLeft());
});
tableContainer.scroll(function() {
fakeContainer.scrollLeft(tableContainer.scrollLeft());
});
})
.large-table-container-3 {
max-width: 200px;
overflow-x: scroll;
overflow-y: auto;
}
.large-table-container-3 table {
}
.large-table-fake-top-scroll-container-3 {
max-width: 200px;
overflow-x: scroll;
overflow-y: auto;
}
.large-table-fake-top-scroll-container-3 div {
background-color: red;
font-size:1px;
line-height:1px;
}
/*misc*/
td {
border: 1px solid gray;
}
th {
text-align: left;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="large-table-fake-top-scroll-container-3">
<div> </div>
</div>
<div class="large-table-container-3">
<table>
<thead>
<tr>
<th colspan="20">
Top and bottom scrollbar:
</th>
</tr>
</thead>
<tbody>
<tr>
<td>00</td>
<td>01</td>
<td>02</td>
<td>03</td>
<td>04</td>
<td>05</td>
<td>06</td>
<td>07</td>
<td>08</td>
<td>09</td>
<td>00</td>
<td>01</td>
<td>02</td>
<td>03</td>
<td>04</td>
<td>05</td>
<td>06</td>
<td>07</td>
<td>08</td>
<td>09</td>
</tr>
</tbody>
</table>
</div>
The working complete code for all three solutions:
$(function() {
var tableContainer = $(".large-table-container-3");
var table = $(".large-table-container-3 table");
var fakeContainer = $(".large-table-fake-top-scroll-container-3");
var fakeDiv = $(".large-table-fake-top-scroll-container-3 div");
var tableWidth = table.width();
fakeDiv.width(tableWidth);
fakeContainer.scroll(function() {
tableContainer.scrollLeft(fakeContainer.scrollLeft());
});
tableContainer.scroll(function() {
fakeContainer.scrollLeft(tableContainer.scrollLeft());
});
})
/*Bottom scrollbar*/
.large-table-container-1 {
max-width: 200px;
overflow-x: scroll;
overflow-y: auto;
}
.large-table-container-1 table {
}
/*Top scrollbar*/
.large-table-container-2 {
max-width: 200px;
overflow-x: scroll;
overflow-y: auto;
transform:rotateX(180deg);
}
.large-table-container-2 table {
transform:rotateX(180deg);
}
/*Top and bottom scrollbar*/
.large-table-container-3 {
max-width: 200px;
overflow-x: scroll;
overflow-y: auto;
}
.large-table-container-3 table {
}
.large-table-fake-top-scroll-container-3 {
max-width: 200px;
overflow-x: scroll;
overflow-y: auto;
}
.large-table-fake-top-scroll-container-3 div {
background-color: red;
font-size:1px;
line-height:1px;
}
/*misc*/
td {
border: 1px solid gray;
}
th {
text-align: left;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="large-table-container-1">
<table>
<thead>
<tr>
<th colspan="20">
Bottom scrollbar:
</th>
</tr>
</thead>
<tbody>
<tr>
<td>00</td>
<td>01</td>
<td>02</td>
<td>03</td>
<td>04</td>
<td>05</td>
<td>06</td>
<td>07</td>
<td>08</td>
<td>09</td>
<td>00</td>
<td>01</td>
<td>02</td>
<td>03</td>
<td>04</td>
<td>05</td>
<td>06</td>
<td>07</td>
<td>08</td>
<td>09</td>
</tr>
</tbody>
</table>
</div>
<div class="large-table-container-2">
<table>
<thead>
<tr>
<th colspan="20">
Top scrollbar:
</th>
</tr>
</thead>
<tbody>
<tr>
<td>00</td>
<td>01</td>
<td>02</td>
<td>03</td>
<td>04</td>
<td>05</td>
<td>06</td>
<td>07</td>
<td>08</td>
<td>09</td>
<td>00</td>
<td>01</td>
<td>02</td>
<td>03</td>
<td>04</td>
<td>05</td>
<td>06</td>
<td>07</td>
<td>08</td>
<td>09</td>
</tr>
</tbody>
</table>
</div>
<div class="large-table-fake-top-scroll-container-3">
<div> </div>
</div>
<div class="large-table-container-3">
<table>
<thead>
<tr>
<th colspan="20">
Top and bottom scrollbar:
</th>
</tr>
</thead>
<tbody>
<tr>
<td>00</td>
<td>01</td>
<td>02</td>
<td>03</td>
<td>04</td>
<td>05</td>
<td>06</td>
<td>07</td>
<td>08</td>
<td>09</td>
<td>00</td>
<td>01</td>
<td>02</td>
<td>03</td>
<td>04</td>
<td>05</td>
<td>06</td>
<td>07</td>
<td>08</td>
<td>09</td>
</tr>
</tbody>
</table>
</div>
这篇关于如何将 dataTables 的水平滚动条放在表格顶部?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何将 dataTables 的水平滚动条放在表格顶部?
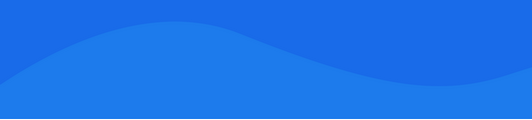
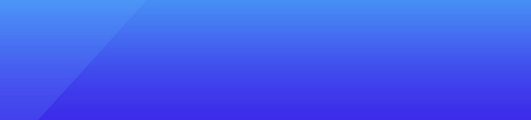
基础教程推荐
- Vue 3 – <过渡>渲染不能动画的非元素根节点 2022-01-01
- 直接将值设置为滑块 2022-01-01
- Electron 将 Node.js 和 Chromium 上下文结合起来意味着 2022-01-01
- 用于 Twitter 小部件宽度的 HTML/CSS 2022-01-01
- html表格如何通过更改悬停边框来突出显示列? 2022-01-01
- 自定义 XMLHttpRequest.prototype.open 2022-01-01
- 我可以在浏览器中与Babel一起使用ES模块,而不捆绑我的代码吗? 2022-01-01
- Chart.js 在线性图表上拖动点 2022-01-01
- 如何使用TypeScrip将固定承诺数组中的项设置为可选 2022-01-01
- 如何使用JIT在顺风css中使用布局变体? 2022-01-01