CryptoKey ArrayBuffer to base64 and Back(CryptoKey ArrayBuffer 到 base64 并返回)
问题描述
我想知道如何解决这个问题.我使用 WebCrypto API 生成 RSA-OAEP 密钥对,然后从导出为 ArrayBuffer 的密钥对中导出 pkcs8 中的私钥,我想将此 ArrayBuffer 编码为 base64,以便将其存储为 PEM.
I was wondering how do I solve this problem. I generate RSA-OAEP keypair using WebCrypto API, then I export private key in pkcs8 from the keypair which exports as ArrayBuffer and I want to encode this ArrayBuffer into base64 so I can store it as a PEM.
在此测试示例中,我将密钥导出为 pkcs8 并将此 pkcs8 导入回 CryptoKey.问题是有时有效,有时无效.
In this testing example I am exporting key as pkcs8 and importing this pkcs8 back to CryptoKey. The problem is that sometimes it works and sometimes it does not.
这些是代码的结果:注意:只发生这些状态之一,而不是同时发生.NOTE2:本例不包含 -----BEGIN PRIVATE KEY----- 前缀和后缀,我只是对密钥进行编码.
These are results of the code: NOTE: Only happens one of these states not all at once. NOTE2: This example does not contain -----BEGIN PRIVATE KEY----- prefix and suffix I am only encoding the key.
Case1: Uncaught (in promise) URIError: URI malformed(...)b64DecodeUnicode @ try.php:20b64toab @ try.php:70wayBack @ try.php:66(anonymous function) @ try.php:56
Case1: Uncaught (in promise) URIError: URI malformed(…)b64DecodeUnicode @ try.php:20b64toab @ try.php:70wayBack @ try.php:66(anonymous function) @ try.php:56
Case2: undefined:1 Uncaught (in promise) DOMException
Case2: undefined:1 Uncaught (in promise) DOMException
案例 3:好的 - 一直有效.
Case3: OK - works all the way back.
我不知道是什么导致了错误,但我认为这与 base64 编码有关.正如我所说,有时私钥会生成 OK,有时则不会.
I don't know what causes the errors but I think it has something to do with base64 encoding. As I said sometimes private key generates OK and sometimes not.
非常感谢您提前提供的每一个帮助.
Thank you very much for every help in advance.
function b64EncodeUnicode(str) {
return btoa(encodeURIComponent(str).replace(/%([0-9A-F]{2})/g, function(match, p1) {
return String.fromCharCode('0x' + p1);
}));
}
function b64DecodeUnicode(str) {
return decodeURIComponent(Array.prototype.map.call(atob(str), function(c) {
return '%' + ('00' + c.charCodeAt(0).toString(16)).slice(-2);
}).join(''));
}
function addNewLines(str) {
var finalString = '';
for(var i=0; i < str.length; i++) {
finalString += str.substring(0, 64) + '
';
str = str.substring(64);
}
return finalString;
}
window.crypto.subtle.generateKey(
{
name: "RSA-OAEP",
modulusLength: 2048,
publicExponent: new Uint8Array([0x01, 0x00, 0x01]),
hash: {name: "SHA-256"}
},
true,
["encrypt", "decrypt"]
).then(function(keyPair) {
window.crypto.subtle.exportKey(
"pkcs8",
keyPair.privateKey
).then(function(exportedPrivateKey) {
var byteArray = new Uint8Array(exportedPrivateKey);
console.log(byteArray);
var byteString = '';
for(var i=0; i < byteArray.byteLength; i++) {
byteString += String.fromCodePoint(byteArray[i]);
}
wayBack(addNewLines(b64EncodeUnicode(byteString)));
});
});
function wayBack(pem) {
var lines = pem.split('
');
var encodedString = '';
for(var i=0; i < lines.length; i++) {
encodedString += lines[i].trim();
}
b64toab(encodedString);
}
function b64toab(b64) {
var byteString = b64DecodeUnicode(b64);
console.log(byteString);
var byteArray = new Uint8Array(byteString.length);
for(var i=0; i < byteString.length; i++) {
byteArray[i] = byteString.codePointAt(i);
}
console.log(byteArray);
window.crypto.subtle.importKey(
"pkcs8",
byteArray,
{
name: "RSA-OAEP",
hash: {name: "SHA-256"}
},
true,
["decrypt"]
).then(function(importedPrivateKey) {
console.log(importedPrivateKey);
});
}
推荐答案
当您将字符串拆分为 64 个字符的块时,您忘记包含 PEM 的最后一部分.只需将 finalString += str;
添加到 addNewLines
You forgot to include the last part of PEM when you split the string in blocks of 64 characters. Just add finalString += str;
to addNewLines
function addNewLines(str) {
var finalString = '';
for(var i=0; i < str.length; i++) {
finalString += str.substring(0, 64) + '
';
str = str.substring(64);
}
finalString += str;
return finalString;
}
我已经重构了您的示例以查看发生了什么.如果您认为它有用,请使用以下代码
I have refactorized your example to see what is happening. Use the below code if you consider it useful
function b64EncodeUnicode(str) {
return btoa(encodeURIComponent(str).replace(/%([0-9A-F]{2})/g, function(match, p1) {
return String.fromCharCode('0x' + p1);
}));
}
function b64DecodeUnicode(str) {
return decodeURIComponent(Array.prototype.map.call(atob(str), function(c) {
return '%' + ('00' + c.charCodeAt(0).toString(16)).slice(-2);
}).join(''));
}
function addNewLines(str) {
var finalString = '';
for(var i=0; i < str.length; i++) {
finalString += str.substring(0, 64) + '
';
str = str.substring(64);
}
finalString += str;
return finalString;
}
function removeLines(pem) {
var lines = pem.split('
');
var encodedString = '';
for(var i=0; i < lines.length; i++) {
encodedString += lines[i].trim();
}
return encodedString;
}
function stringToArrayBuffer(byteString){
var byteArray = new Uint8Array(byteString.length);
for(var i=0; i < byteString.length; i++) {
byteArray[i] = byteString.codePointAt(i);
}
return byteArray;
}
function arrayBufferToString(exportedPrivateKey){
var byteArray = new Uint8Array(exportedPrivateKey);
var byteString = '';
for(var i=0; i < byteArray.byteLength; i++) {
byteString += String.fromCodePoint(byteArray[i]);
}
return byteString;
}
window.crypto.subtle.generateKey(
{
name: "RSA-OAEP",
modulusLength: 2048,
publicExponent: new Uint8Array([0x01, 0x00, 0x01]),
hash: {name: "SHA-256"}
},
true,
["encrypt", "decrypt"]
).then(function(keyPair) {
window.crypto.subtle.exportKey(
"pkcs8",
keyPair.privateKey
).then(function(exportedPrivateKey) {
var privateKeyDer = arrayBufferToString(exportedPrivateKey); //pkcs#8 to DER
var privateKeyB64 = b64EncodeUnicode(privateKeyDer); //btoa(privateKeyDer);
var privateKeyPEMwithLines = addNewLines(privateKeyB64); //split PEM into 64 character strings
var privateKeyPEMwithoutLines = removeLines(privateKeyPEMwithLines); //join PEM
var privateKeyDerDecoded = b64DecodeUnicode(privateKeyPEMwithoutLines); // atob(privateKeyB64);
var privateKeyArrayBuffer = stringToArrayBuffer(privateKeyDerDecoded); //DER to arrayBuffer
window.crypto.subtle.importKey( //importKEy
"pkcs8",
privateKeyArrayBuffer,
{
name: "RSA-OAEP",
hash: {name: "SHA-256"}
},
true,
["decrypt"]
).then(function(importedPrivateKey) {
console.log(importedPrivateKey);
});
});
});
这篇关于CryptoKey ArrayBuffer 到 base64 并返回的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:CryptoKey ArrayBuffer 到 base64 并返回
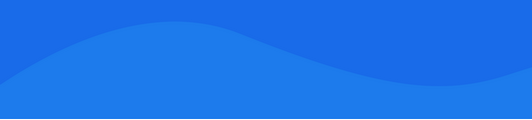
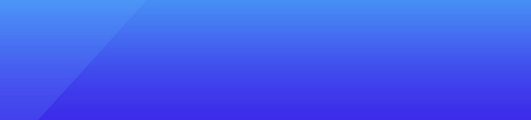
基础教程推荐
- 我可以在浏览器中与Babel一起使用ES模块,而不捆绑我的代码吗? 2022-01-01
- Electron 将 Node.js 和 Chromium 上下文结合起来意味着 2022-01-01
- 用于 Twitter 小部件宽度的 HTML/CSS 2022-01-01
- 如何使用JIT在顺风css中使用布局变体? 2022-01-01
- html表格如何通过更改悬停边框来突出显示列? 2022-01-01
- 直接将值设置为滑块 2022-01-01
- 自定义 XMLHttpRequest.prototype.open 2022-01-01
- Chart.js 在线性图表上拖动点 2022-01-01
- 如何使用TypeScrip将固定承诺数组中的项设置为可选 2022-01-01
- Vue 3 – <过渡>渲染不能动画的非元素根节点 2022-01-01