VueJS display grouped array of objects(VueJS 显示分组的对象数组)
问题描述
我正在从 API 中获取国家/地区列表,我想按次区域(大陆)分组显示它们.像这样:
I'm fetching a list of countries from the API and I want to display them in groups by subregion (continent). Like that:
API 给我一个响应,它是一个对象(国家)数组,每个对象都有一个名为子区域"的键 - 我想按该键进行分组.我使用lodash进行分组,但也许有一个我不熟悉的Vue方法.我的 JS 代码:
API gives me a response which is an array of objects (countries) and for each object there is a key called 'subregion' - I want to group by that key. I use lodash for grouping, but maybe there is a Vue method I'm not familiar with. My JS code:
var app = new Vue({
el: '#app',
data: {
countries: null,
},
created: function () {
this.fetchData();
},
methods: {
fetchData: function() {
var xhr = new XMLHttpRequest();
var self = this;
xhr.open('GET', apiURL);
xhr.onload = function() {
var countryList = JSON.parse(xhr.responseText);
self.countries = _.groupBy(countryList, "subregion");
};
xhr.send();
},
},
});
还有 HTML:
<li v-for="country in countries">
{{ country.name }} (+{{ country.callingCodes[0] }})
</li>
我怎样才能实现图片中的内容?
How can I achieve what's in the picture?
推荐答案
您已经使用 lodash 和以下代码正确地按子区域对国家进行了分组.
You've correctly grouped countries by their subregion using lodash with the following code.
_.groupBy(countryList, "subregion")
这为您提供了一个对象,其键是子区域的名称,值是具有此类子区域的对象数组.
This has given you an object whose keys are names of subregions, and the values are arrays of objects with such subregion.
所以您的错误是您希望 countries
中的值包含 name
.相反,它包含一个 array 具有 name
s 的对象.
So your mistake is that you expect a value in countries
to contain name
. Instead, it contains an array of objects with name
s.
为此,您需要两个 for 循环.
You need two for-loops for this.
这是一个普通的实现.这里也是一个bin.
Here's a vanilla implementation. Here's a bin, too.
fetch('https://restcountries.eu/rest/v2/all')
.then(r => r.json())
.then(data => {
const grouped = _.groupBy(data, 'subregion')
const listOfSubregions = Object.keys(grouped)
listOfSubregions.forEach(key => {
console.log(' =========== ' + key + ' =========== ')
const countriesInThisSubregion = grouped[key]
countriesInThisSubregion.forEach(country => {
console.log(country.name + ' ' + country.callingCodes[0])
})
})
})
使用 Vue,您将拥有类似以下的内容(未经测试,但根据上面的代码应该非常容易推断).
With Vue, you'd have something like the following (not tested, but should be super-easy to deduce based on the above code).
<li v-for="subregion in subregions">
<ul>
<li v-for="country of subregion">
{{ country.name }} ({{ country.callingCodes[0] }})
</li>
</ul>
</li>
这篇关于VueJS 显示分组的对象数组的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:VueJS 显示分组的对象数组
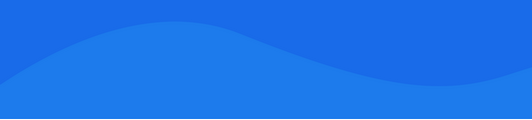
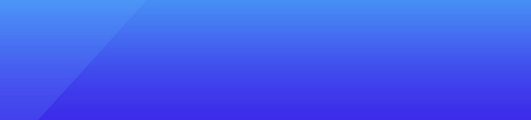
基础教程推荐
- 如何使用JIT在顺风css中使用布局变体? 2022-01-01
- Vue 3 – <过渡>渲染不能动画的非元素根节点 2022-01-01
- 直接将值设置为滑块 2022-01-01
- html表格如何通过更改悬停边框来突出显示列? 2022-01-01
- 我可以在浏览器中与Babel一起使用ES模块,而不捆绑我的代码吗? 2022-01-01
- 用于 Twitter 小部件宽度的 HTML/CSS 2022-01-01
- Chart.js 在线性图表上拖动点 2022-01-01
- 如何使用TypeScrip将固定承诺数组中的项设置为可选 2022-01-01
- 自定义 XMLHttpRequest.prototype.open 2022-01-01
- Electron 将 Node.js 和 Chromium 上下文结合起来意味着 2022-01-01