How can I migrate my code to Discord.js v12 from v11?(如何将我的代码从 v11 迁移到 Discord.js v12?)
问题描述
我升级到 Discord.js v12,但它破坏了我现有的 v11 代码.以下是一些导致错误的示例:
I upgraded to Discord.js v12, but it broke my existing v11 code. Here are some examples of things that cause errors:
// TypeError: client.users.get is not a function
const user = client.users.get('123456789012345678')
// TypeError: message.guild.roles.find is not a function
const role = message.guild.roles.find(r => r.name === 'Admin')
// TypeError: message.member.addRole is not a function
await message.member.addRole(role)
// TypeError: message.guild.createChannel is not a function
await message.guild.createChannel('welcome')
// TypeError: message.channel.fetchMessages is not a function
const messages = await message.channel.fetchMessages()
const {RichEmbed} = require('discord.js')
// TypeError: RichEmbed is not a constructor
const embed = new RichEmbed()
const connection = await message.channel.join()
// TypeError: connection.playFile is not a function
const dispatcher = connection.playFile('./music.mp3')
如何将我的代码迁移到 Discord.js v12 并修复这些错误?我在哪里可以看到引入的重大更改 v12?
How can I migrate my code to Discord.js v12 and fix these errors? Where can I see the breaking changes v12 introduced?
推荐答案
以下是人们遇到的 Discord.js v12 中引入的一些最常见的重大更改.
Here are some of the most common breaking changes introduced in Discord.js v12 that people run into.
Client#users
和 Guild#roles
等属性现在是 managers,而不是缓存的 Collection
的项目.要访问此集合,请使用 cache
属性:
Properties such as Client#users
and Guild#roles
are now managers, instead of the cached Collection
of items. To access this collection, use the cache
property:
const user = client.users.cache.get('123456789012345678')
const role = message.guild.roles.cache.find(r => r.name === 'Admin')
此外,GuildMember#addRole
、Guild#createChannel
和 TextBasedChannel#fetchMessages
等方法已移至各自的管理器:
In addition, methods such as GuildMember#addRole
, Guild#createChannel
, and TextBasedChannel#fetchMessages
have moved to the respective managers:
await message.member.roles.add(role)
await message.guild.channels.create('welcome')
const messages = await message.channel.messages.fetch()
集合
Collection
类(例如 client.users.cache
、guild.roles.cache
、guild.channels.cache
)现在只接受 functions,而不是属性键和值,用于 .find
和 .findKey
:
Collection
The Collection
class (e.g. client.users.cache
, guild.roles.cache
, guild.channels.cache
) now only accepts functions, not property keys and values, for .find
and .findKey
:
// v11: collection.find('property', 'value')
collection.find(item => item.property === 'value')
.exists
、.deleteAll
、.filterArray
、.findAll
也已被删除:
// v11: collection.exists('property', 'value')
collection.some(item => item.property === 'value')
// v11: collection.deleteAll()
Promise.all(collection.map(item => item.delete()))
// v11: collection.filterArray(fn)
collection.filter(fn).array()
// v11: collection.findAll('property', value')
collection.filter(item => item.property === 'value').array()
.tap
现在在集合上运行一个函数,而不是在集合中的每个项目上运行:
.tap
now runs a function on the collection instead of every item in the collection:
// v11: collection.tap(item => console.log(item))
collection.each(item => console.log(item))
// New .tap behaviour:
collection.tap(coll => console.log(`${coll.size} items`))
RichEmbed
/MessageEmbed
RichEmbed
类已被移除;使用 MessageEmbed
类,现在用于所有嵌入(而不是仅接收到的嵌入).
RichEmbed
/MessageEmbed
The RichEmbed
class has been removed; use the MessageEmbed
class instead which is now used for all embeds (instead of just received embeds).
const {MessageEmbed} = require('discord.js')
const embed = new MessageEmbed()
addBlankField
方法也已被删除.该方法只是简单地添加了一个带有零宽度空格 (u200B
) 的字段作为名称和值,因此要添加一个空白字段,请执行以下操作:
The addBlankField
method has also been removed. This method simply added a field with a zero-width space (u200B
) as the name and value, so to add a blank field do this:
embed.addField('u200B', 'u200B')
语音
所有 VoiceConnection
/VoiceBroadcast#play***
方法已统一在一个 play
方法:
Voice
All of the VoiceConnection
/VoiceBroadcast#play***
methods have been unified under a single play
method:
const dispatcher = connection.play('./music.mp3')
Client#createVoiceBroadcast
已移至 ClientVoiceManager
:
const broadcast = client.voice.createVoiceBroadcast()
此外,StreamDispatcher
扩展了 Node.js 的 stream.Writable
,所以使用 dispatcher.destroy()
而不是 dispatcher.end()
.end
事件已被移除,取而代之的是原生 finish
事件.
Additionally, StreamDispatcher
extends Node.js' stream.Writable
, so use dispatcher.destroy()
instead of dispatcher.end()
. The end
event has been removed in favour of the native finish
event.
User#displayAvatarURL
和 Guild#iconURL
等属性现在是方法:
Properties such as User#displayAvatarURL
and Guild#iconURL
are now methods:
const avatar = user.displayAvatarURL()
const icon = mesage.guild.iconURL()
您还可以传递 ImageURLOptions
自定义格式和大小等内容.
You can also pass an ImageURLOptions
to customise things like format and size.
要了解有关 v12 重大更改的更多信息,请查看 the更新指南和changelog.文档也是寻找特定方法的好资源/属性.
To find out more about the v12 breaking changes, take a look at the updating guide and the changelog. The documentation is also a good resource for finding a particular method/property.
这篇关于如何将我的代码从 v11 迁移到 Discord.js v12?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何将我的代码从 v11 迁移到 Discord.js v12?
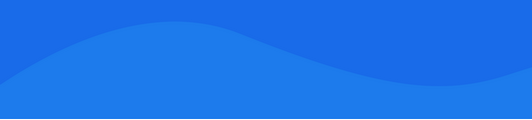
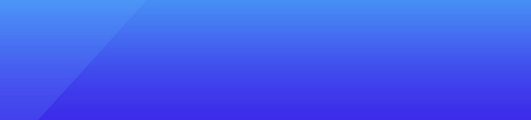
基础教程推荐
- Chart.js 在线性图表上拖动点 2022-01-01
- 自定义 XMLHttpRequest.prototype.open 2022-01-01
- 直接将值设置为滑块 2022-01-01
- 我可以在浏览器中与Babel一起使用ES模块,而不捆绑我的代码吗? 2022-01-01
- 如何使用JIT在顺风css中使用布局变体? 2022-01-01
- html表格如何通过更改悬停边框来突出显示列? 2022-01-01
- 如何使用TypeScrip将固定承诺数组中的项设置为可选 2022-01-01
- Electron 将 Node.js 和 Chromium 上下文结合起来意味着 2022-01-01
- Vue 3 – <过渡>渲染不能动画的非元素根节点 2022-01-01
- 用于 Twitter 小部件宽度的 HTML/CSS 2022-01-01