Leaflet custom coordinates on image(图片上的传单自定义坐标)
问题描述
我有一个尺寸为 8576x8576px 的图像,我想让坐标匹配 1:1.我还想要图像中心的坐标 0,0(现在中心是 -128,128).我也想显示坐标.我想为用户插入坐标放置一个定位按钮,然后在地图上找到它们.像这样:http://xero-hurtworld.com/map_steam.php(我使用相同的图像但更大).我设置的 tile 大小为 268px.
I have an image which size is 8576x8576px, and I want to make the coordinates match 1:1. Also I want the coordinates 0,0 in the center of the image (now the center is -128,128). And I want to show the coordinates too. I want to put a locate button for the user insert coordinates and then find them on the map. Something like this: http://xero-hurtworld.com/map_steam.php (I am using the same image but bigger). The tile size I made its 268px.
到目前为止我的代码:
https://jsfiddle.net/ze62dte0/
<!DOCTYPE html>
<html>
<head>
<title>Map</title>
<meta charset="utf-8"/>
<meta name="viewport" content="initial-scale=1.0, user-scalable=no"/>
<link rel="stylesheet" href="http://cdn.leafletjs.com/leaflet-0.6.4/leaflet.css" />
<!--[if lte IE 8]>
<link rel="stylesheet" href="http://cdn.leafletjs.com/leaflet-0.6.4/leaflet.ie.css" />
<![endif]-->
<script src="http://cdn.leafletjs.com/leaflet-0.6.4/leaflet.js" charset="utf-8"></script>
<script>
function init() {
var mapMinZoom = 0;
var mapMaxZoom = 3;
var map = L.map('map', {
maxZoom: mapMaxZoom,
minZoom: mapMinZoom,
crs: L.CRS.Simple
}).setView([0, 0], mapMaxZoom);
window.latLngToPixels = function(latlng){
return window.map.project([latlng.lat,latlng.lng], window.map.getMaxZoom());
};
window.pixelsToLatLng = function(x,y){
return window.map.unproject([x,y], window.map.getMaxZoom());
};
var mapBounds = new L.LatLngBounds(
map.unproject([0, 8576], mapMaxZoom),
map.unproject([8576, 0], mapMaxZoom));
map.fitBounds(mapBounds);
L.tileLayer('{z}/{x}/{y}.jpg', {
minZoom: mapMinZoom, maxZoom: mapMaxZoom,
bounds: mapBounds,
noWrap: true,
tms: false
}).addTo(map);
L.marker([0, 0]).addTo(map).bindPopup("Zero");
L.marker([-128, 128]).addTo(map).bindPopup("center");
var popup = L.popup();
<!-- Click pop-up>
var popup = L.popup();
function onMapClick(e) {
popup
.setLatLng(e.latlng)
.setContent("You clicked in " + e.latlng.toString ())
.openOn(map);
}
map.on('click', onMapClick);
}
</script>
<style>
html, body, #map { width:100%; height:100%; margin:0; padding:0; }
</style>
</head>
<body onload="init()">
<div id="map"></div>
</body>
</html>
推荐答案
如果我理解正确,你想要一个类似于 L.CRS.Simple
的 CRS,它放置 tile 0/0/0(tile大小为 268px,即 8576/2⁵),这样:
If I understand correctly, you want a CRS similar to L.CRS.Simple
that places tile 0/0/0 (tile size 268px, which is 8576 / 2⁵) so that:
- 位置
[0, 0]
位于该图块的中心. - 整个世界(即整个 tile 0/0/0)从位置
[-8576/2, -8576/2]
到[8576/2, 8576/2]
.
- Position
[0, 0]
is at the center of that tile. - The entire world (i.e. entire tile 0/0/0) goes from position
[-8576/2, -8576/2]
to[8576/2, 8576/2]
.
您只需要使用适当的转换来调整 L.CRS.Simple
,以说明 1/2⁵ = 1/32(而不仅仅是 1)的比例和 8576 的偏移量* 1/32/2 = 268/2 = 134(而不是 0.5).
You would just need to adjust the L.CRS.Simple
with the appropriate transformation, to account for this scale of 1/2⁵ = 1/32 (instead of just 1) and offset of 8576 * 1/32 / 2 = 268 / 2 = 134 (instead of 0.5).
L.CRS.MySimple = L.extend({}, L.CRS.Simple, {
transformation: new L.Transformation(1 / 32, 134, -1 / 32, 134)
});
var map = L.map('map', {
maxZoom: mapMaxZoom,
minZoom: mapMinZoom,
crs: L.CRS.MySimple
}).setView([0, 0], mapMaxZoom);
演示:http://plnkr.co/edit/5SQqp7SP4nf8muPM5iso?p=preview(我使用 Plunker 而不是 jsfiddle,因为您提供了带有 HTML 的完整页面代码,而 jsfiddle 希望您将 HTML、CSS 和 JavaScript 代码拆分为单独的块).
Demo: http://plnkr.co/edit/5SQqp7SP4nf8muPM5iso?p=preview (I used Plunker instead of jsfiddle because you provided a full page code with HTML, whereas jsfiddle expects you to split your HTML, CSS and JavaScript codes into separate blocks).
至于显示坐标和定位"按钮,它很容易实现,因此与您提到的示例相似.如果您需要帮助,请随时提出新问题.
As for showing the coordinates and a "locate" button, it would be quite easy to implement so that it is similar to the example you mention. Feel free to open new questions if you need help.
在上面的演示中,我使用 Leaflet.Coordinates 插件 来快速实现这两个功能(参见地图左下角的控件;您必须开始在地图上移动鼠标才能显示坐标;单击该控件以打开编辑模式.
In the above demo, I used Leaflet.Coordinates plugin to implement quickly both functionalities (see the control on bottom left corner of the map; you have to start moving your mouse on the map for the coordinates to appear; click on that control to open the edition mode).
对于 Leaflet.Coordinates 插件,它将显示的坐标经度包裹起来以保持在 [-180;180] 度.
As for the Leaflet.Coordinates plugin, it wraps displayed coordinates longitude to stay within [-180; 180] degrees.
在坐标不是度数的情况下,包裹经度没有意义.
In your case where coordinates are not degrees, there is no point wrapping the longitude.
我认为这是造成点击弹窗与控件坐标不一致的原因.
I think this is the cause for the discrepancy of coordinates between the click popup and the control.
只需修补插件代码以防止包装:
Simply patch the plugin code to prevent wrapping:
// Patch first to avoid longitude wrapping.
L.Control.Coordinates.include({
_update: function(evt) {
var pos = evt.latlng,
opts = this.options;
if (pos) {
//pos = pos.wrap(); // Remove that instruction.
this._currentPos = pos;
this._inputY.value = L.NumberFormatter.round(pos.lat, opts.decimals, opts.decimalSeperator);
this._inputX.value = L.NumberFormatter.round(pos.lng, opts.decimals, opts.decimalSeperator);
this._label.innerHTML = this._createCoordinateLabel(pos);
}
}
});
更新的演示:http://plnkr.co/edit/M3Ru0xqn6AxAaSb4kIJU?p=preview一个>
这篇关于图片上的传单自定义坐标的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:图片上的传单自定义坐标
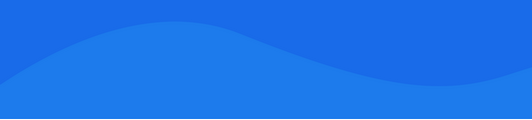
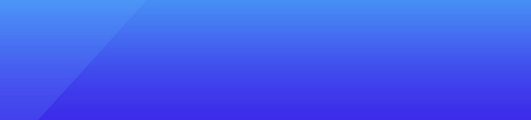
基础教程推荐
- 自定义 XMLHttpRequest.prototype.open 2022-01-01
- 如何使用TypeScrip将固定承诺数组中的项设置为可选 2022-01-01
- Chart.js 在线性图表上拖动点 2022-01-01
- 如何使用JIT在顺风css中使用布局变体? 2022-01-01
- Vue 3 – <过渡>渲染不能动画的非元素根节点 2022-01-01
- 我可以在浏览器中与Babel一起使用ES模块,而不捆绑我的代码吗? 2022-01-01
- Electron 将 Node.js 和 Chromium 上下文结合起来意味着 2022-01-01
- 直接将值设置为滑块 2022-01-01
- 用于 Twitter 小部件宽度的 HTML/CSS 2022-01-01
- html表格如何通过更改悬停边框来突出显示列? 2022-01-01