How can I upload an embedded image with JavaScript?(如何使用 JavaScript 上传嵌入的图像?)
问题描述
我想构建一个包含 JavaScript 的简单 HTML 页面,以执行表单 POST,其中包含嵌入在 HTML 中的图像数据与磁盘文件.
我看过这篇可以处理常规表单数据的帖子,但我对图像数据感到困惑.
I'd like to build a simple HTML page that includes JavaScript to perform a form POST with image data that is embedded in the HTML vs a file off disk.
I've looked at this post which would work with regular form data but I'm stumped on the image data.
JavaScript post request like a form submit
** UPDATE ** Feb. 2014 **
New and improved version available as a jQuery plugin: https://github.com/CoeJoder/jquery.image.blob
Usage:
$('img').imageBlob().ajax('/upload', {
complete: function(jqXHR, textStatus) { console.log(textStatus); }
});
Requirements
- the canvas element (HTML 5)
- FormData
- XMLHttpRequest.send(:FormData)
- Blob constructor
- Uint8Array
- atob(), escape()
Thus the browser requirements are:
- Chrome: 20+
- Firefox: 13+
- Internet Explorer: 10+
- Opera: 12.5+
- Safari: 6+
Note: The images must be of the same-origin as your JavaScript, or else the browser security policy will prevent calls to canvas.toDataURL()
(for more details, see this SO question: Why does canvas.toDataURL() throw a security exception?). A proxy server can be used to circumvent this limitation via response header injection, as described in the answers to that post.
Here is a jsfiddle of the below code. It should throw an error message, because it's not submitting to a real URL ('/some/url'). Use firebug or a similar tool to inspect the request data and verify that the image is serialized as form data (click "Run" after the page loads):
Example Markup
<img id="someImage" src="../img/logo.png"/>
The JavaScript
(function() {
// access the raw image data
var img = document.getElementById('someImage');
var canvas = document.createElement('canvas');
var ctx = canvas.getContext('2d');
canvas.width = img.width;
canvas.height = img.height;
ctx.drawImage(img, 0, 0);
var dataUrl = canvas.toDataURL('image/png');
var blob = dataUriToBlob(dataUrl);
// submit as a multipart form, along with any other data
var form = new FormData();
var xhr = new XMLHttpRequest();
xhr.open('POST', '/some/url', true); // plug-in desired URL
xhr.onreadystatechange = function() {
if (xhr.readyState == 4) {
if (xhr.status == 200) {
alert('Success: ' + xhr.responseText);
} else {
alert('Error submitting image: ' + xhr.status);
}
}
};
form.append('param1', 'value1');
form.append('param2', 'value2');
form.append('theFile', blob);
xhr.send(form);
function dataUriToBlob(dataURI) {
// serialize the base64/URLEncoded data
var byteString;
if (dataURI.split(',')[0].indexOf('base64') >= 0) {
byteString = atob(dataURI.split(',')[1]);
}
else {
byteString = unescape(dataURI.split(',')[1]);
}
// parse the mime type
var mimeString = dataURI.split(',')[0].split(':')[1].split(';')[0]
// construct a Blob of the image data
var array = [];
for(var i = 0; i < byteString.length; i++) {
array.push(byteString.charCodeAt(i));
}
return new Blob(
[new Uint8Array(array)],
{type: mimeString}
);
}
})();
References
SO: 'Convert DataURI to File and append to FormData
这篇关于如何使用 JavaScript 上传嵌入的图像?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何使用 JavaScript 上传嵌入的图像?
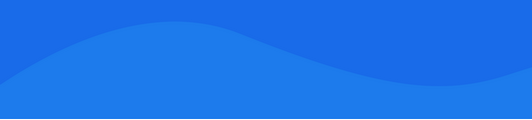
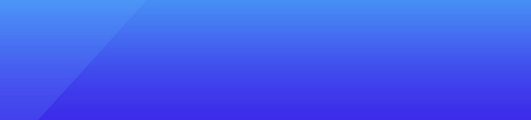
基础教程推荐
- 直接将值设置为滑块 2022-01-01
- Chart.js 在线性图表上拖动点 2022-01-01
- Electron 将 Node.js 和 Chromium 上下文结合起来意味着 2022-01-01
- 用于 Twitter 小部件宽度的 HTML/CSS 2022-01-01
- 如何使用JIT在顺风css中使用布局变体? 2022-01-01
- 我可以在浏览器中与Babel一起使用ES模块,而不捆绑我的代码吗? 2022-01-01
- 如何使用TypeScrip将固定承诺数组中的项设置为可选 2022-01-01
- html表格如何通过更改悬停边框来突出显示列? 2022-01-01
- Vue 3 – <过渡>渲染不能动画的非元素根节点 2022-01-01
- 自定义 XMLHttpRequest.prototype.open 2022-01-01