How would I dynamically create input boxes on the fly?(我将如何动态创建输入框?)
问题描述
我想使用 HTML 下拉框的值并在下面创建该数量的输入框.我希望我能在飞行中实现这一目标.此外,如果值发生变化,它应该适当地添加或删除.
我需要用什么编程语言来做这件事?我正在为整个网站使用 PHP.
这是一个使用 jQuery 实现目标的示例:
假设你有以下 html:
<div><选择 id="input_count"><option value="1">1个输入</option><option value="2">2 个输入</option><option value="3">3 个输入</option></选择><div id="输入"></div>这是你的任务的 js 代码:
$('#input_count').change(function() {var selectObj = $(this);var selectedOption = selectObj.find(":selected");var selectedValue = selectedOption.val();var targetDiv = $("#inputs");targetDiv.html("");for(var i = 0; i < selectedValue; i++) {targetDiv.append($("<input/>"));}});
您可以将这段代码简化如下:
$('#input_count').change(function() {var selectedValue = $(this).val();var targetDiv = $("#inputs").html("");for(var i = 0; i < selectedValue; i++) {targetDiv.append($("<input/>"));}});
这是一个有效的小提琴示例:http://jsfiddle.net/melih/VnRBm/
您可以阅读有关 jQuery 的更多信息:http://jquery.com/
I want to use the value of a HTML dropdown box and create that number of input boxes underneath. I'm hoping I can achieve this on the fly. Also if the value changes it should add or remove appropriately.
What programming language would I need to do this in? I'm using PHP for the overall website.
解决方案 Here is an example that uses jQuery to achieve your goals:
Assume you have following html:
<div>
<select id="input_count">
<option value="1">1 input</option>
<option value="2">2 inputs</option>
<option value="3">3 inputs</option>
</select>
<div>
<div id="inputs"> </div>
And this is the js code for your task:
$('#input_count').change(function() {
var selectObj = $(this);
var selectedOption = selectObj.find(":selected");
var selectedValue = selectedOption.val();
var targetDiv = $("#inputs");
targetDiv.html("");
for(var i = 0; i < selectedValue; i++) {
targetDiv.append($("<input />"));
}
});
You can simplify this code as follows:
$('#input_count').change(function() {
var selectedValue = $(this).val();
var targetDiv = $("#inputs").html("");
for(var i = 0; i < selectedValue; i++) {
targetDiv.append($("<input />"));
}
});
Here is a working fiddle example: http://jsfiddle.net/melih/VnRBm/
You can read more about jQuery: http://jquery.com/
这篇关于我将如何动态创建输入框?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:我将如何动态创建输入框?
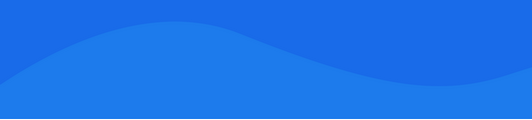
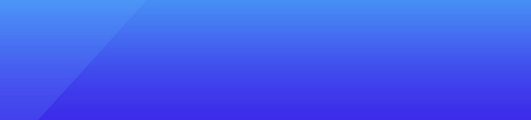
基础教程推荐
- html表格如何通过更改悬停边框来突出显示列? 2022-01-01
- 我可以在浏览器中与Babel一起使用ES模块,而不捆绑我的代码吗? 2022-01-01
- Electron 将 Node.js 和 Chromium 上下文结合起来意味着 2022-01-01
- 自定义 XMLHttpRequest.prototype.open 2022-01-01
- 直接将值设置为滑块 2022-01-01
- Vue 3 – <过渡>渲染不能动画的非元素根节点 2022-01-01
- Chart.js 在线性图表上拖动点 2022-01-01
- 如何使用JIT在顺风css中使用布局变体? 2022-01-01
- 用于 Twitter 小部件宽度的 HTML/CSS 2022-01-01
- 如何使用TypeScrip将固定承诺数组中的项设置为可选 2022-01-01