How do I add and remove classes to multiple child elements nested in an UL with a single click using Javascript?(如何使用 Javascript 通过单击向嵌套在 UL 中的多个子元素添加和删除类?)
问题描述
I want to start by apologizing for such a long question, I just hope I wont make it difficult to understand as a result.
I have created a side bar with three Menu elements in an UL which expand to show child elements, change background color and remove hover effect when clicked. I did this by defining a function that adds and remove classes containing relevant properties when the menu element is clicked.
The four specific things that I want the sidebar to do but cant seem to get it to do are as follows;
- only one selected/clicked item to expand at a time while all the rest of the unselected menu elements are collapsed. That means if I click the first item, it expands and when I click the second one, the first one collapses while the one I clicked expands etc.
- The selected/clicked element changes its background color to indicate it's selected.
- The selected/clicked element has no hover effect on the text while the unselected elements have a hover effect of text color change on them.
- I also want the selected menu element be able to toggle the expansion on and off not affecting the other elements in the UL.
I think where I'm having most trouble with my code is in the adding and removal of classes especially given that the <a>
Tags which are nested inside the <li>
Tags (clicked elements) are where the "hover" class needs to be added/removed, as well as the <ul>
Tags that expand are also nested inside the clicked elements.
function toggleMenu(e) {
var kids = document.querySelector("#menuList").children;
var unselectedLink = document.querySelectorAll(".unselected a");
var unselectedDropdown = document.querySelectorAll(".unselected ul");
//adds "unselected" class to all elements exept the selected one
for (var i = 0; i < kids.length; i++) {
kids[i].className = "unselected";
}
//adds "menuHover" class to all elements exept the selected element
for (var i = 0; i < unselectedLink.length; i++) {
unselectedLink[i].className = "menuHover";
}
for (var i = 0; i < unselectedDropdown.length; i++) {
unselectedDropdown[i].classList.remove("show")
}
//adds "selected" class, removes "menuHover" class and adds "toggle" to the selected element
e.className = "selected";
document.querySelector(".selected a").classList.remove("menuHover");
document.querySelector(".selected ul").classList.toggle("show");
}
.sidebar {
position: fixed;
width: 250px;
height: 100%;
left: 0px;
top: 0;
background: #1b1b1b;
font-family: sans-serif;
}
.menu-bar {
background: #1b1b1b;
height: 60px;
display: flex;
align-items: center;
padding-left: 42px;
}
.side-text {
color: #C5C5C5;
font-weight: bold;
font-size: 20px;
}
nav ul {
background: #1b1b1b;
height: 100%;
width: 100%;
list-style: none;
margin-left: 0;
padding-left: 0;
}
nav ul li {
line-height: 40px;
}
nav ul li a {
position: relative;
color: #C5C5C5;
text-decoration: none;
font-size: 14px;
padding-left: 43px;
font-weight: normal;
display: block;
width: 100%;
}
nav ul ul {
position: static;
display: none;
}
nav ul ul li a {
font-family: sans-serif;
font-size: 13px;
color: #e6e6e6;
padding-left: 80px;
font-weight: lighter;
}
.submenu-item:hover {
background: #1e1e1e!important;
}
/*...........selected and show..................*/
.selected {
background-color: #255DAA;
}
.show {
display: block;
}
/*...........unselected and hover..................*/
.unselected {
color: #1e1e1e;
}
.menuHover:hover {
color: #255DAA;
}
<nav class="sidebar">
<div class="menu-bar">
<label class="side-text">MENU</label>
</div>
<ul id="menuList">
<li class="selected" onclick="toggleMenu(this)">
<a href="#" class="" id="staff-btn">Staff</a>
<ul>
<li><a href="#">New Staff</a></li>
<li><a href="#">View Staff</a></li>
</ul>
</li>
<li class="unselected" onclick="toggleMenu(this)">
<a href="#" id="notes-btn" class="menuHover">Notes</a>
<ul>
<li><a href="#">New Note</a></li>
<li><a href="#">Edit Notes</a></li>
</ul>
</li>
<li class="unselected" onclick="toggleMenu(this)">
<a href="#" class="menuHover" id="tasks-btn">Tasks</a>
<ul>
<li><a href="#">New Tasks</a></li>
<li><a href="#">Edit Task</a></li>
</ul>
</li>
</nav>
I am quite close but somehow, logically I am doing things the wrong way with the JavaScript, so any adjustments to the code to make it reach all four of the above goals will be much appreciated. thanks
A simple way to do this:
If the element was selected, just unselect it
If it wasnt, unselect all elements and select the clicked element
function toggleMenu(el) {
if (el.classList.contains("selected")) {
el.classList.remove("selected");
el.classList.add("unselected");
}
else {
for (const child of document.getElementById("menuList").children) {
child.classList.remove("selected");
child.classList.add("unselected");
}
el.classList.remove("unselected");
el.classList.add("selected");
}
}
Edit 1 You can use the following css to unhide the submenu of a selected menuitem:
.selected ul {
display: block;
}
Edit 2 I went to the trouble of actually implementing it.
function toggleMenu(el) {
if (el.classList.contains("selected")) {
el.classList.remove("selected");
el.classList.add("unselected");
}
else {
for (const child of document.getElementById("menuList").children) {
child.classList.remove("selected");
child.classList.add("unselected");
}
el.classList.remove("unselected");
el.classList.add("selected");
}
}
.sidebar {
position: fixed;
width: 250px;
height: 100%;
left: 0px;
top: 0;
background: #1b1b1b;
font-family: sans-serif;
}
.menu-bar {
background: #1b1b1b;
height: 60px;
display: flex;
align-items: center;
padding-left: 42px;
}
.side-text {
color: #C5C5C5;
font-weight: bold;
font-size: 20px;
}
/* menu */
.menu {
background: #1b1b1b;
height: 100%;
width: 100%;
list-style: none;
margin-left: 0;
padding-left: 0;
}
.menu-item {
line-height: 40px;
}
.menu-item a {
position: relative;
color: #C5C5C5;
text-decoration: none;
font-size: 14px;
padding-left: 43px;
font-weight: normal;
display: block;
width: 100%;
}
/* submenu */
.submenu {
position: static;
display: none;
list-style: none;
}
.submenu-item a {
font-family: sans-serif;
font-size: 13px;
color: #e6e6e6;
padding-left: 80px;
font-weight: lighter;
}
.submenu-item:hover {
background: #1e1e1e;
}
/* selected and unselected */
.selected {
background-color: #255DAA;
}
.selected .submenu {
display: block;
}
.unselected {
color: #1e1e1e;
}
.unselected:hover a {
color: #255DAA;
}
<nav class="sidebar">
<div class="menu-bar">
<label class="side-text">MENU</label>
</div>
<ul class="menu" id="menuList">
<li class="menu-item selected" onclick="toggleMenu(this)">
<a href="#" id="staff-btn">Staff</a>
<ul class="submenu">
<li class="submenu-item"><a href="#">New Staff</a></li>
<li class="submenu-item"><a href="#">View Staff</a></li>
</ul>
</li>
<li class="menu-item unselected" onclick="toggleMenu(this)">
<a href="#" id="notes-btn">Notes</a>
<ul class="submenu">
<li class="submenu-item"><a href="#">New Note</a></li>
<li class="submenu-item"><a href="#">Edit Notes</a></li>
</ul>
</li>
<li class="menu-item unselected" onclick="toggleMenu(this)">
<a href="#" id="tasks-btn">Tasks</a>
<ul class="submenu">
<li class="submenu-item"><a href="#">New Tasks</a></li>
<li class="submenu-item"><a href="#">Edit Task</a></li>
</ul>
</li>
</ul>
</nav>
这篇关于如何使用 Javascript 通过单击向嵌套在 UL 中的多个子元素添加和删除类?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何使用 Javascript 通过单击向嵌套在 UL 中的多个
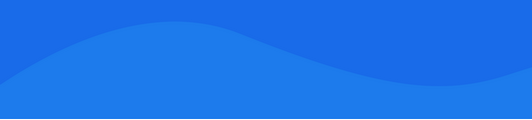
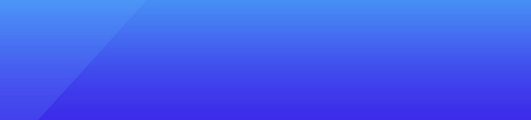
基础教程推荐
- Chart.js 在线性图表上拖动点 2022-01-01
- 用于 Twitter 小部件宽度的 HTML/CSS 2022-01-01
- 自定义 XMLHttpRequest.prototype.open 2022-01-01
- 如何使用TypeScrip将固定承诺数组中的项设置为可选 2022-01-01
- html表格如何通过更改悬停边框来突出显示列? 2022-01-01
- 如何使用JIT在顺风css中使用布局变体? 2022-01-01
- Electron 将 Node.js 和 Chromium 上下文结合起来意味着 2022-01-01
- 直接将值设置为滑块 2022-01-01
- Vue 3 – <过渡>渲染不能动画的非元素根节点 2022-01-01
- 我可以在浏览器中与Babel一起使用ES模块,而不捆绑我的代码吗? 2022-01-01