下面是“Servlet+JDBC实现登陆功能的小例子(带验证码)”的完整攻略,包含以下内容:
下面是“Servlet+JDBC实现登陆功能的小例子(带验证码)”的完整攻略,包含以下内容:
需求分析
我们需要实现一个包含验证码的登陆功能,用户需要输入用户名、密码和验证码,当用户点击提交按钮时,系统会校验用户的输入,如果输入正确,则跳转到登录成功页面,否则提示错误信息。
技术选择
我们使用Servlet技术实现用户输入数据的接收和校验,使用JDBC技术实现数据的存储和查询,使用JSP技术实现登录成功页面的展示。
开发步骤
步骤一:创建数据库和数据表
在MySQL数据库中创建一个名为“test”的数据库,并在该数据库中创建一个名为“user”的数据表,该数据表包含以下字段:
- id(int):主键字段;
- username(varchar):用户名字段;
- password(varchar):密码字段。
步骤二:创建JavaBean类
创建一个JavaBean类User,用于表示用户信息,该类的代码如下:
public class User {
private int id;
private String username;
private String password;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
步骤三:编写DAO层代码
编写一个UserDAO类,用于实现与数据库的数据交互,该类的代码如下:
public class UserDAO {
private Connection conn = null;
private PreparedStatement ps = null;
private ResultSet rs = null;
// 连接数据库方法
private void getConn() throws Exception {
Class.forName("com.mysql.jdbc.Driver");
String url = "jdbc:mysql://localhost:3306/test";
String username = "root";
String password = "root";
conn = DriverManager.getConnection(url, username, password);
}
// 关闭数据库方法
private void closeConn() throws Exception {
if (rs != null) {
rs.close();
}
if (ps != null) {
ps.close();
}
if (conn != null) {
conn.close();
}
}
// 查询用户方法
public User getUser(String username, String password) throws Exception {
getConn();
String sql = "select * from user where username=? and password=?";
ps = conn.prepareStatement(sql);
ps.setString(1, username);
ps.setString(2, password);
rs = ps.executeQuery();
User user = null;
while (rs.next()) {
user = new User();
user.setId(rs.getInt("id"));
user.setUsername(rs.getString("username"));
user.setPassword(rs.getString("password"));
}
closeConn();
return user;
}
// 保存用户方法
public void saveUser(User user) throws Exception {
getConn();
String sql = "insert into user (username,password) values(?,?)";
ps = conn.prepareStatement(sql);
ps.setString(1, user.getUsername());
ps.setString(2, user.getPassword());
ps.executeUpdate();
closeConn();
}
}
步骤四:编写Servlet代码
编写一个LoginServlet类,用于实现用户登录的功能,包括输入数据的接收和校验,验证码的生成和校验,以及页面的跳转等,该类的代码如下:
public class LoginServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
// 生成指定长度的随机字符串
private String getRandomString(int length) {
String str = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789";
Random random = new Random();
StringBuffer sb = new StringBuffer();
for (int i = 0; i < length; i++) {
int number = random.nextInt(62);
sb.append(str.charAt(number));
}
return sb.toString();
}
//生成验证码图片
private void outputCaptcha(HttpServletRequest request, HttpServletResponse response) throws IOException {
// 设置响应的类型格式为图片格式
response.setContentType("image/jpeg");
//禁止图像缓存。
response.setHeader("Cache-Control", "no-store");
response.setHeader("Pragma", "no-cache");
response.setDateHeader("Expires", 0);
int width = 80, height = 30;
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
Graphics g = image.getGraphics();
Random random = new Random();
g.setColor(getRandColor(200, 250));
g.fillRect(0, 0, width, height);
g.setFont(new Font("Times New Roman", Font.PLAIN, 18));
g.setColor(getRandColor(160, 200));
for (int i = 0; i < 155; i++) {
int x = random.nextInt(width);
int y = random.nextInt(height);
int xl = random.nextInt(12);
int yl = random.nextInt(12);
g.drawLine(x, y, x + xl, y + yl);
}
String captcha = getRandomString(4);
request.getSession().setAttribute("captcha", captcha);
g.setFont(new Font("Arial", Font.BOLD, 20));
g.setColor(new Color(20 + random.nextInt(110), 20 + random.nextInt(110), 20 + random.nextInt(110)));
g.drawString(captcha, 15, 25);
g.dispose();
ImageIO.write(image, "JPEG", response.getOutputStream());
}
//获取给定范围内的随机颜色
private Color getRandColor(int fc, int bc) {
Random random = new Random();
if (fc > 255)
fc = 255;
if (bc > 255)
bc = 255;
int r = fc + random.nextInt(bc - fc);
int g = fc + random.nextInt(bc - fc);
int b = fc + random.nextInt(bc - fc);
return new Color(r, g, b);
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doPost(request, response);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
String username = request.getParameter("username");
String password = request.getParameter("password");
String captcha = request.getParameter("captcha");
String sessionCaptcha = (String) request.getSession().getAttribute("captcha");
if (captcha == null || !captcha.equals(sessionCaptcha)) {
request.setAttribute("message", "验证码错误");
request.getRequestDispatcher("/login.jsp").forward(request, response);
return;
}
UserDAO dao = new UserDAO();
User user = null;
try {
user = dao.getUser(username, password);
} catch (Exception e) {
e.printStackTrace();
}
if (user == null) {
request.setAttribute("message", "用户名或密码错误");
request.getRequestDispatcher("/login.jsp").forward(request, response);
} else {
request.getRequestDispatcher("/success.jsp").forward(request, response);
}
}
}
步骤五:编写验证码页面代码
编写一个captcha.jsp页面,用于显示验证码图片,该页面的代码如下:
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="java.awt.*,java.awt.image.*"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Captcha</title>
</head>
<body>
<img src="loginServlet?method=outputCaptcha" onclick="this.src='loginServlet?method=outputCaptcha&' + Math.random();" />
</body>
</html>
步骤六:编写登录页面代码
编写一个login.jsp页面,用于输入用户名、密码和验证码,该页面的代码如下:
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Login</title>
</head>
<body>
<form action="loginServlet" method="post">
<label>用户名:</label><input type="text" name="username" /><br />
<label>密 码:</label><input type="password" name="password" /><br />
<label>验证码:</label><input type="text" name="captcha" /><img src="captcha.jsp" /><br />
<input type="submit" value="登录" />
</form>
<% if (request.getAttribute("message") != null) { %>
<p><%= request.getAttribute("message") %></p>
<% } %>
</body>
</html>
步骤七:编写登录成功页面代码
编写一个success.jsp页面,用于显示登录成功的信息,该页面的代码如下:
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Login Success</title>
</head>
<body>
<h1>Login Success</h1>
<p>欢迎您,<%= request.getParameter("username") %>!</p>
</body>
</html>
示例说明
示例一:正确输入用户名、密码和验证码登录
- 打开浏览器,访问登录页面,如:http://localhost:8080/login.jsp
- 输入正确的用户名和密码,输入正确的验证码,点击登录按钮
- 系统提示登录成功,跳转到成功页面,展示登录的用户名信息
示例二:输入错误的验证码
- 打开浏览器,访问登录页面,如:http://localhost:8080/login.jsp
- 输入正确的用户名和密码,输入错误的验证码,点击登录按钮
- 系统提示验证码错误,重新输入正确的验证码,或刷新验证码重新获取
本文标题为:Servlet+JDBC实现登陆功能的小例子(带验证码)
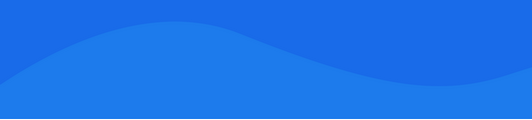
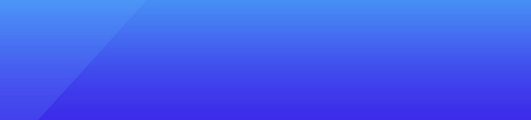
基础教程推荐
- Java聊天室之实现客户端一对一聊天功能 2023-06-30
- 如何在Java中使用正则表达式API 2022-12-16
- 浅谈apache和nginx的rewrite的区别 2023-12-15
- Java编写Mapreduce程序过程浅析 2023-07-15
- Java并发工具类Future使用示例 2022-11-29
- springboot加载一个properties文件转换为map方式 2023-02-27
- URL @PathVariable 变量的匹配原理分析 2024-01-11
- 详解Spring Controller autowired Request变量 2023-08-01
- SpringBoot整合Web开发之Json数据返回的实现 2023-04-12
- Spring AOP实现记录操作日志 2023-05-08