Vue.js 是一款 MVVM 框架,常用来构建单页应用程序(SPA)。在前后端分离的架构下,前端需要向后台发送请求来获取数据。Vue 框架可以通过内置的 axios 库来发送请求和接收响应。下面我们将以一个示例代码的形式演示如何使用 Vue.js 发送请求并处
Vue.js 是一款 MVVM 框架,常用来构建单页应用程序(SPA)。在前后端分离的架构下,前端需要向后台发送请求来获取数据。Vue 框架可以通过内置的 axios 库来发送请求和接收响应。下面我们将以一个示例代码的形式演示如何使用 Vue.js 发送请求并处理响应。
步骤一:安装 axios
在使用 axios 前,需要先通过npm或yarn 安装 axios 库。
npm install axios
#或
yarn add axios
步骤二:引入 axios
在需要使用 axios 的组件中引入 axios 库:
import axios from 'axios'
步骤三:发送请求
通过 axios 发送 GET 请求:
axios.get('/api/data')
.then(function (response) {
console.log(response.data);
})
.catch(function (error) {
console.log(error);
});
通过 axios 发送 POST 请求:
axios.post('/api/data', {name: 'John', age: 30})
.then(function (response) {
console.log(response);
})
.catch(function (error) {
console.log(error);
});
示例一
在实际开发中,经常需要向后台请求数据并将数据渲染到页面中。以下示例演示如何使用 axios 发送请求并在 Vue 组件中渲染数据。
在组件中定义一个 data 属性,用于存储从后台获取到的数据:
export default {
data() {
return {
items: []
}
}
}
在组件的 created 生命周期函数中发送 GET 请求,并将响应中的数据存储在 items 数组中:
export default {
data() {
return {
items: []
}
},
created() {
axios.get('/api/items')
.then((response) => {
this.items = response.data
})
.catch((error) => {
console.log(error)
})
}
}
在模板中渲染数据:
<ul>
<li v-for="item in items" :key="item.id">{{ item.name }}</li>
</ul>
示例二
在实际开发中,经常需要向后台发送数据并根据响应结果执行相应操作。以下示例演示如何使用 axios 发送请求并根据响应结果执行相应操作。
在按钮上添加点击事件,当用户点击按钮时,触发发送 POST 请求的函数:
<template>
<div>
<button @click="addToCart">Add to Cart</button>
</div>
</template>
在组件 methods 中定义发送 POST 请求的函数,并根据响应结果执行相应操作:
export default {
data() {
return {
success: false
}
},
methods: {
addToCart() {
axios.post('/api/cart', {productId: 1})
.then((response) => {
if (response.data.success === true) {
this.success = true
} else {
this.success = false
}
})
.catch((error) => {
console.log(error)
})
}
}
}
在模板中显示操作结果:
<template>
<div>
<button @click="addToCart">Add to Cart</button>
<p v-if="success">Successfully added to cart.</p>
<p v-else>Failed to add to cart.</p>
</div>
</template>
以上就是 Vue.js 请求后台数据的实例代码的完整攻略,希望能对你有所帮助。
本文标题为:vue 请求后台数据的实例代码
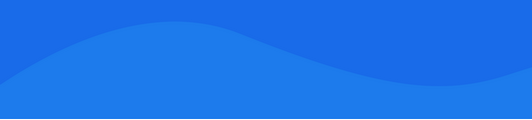
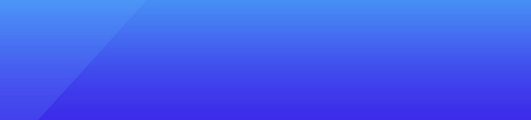
基础教程推荐
- 如何在MySQL数据库中建模保存自身实例的Java对象? 2023-11-06
- springboot swagger不显示接口的问题及解决 2023-01-24
- jsp Response对象页面重定向、时间的动态显示 2023-07-30
- Spring6整合JUnit的详细步骤 2023-07-14
- Spring Data JPA系列QueryByExampleExecutor使用详解 2023-06-02
- Java实现窗体程序显示日历表 2023-01-02
- 详解Java Bellman-Ford算法原理及实现 2023-02-27
- Java树形结构查询用法介绍 2023-10-08
- java开发RocketMQ生产者高可用示例详解 2023-04-06
- Spring在web.xml中的配置详细介绍 2023-07-31