下面是关于Swing登录注册界面设计的详细攻略。
下面是关于"Swing登录注册界面设计"的详细攻略。
1. Swing介绍
Swing是Java的一个GUI工具包,可以使用Swing创建出各种类型的GUI应用程序,包括桌面应用程序,游戏应用程序等。Swing提供了许多控件如文本框(Text Field)、标签(Label)、按钮(Button)、下拉框(Combo Box)、表格(Table)、列表(List)等等。Swing提供强大的定制及扩展能力,可以使用Swing定制自己风格的控件,并实现复杂的图形界面。
2. 登录注册界面的设计过程
2.1 确定需求
首先我们需要明确我们的需求,我们需要实现什么样的登录注册功能,具体需要哪些控件,需要哪些功能。在这里,我们打算实现一个简单的用户登录注册系统,需要使用到用户名、密码、邮箱等控件,需要实现用户的登录、注册、修改密码等功能。
2.2 设计界面
接下来,我们需要设计我们的登录注册界面。我们可以使用Swing提供的控件,如JLabel、JTextField、JPasswordField、JButton等,同时也可以使用布局管理器来布局控件,如FlowLayout、GridLayout、BorderLayout等。
下面是一个简单的登录界面设计示例:
import javax.swing.*;
import java.awt.*;
public class LoginFrame extends JFrame {
private JLabel userLabel, passwordLabel;
private JTextField userText;
private JPasswordField passwordText;
private JButton loginButton, registerButton;
private void init() {
setTitle("登录");
setSize(300, 180);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 用户名和密码输入框
userLabel = new JLabel("用户名:");
passwordLabel = new JLabel("密码:");
userText = new JTextField(20);
passwordText = new JPasswordField(20);
// 登录和注册按钮
loginButton = new JButton("登录");
registerButton = new JButton("注册");
// 创建面板,添加控件
JPanel inputPanel = new JPanel();
inputPanel.setLayout(new GridLayout(2, 2));
inputPanel.add(userLabel);
inputPanel.add(userText);
inputPanel.add(passwordLabel);
inputPanel.add(passwordText);
JPanel buttonPanel = new JPanel();
buttonPanel.setLayout(new FlowLayout());
buttonPanel.add(loginButton);
buttonPanel.add(registerButton);
// 创建主面板,添加子面板
JPanel mainPanel = new JPanel();
mainPanel.setLayout(new BorderLayout());
mainPanel.add(inputPanel, BorderLayout.CENTER);
mainPanel.add(buttonPanel, BorderLayout.SOUTH);
// 添加主面板到窗口
getContentPane().add(mainPanel);
}
public LoginFrame() {
init();
}
public static void main(String[] args) {
LoginFrame frame = new LoginFrame();
frame.setVisible(true);
}
}
2.3 实现功能
接下来,我们需要实现我们的功能,包括用户登录、注册、修改密码等功能。我们可以使用Swing提供的事件处理机制,如ActionListener、MouseListener等来处理我们的事件。同时,我们也可以使用JOptionPane来显示提示信息或对话框。
下面是一个简单的登录实现示例:
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class LoginFrame extends JFrame implements ActionListener {
private JLabel userLabel, passwordLabel;
private JTextField userText;
private JPasswordField passwordText;
private JButton loginButton, registerButton;
private void init() {
setTitle("登录");
setSize(300, 180);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 用户名和密码输入框
userLabel = new JLabel("用户名:");
passwordLabel = new JLabel("密码:");
userText = new JTextField(20);
passwordText = new JPasswordField(20);
// 登录和注册按钮
loginButton = new JButton("登录");
registerButton = new JButton("注册");
// 添加事件监听器
loginButton.addActionListener(this);
registerButton.addActionListener(this);
// 创建面板,添加控件
JPanel inputPanel = new JPanel();
inputPanel.setLayout(new GridLayout(2, 2));
inputPanel.add(userLabel);
inputPanel.add(userText);
inputPanel.add(passwordLabel);
inputPanel.add(passwordText);
JPanel buttonPanel = new JPanel();
buttonPanel.setLayout(new FlowLayout());
buttonPanel.add(loginButton);
buttonPanel.add(registerButton);
// 创建主面板,添加子面板
JPanel mainPanel = new JPanel();
mainPanel.setLayout(new BorderLayout());
mainPanel.add(inputPanel, BorderLayout.CENTER);
mainPanel.add(buttonPanel, BorderLayout.SOUTH);
// 添加主面板到窗口
getContentPane().add(mainPanel);
}
public LoginFrame() {
init();
setLocationRelativeTo(null);
}
/**
* 处理按钮事件
*/
@Override
public void actionPerformed(ActionEvent e) {
// 登录按钮
if (e.getSource() == loginButton) {
String username = userText.getText();
String password = new String(passwordText.getPassword());
// TODO:实现登录功能
if (username.equals("admin") && password.equals("admin")) {
JOptionPane.showMessageDialog(this, "登录成功!");
} else {
JOptionPane.showMessageDialog(this, "用户名或密码不正确!");
}
}
// 注册按钮
if (e.getSource() == registerButton) {
// TODO:实现注册功能
JOptionPane.showMessageDialog(this, "注册功能暂未实现!");
}
}
public static void main(String[] args) {
LoginFrame frame = new LoginFrame();
frame.setVisible(true);
}
}
3. 总结
以上就是关于"Swing登录注册界面设计"的详细攻略,通过这次学习,我们能够设计出各种类型的GUI应用程序,并实现各项功能。当然,我们的设计和实现还有很多需要改进的地方,例如界面的美观性、代码的可维护性等等。相信在不断的学习和实践中,我们会变得越来越优秀!
本文标题为:swing登录注册界面设计
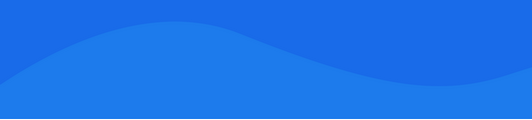
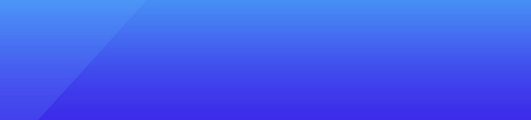
基础教程推荐
- 详解Java8中Optional的常见用法 2023-05-14
- Spring MVC学习笔记之json格式的输入和输出 2024-02-25
- java – 用数据库设置应用程序 – tapestry-hibernate失败 2023-11-09
- spring boot项目使用@Async注解的坑 2023-03-07
- Java实现获取Excel中的表单控件 2022-11-16
- SpringBoot请求参数相关注解说明小结 2022-11-08
- MyEclipse中jsp的注释报错解决方法 2023-12-15
- SpringBoot自定义转换器应用实例讲解 2023-04-17
- Gateway网关源码解析 2023-03-22
- jsp页面验证码完整实例 2023-07-30