下面我会详细讲解“Android开发中的文件操作工具类FileUtil完整实例”的攻略,包含以下几个方面的内容:
下面我会详细讲解“Android开发中的文件操作工具类FileUtil完整实例”的攻略,包含以下几个方面的内容:
- 简介
- 文件读取
- 文件写入
- 文件复制
- 文件删除
- 示例说明
- 结论
1. 简介
在Android开发中,文件操作时常遇到,因此可以写一个工具类封装常用的文件操作,方便进行文件操作。
2. 文件读取
使用FileInputStream对象打开文件,然后使用BufferedReader对象读取数据。代码示例如下:
public static String readFromFile(String filePath) {
StringBuilder sb = new StringBuilder();
try {
FileInputStream inputStream = new FileInputStream(filePath);
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(inputStream, "UTF-8"));
String line;
while ((line = bufferedReader.readLine()) != null) {
sb.append(line);
}
inputStream.close();
bufferedReader.close();
} catch (IOException e) {
e.printStackTrace();
}
return sb.toString();
}
3. 文件写入
使用FileOutputStream对象打开文件,然后使用BufferedWriter对象写入数据。代码示例如下:
public static void writeToFile(String filePath, String content) {
try {
FileOutputStream outputStream = new FileOutputStream(filePath, false);
OutputStreamWriter outputStreamWriter = new OutputStreamWriter(outputStream, "UTF-8");
BufferedWriter bufferedWriter = new BufferedWriter(outputStreamWriter);
bufferedWriter.write(content);
bufferedWriter.flush();
bufferedWriter.close();
outputStreamWriter.close();
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
4. 文件复制
使用FileInputStream和FileOutputStream对象打开文件,然后使用BufferedInputStream和BufferedOutputStream对象进行文件复制。代码示例如下:
public static void copyFile(String sourceFilePath, String targetFilePath) {
try {
FileInputStream inputStream = new FileInputStream(sourceFilePath);
BufferedInputStream bufferedInputStream = new BufferedInputStream(inputStream);
FileOutputStream outputStream = new FileOutputStream(targetFilePath);
BufferedOutputStream bufferedOutputStream = new BufferedOutputStream(outputStream);
byte[] buffer = new byte[1024];
int length;
while ((length = bufferedInputStream.read(buffer)) != -1) {
bufferedOutputStream.write(buffer, 0, length);
}
bufferedOutputStream.flush();
bufferedInputStream.close();
inputStream.close();
bufferedOutputStream.close();
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
5. 文件删除
使用File对象的delete()方法删除文件,代码示例如下:
public static void deleteFile(String filePath) {
File file = new File(filePath);
if (file.exists()) {
file.delete();
}
}
6. 示例说明
以下是使用FileUtil工具类读写文件的示例代码:
String fileName = "test.txt";
String content = "Hello, world!";
FileUtil.writeToFile(fileName, content);
String result = FileUtil.readFromFile(fileName);
Log.d(TAG, result);
以下是使用FileUtil工具类复制、删除文件的示例代码:
String sourceFilePath = "source.txt";
String targetFilePath = "target.txt";
FileUtil.copyFile(sourceFilePath, targetFilePath);
FileUtil.deleteFile(sourceFilePath);
FileUtil.deleteFile(targetFilePath);
7. 结论
本文以文件读取、文件写入、文件复制和文件删除为例,详细讲解了Android开发中的文件操作工具类FileUtil的使用。使用该工具类可以方便地进行文件操作,提高开发效率。
本文标题为:Android开发中的文件操作工具类FileUtil完整实例
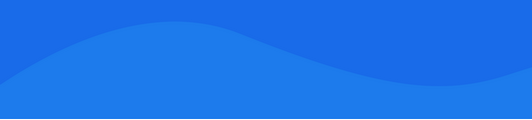
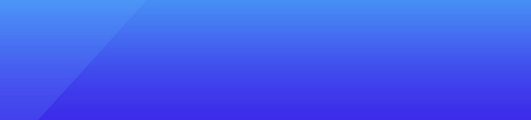
基础教程推荐
- JSP+EXt2.0实现分页的方法 2024-01-10
- jsp中定义和使用方法示例介绍 2023-12-16
- SpringBoot详解整合Spring Boot Admin实现监控功能 2023-02-19
- JSP由浅入深(5)—— Scriptlets和HTML的混合 2024-01-13
- Spring Cloud Alibaba使用Nacos作为注册中心和配置中心 2023-01-08
- Java zookeeper服务的使用详解 2023-03-22
- jsp用过滤器解决中文乱码问题的方法 2023-08-01
- Spring底层原理深入分析 2023-02-28
- 微信小程序获取手机号的完整实例(Java后台实现) 2023-01-09
- 再有人问你Java内存模型是什么,就把这篇文章发给他 2023-09-01