在SpringMVC中,我们可以使用注解@ResponseBody将一个Java对象转换为JSON格式的字符串进行传输,但是在某些场景下,我们可能需要对Java对象进行自定义的序列化和反序列化操作,以满足特定的需求。
SpringMVC Json自定义序列化和反序列化的操作方法
在SpringMVC中,我们可以使用注解@ResponseBody将一个Java对象转换为JSON格式的字符串进行传输,但是在某些场景下,我们可能需要对Java对象进行自定义的序列化和反序列化操作,以满足特定的需求。
自定义序列化
自定义序列化的实现,一般通过实现Spring提供的JsonSerializer
接口来完成。下面给出一个示例,演示如何实现对Java中Date类型的格式化输出:
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Date;
import com.fasterxml.jackson.core.JsonGenerator;
import com.fasterxml.jackson.databind.JsonSerializer;
import com.fasterxml.jackson.databind.SerializerProvider;
public class JsonDateSerializer extends JsonSerializer<Date>{
@Override
public void serialize(Date date, JsonGenerator jsonGenerator, SerializerProvider provider) throws IOException {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
jsonGenerator.writeString(sdf.format(date));
}
}
上面的示例中,我们定义了一个JsonDateSerializer
类,实现了Jackson提供的JsonSerializer
接口,覆盖其中的serialize()
方法。在该实现中,我们使用SimpleDateFormat将Date类型的值序列化为 yyyy-MM-dd 格式的字符串后,写入JsonGenerator中。
接下来,我们需要将自定义实现的JsonDateSerializer
注册到Jackson的ObjectMapper中,让其在执行序列化操作时被优先调用。可以在Spring的配置文件中添加以下配置:
<mvc:annotation-driven>
<mvc:message-converters>
<bean class="org.springframework.http.converter.json.MappingJackson2HttpMessageConverter">
<property name="objectMapper">
<bean class="com.fasterxml.jackson.databind.ObjectMapper">
<property name="dateFormat">
<bean class="java.text.SimpleDateFormat">
<constructor-arg value="yyyy-MM-dd HH:mm:ss">
</constructor-arg>
</bean>
</property>
<property name="serializers">
<array>
<bean class="com.example.JsonDateSerializer" />
</array>
</property>
</bean>
</property>
</bean>
</mvc:message-converters>
</mvc:annotation-driven>
在以上配置中,我们首先配置了一个Jackson的ObjectMapper对象,其中包含了格式化日期的SimpleDateFormat实例和自定义的JsonSerializer实例,使其被注册到Jackson中。
自定义反序列化
自定义反序列化的实现,一般也是通过实现Spring提供的JsonDeserializer
接口来完成。下面给出一个示例,演示如何实现对Json中自定义字段的解析:
import java.io.IOException;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.DeserializationContext;
import com.fasterxml.jackson.databind.JsonDeserializer;
import com.fasterxml.jackson.databind.JsonNode;
public class CustomObjectDeserializer extends JsonDeserializer<CustomObject> {
@Override
public CustomObject deserialize(JsonParser jsonParser, DeserializationContext context)
throws IOException, JsonProcessingException {
JsonNode node = jsonParser.getCodec().readTree(jsonParser);
String value1 = node.get("customValue1").asText();
String value2 = node.get("customValue2").asText();
return new CustomObject(value1, value2);
}
}
在上面的示例中,我们定义了一个CustomObjectDeserializer
类,实现了Jackson提供的JsonDeserializer
接口,覆盖其中的deserialize()
方法。在该方法中,我们通过JsonParser读取Json字符串,获得自定义字段customValue1和customValue2的值,并构造一个新的CustomObject实例进行返回。
接下来,我们也需要将自定义实现的CustomObjectDeserializer
注册到Jackson的ObjectMapper中,使其在执行反序列化操作时被优先调用。可以按照以下方式进行配置:
<bean class="com.fasterxml.jackson.databind.ObjectMapper">
<property name="deserializers">
<map>
<entry key="[Lcom.example.CustomObject;">
<bean class="com.example.CustomObjectDeserializer"/>
</entry>
</map>
</property>
</bean>
在以上配置中,我们将自定义的CustomObjectDeserializer
实例注册到Jackson的ObjectMapper中,当Json字符串中包含CustomObject类型的数据时,将优先调用该实例完成反序列化操作。
示例说明
示例1:自定义序列化
假设有一个User类,其中包含了一个Date类型的字段birthday,我们希望在序列化过程中,将其以"yyyy-MM-dd"格式的字符串输出。我们可以按照以下方式,在User类中使用自定义的JsonDateSerializer实现:
public class User {
private String userName;
private Date birthday;
//getter和setter方法省略
@JsonSerialize(using = JsonDateSerializer.class)
public Date getBirthday() {
return birthday;
}
}
在以上示例中,我们为User类的birthday字段添加了@JsonSerialize
注解,指定其使用我们自定义的JsonDateSerializer
实例进行序列化操作。
示例2:自定义反序列化
假设有一个CustomObject类:
public class CustomObject {
private String customValue1;
private String customValue2;
//getter和setter方法省略
}
我们希望在反序列化Json字符串时,将其中的特定字段customValue1和customValue2解析出来,并构造一个新的CustomObject实例返回。我们可以按照以下方式,在CustomObject类中使用自定义的CustomObjectDeserializer实现:
public class CustomObject {
private String customValue1;
private String customValue2;
//getter和setter方法省略
@JsonDeserialize(using = CustomObjectDeserializer.class)
public void setValue(String jsonStr) {
//...
}
}
在以上示例中,我们为CustomObject类的setValue方法添加了@JsonDeserialize
注解,指定其使用我们自定义的CustomObjectDeserializer
实例进行反序列化操作。
本文标题为:SpringMVC Json自定义序列化和反序列化的操作方法
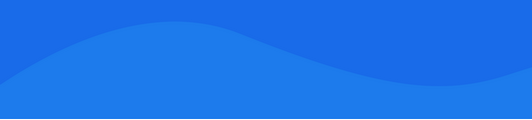
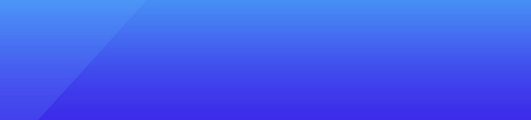
基础教程推荐
- Java postgresql数组字段类型处理方法详解 2023-06-16
- maven依赖传递和依赖冲突原理 2023-07-15
- Java多线程并发与并行和线程与进程案例 2023-01-08
- JSP开发中hibernate框架的常用检索方式总结 2023-08-02
- 源码解析springbatch的job运行机制 2023-04-07
- Mysql中备份表的多种方法 2023-07-01
- Http请求长时间等待无结果返回解决办法 2023-07-31
- java – Hibernate并发插入(MySQL INSERT IGNORE) 2023-11-03
- ShardedJedisPool的使用package com.test; import java.util.ArrayList; import java.util.List; import redis 2023-11-04
- MySQL MyBatis 默认插入当前时间方式 2023-06-10