一、springmvc图片上传过程详解
一、springmvc图片上传过程详解
1.在pom.xml文件中添加以下依赖
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.4</version>
</dependency>
2.在springmvc.xml中添加以下配置
<!--文件上传配置-->
<bean id="multipartResolver"
class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<property name="defaultEncoding" value="UTF-8"/>
<property name="maxUploadSize" value="2097152"/>
<property name="maxInMemorySize" value="1048576"/>
<!--默认上传临时文件目录-->
<property name="uploadTempDir">
<bean class="org.springframework.web.multipart.commons.CommonsMultipartFile"
factory-bean="fileItemFactory"
factory-method="getUploadDir"/>
</property>
</bean>
<!--文件上传工厂类-->
<bean id="fileItemFactory" class="org.apache.commons.fileupload.disk.DiskFileItemFactory">
<property name="repository"
value="D:/temp/upload"/>
<!--上传文件大于10KB时生成新的临时文件-->
<property name="sizeThreshold"
value="10240"/>
</bean>
3.在controller中添加以下代码
@RequestMapping("/upload")
public String upload(@RequestParam("file") MultipartFile file,
HttpServletRequest request) {
//检查文件是否为空
if (!file.isEmpty()) {
try {
//保存文件
String filename = file.getOriginalFilename();
String path = request.getSession().getServletContext()
.getRealPath("upload/" + filename);
file.transferTo(new File(path));
return "上传成功";
} catch (Exception e) {
e.printStackTrace();
return "上传失败";
}
} else {
return "文件为空";
}
}
二、json数据转换过程详解
1.在pom.xml文件中添加以下依赖
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.13.0</version>
</dependency>
2.在controller中添加以下代码
@RequestMapping("/json")
@ResponseBody
public Map<String, Object> json() {
Map<String, Object> map = new HashMap<>(); map.put("name", "Tom");
map.put("age", 18);
return map;
}
3.访问json接口,可以看到返回的数据格式为json
{
"name": "Tom",
"age": 18
}
示例1:springmvc图片上传
1.上传文件的html代码段
<form method="POST" action="/upload" enctype="multipart/form-data">
<input type="file" name="file"/>
<button type="submit">上传</button>
</form>
2.上传文件的controller
@RequestMapping("/upload")
@ResponseBody
public String upload(@RequestParam("file") MultipartFile file,
HttpServletRequest request) {
//检查文件是否为空
if (!file.isEmpty()) {
try {
//保存文件
String filename = file.getOriginalFilename();
String path = request.getSession().getServletContext()
.getRealPath("upload/" + filename);
file.transferTo(new File(path));
return "上传成功";
} catch (Exception e) {
e.printStackTrace();
return "上传失败";
}
} else {
return "文件为空";
}
}
示例2:json数据转换
1.获取json数据的html代码段
<button type="button" onclick="getJson()">获取Json数据</button>
<script>
function getJson() {
$.ajax({
url: "/json",
type: "GET",
dataType: "json",
success: function (data) {
alert(JSON.stringify(data))
},
error: function () {
alert("获取数据失败")
}
})
}
</script>
2.获取json数据的controller
@RequestMapping("/json")
@ResponseBody
public Map<String, Object> json() {
Map<String, Object> map = new HashMap<>();
map.put("name", "Tom");
map.put("age", 18);
return map;
}
沃梦达教程
本文标题为:springmvc图片上传及json数据转换过程详解
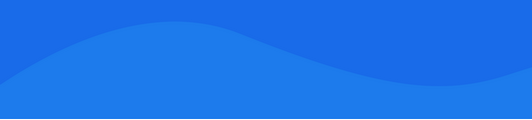
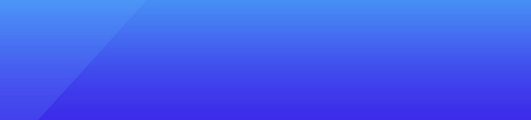
基础教程推荐
猜你喜欢
- Netty序列化深入理解与使用 2023-03-22
- 深入解读MVC模式和三层架构 2024-02-26
- 是否有适用于ARM Linux的java sqlite3库? 2023-11-05
- postman 如何实现传递 ArrayList 给后台 2023-08-10
- java – Microsoft SQL JDBC驱动程序v6.2为DATETIME字段返回不正确的SQL类型代码 2023-11-07
- java – 如何以编程方式将数据库中的数据导出为.csv格式? 2023-11-08
- java中断线程的正确姿势完整示例 2023-07-15
- java 如何在list中删除我指定的对象 2023-08-11
- 关于Feign的覆写默认配置和Feign的日志 2023-01-12
- Mybatis条件if test如何使用枚举值 2022-12-07