下面是SpringBoot使用Fastjson解析Json数据的攻略,包含以下几个部分:
下面是SpringBoot使用Fastjson解析Json数据的攻略,包含以下几个部分:
- 添加Fastjson的依赖
- 编写用于解析Json数据的代码
- 示例
添加Fastjson的依赖
首先需要在项目的pom.xml文件中添加Fastjson的依赖,可以在官方网站中查看最新版本并添加如下代码:
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.58</version>
</dependency>
编写用于解析Json数据的代码
在SpringBoot中,可以在Controller层中编写用于解析Json数据的代码。首先需要引入Fastjson的相关包,在方法中调用Fastjson的API来解析Json数据。
以下是一个简单的例子:
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ExampleController {
@PostMapping("/example")
public void handleJsonData(@RequestBody String jsonStr) {
JSONObject jsonObject = JSON.parseObject(jsonStr);
String name = jsonObject.getString("name");
int age = jsonObject.getInteger("age");
System.out.println("name:" + name + ", age:" + age);
}
}
在上面的代码中,我们使用JSON.parseObject
方法将接收到的json字符串转换为JSONObject
对象,然后通过getString
和getInteger
方法来获取其中的属性值。
示例
下面举一个更完整的例子,在这个例子中,我们需要解析以下Json数据:
{
"code": 200,
"msg": "请求成功",
"data": {
"id": 123,
"name": "Tom",
"gender": "male",
"scores": [88, 92, 95, 90]
}
}
我们可以通过以下方式进行解析:
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ExampleController {
@PostMapping("/example")
public void handleJsonData(@RequestBody String jsonStr) {
JSONObject jsonObject = JSON.parseObject(jsonStr);
int code = jsonObject.getInteger("code");
String msg = jsonObject.getString("msg");
JSONObject data = jsonObject.getJSONObject("data");
int id = data.getInteger("id");
String name = data.getString("name");
String gender = data.getString("gender");
JSONArray scores = data.getJSONArray("scores");
for (int i = 0; i < scores.size(); i++) {
int score = scores.getInteger(i);
System.out.println("score:" + score);
}
}
}
在上面的代码中,我们首先使用JSON.parseObject
方法将接收到的Json字符串转换为JSONObject
对象,然后通过getInteger
、getString
和getJSONArray
等方法分别获取其中的属性值,并且可以通过JSONArray.size
方法来获取数组的大小。
本文标题为:SpringBoot如何使用Fastjson解析Json数据
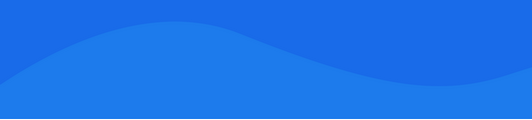
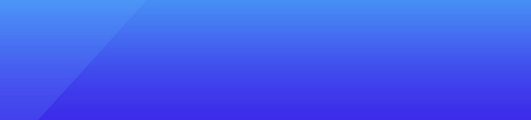
基础教程推荐
- 一文搞懂Spring循环依赖的原理 2023-02-27
- Java Spring框架创建项目与Bean的存储与读取详解 2023-03-21
- Java实现替换Word中文本和图片功能 2022-11-29
- Java Swing实现画板的简单操作 2022-12-16
- Java线程池详细解读 2024-03-08
- JAVA使用commos-fileupload实现文件上传与下载实例解析 2024-02-28
- 一个JSP页面导致的tomcat内存溢出的解决方法 2023-12-17
- java – 我是否需要管理有关数据库访问的并发性? 2023-11-08
- Java / MySQL – 日期时间问题 2023-11-05
- spring boot教程之IDEA环境下的热加载与热部署 2023-05-08