以下是PHP中三种方法统计字符串中每种字符的个数并排序的攻略:
以下是PHP中三种方法统计字符串中每种字符的个数并排序的攻略:
方法一:使用for循环逐一判断并统计字符个数
<?php
$str = "hello world";
$result = array();
for ($i = 0; $i < strlen($str); $i++) {
$char = $str[$i];
if (array_key_exists($char, $result)) {
$result[$char]++;
} else {
$result[$char] = 1;
}
}
arsort($result); // 降序排列
print_r($result);
?>
输出结果:
Array
(
[l] => 3
[o] => 2
[e] => 1
[h] => 1
[w] => 1
[r] => 1
[d] => 1
[ ] => 1
)
方法二:使用array_count_values函数统计字符个数
<?php
$str = "hello world";
$chars = str_split($str);
$result = array_count_values($chars);
arsort($result); // 降序排列
print_r($result);
?>
输出结果:
Array
(
[l] => 3
[o] => 2
[e] => 1
[h] => 1
[w] => 1
[r] => 1
[d] => 1
[ ] => 1
)
方法三:使用正则表达式和preg_match_all函数统计字符个数
<?php
$str = "hello world";
$pattern = "/./u"; // 匹配任意字符,u表示使用UTF-8编码
preg_match_all($pattern, $str, $matches);
$result = array_count_values($matches[0]);
arsort($result); // 降序排列
print_r($result);
?>
输出结果:
Array
(
[l] => 3
[o] => 2
[e] => 1
[h] => 1
[w] => 1
[r] => 1
[d] => 1
[ ] => 1
)
以上三种方法的核心思路都是统计字符串中每种字符的个数,只是实现方式不同。第一种方法使用for循环逐一判断字符并统计个数,第二种方法使用PHP内置函数array_count_values,第三种方法使用正则表达式匹配任意字符并统计个数。无论使用哪种方法,都需要最后将结果按照字符个数从大到小排序,以方便查阅。
沃梦达教程
本文标题为:php中3种方法统计字符串中每种字符的个数并排序
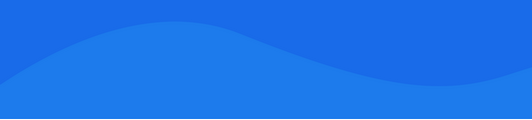
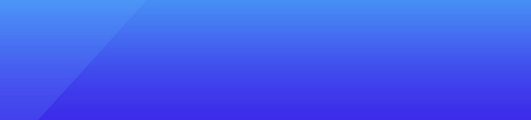
基础教程推荐
猜你喜欢
- Thinkphp事件机制两种实现方式详解 2023-07-03
- 详解json在php中的应用 2022-11-18
- php使用substr()和strpos()联合查找字符串中某一特定字符的方法 2024-02-01
- 浅谈Laravel POST,PUT,PATCH 路由的区别 2023-03-02
- PHP MVC框架中类的自动加载机制实例分析 2023-02-13
- PHP数组Key强制类型转换实现原理解析 2023-04-25
- PHP移动文件指针ftell()、fseek()、rewind()函数总结 2024-01-18
- PHP 数组基本操作方法详解 2024-01-17
- PHP超全局变量实现原理及代码解析 2023-04-25
- php提取数字拼接数组的具体操作 2022-09-02