获取元素位置和尺寸信息是web开发中经常需要面对的问题。下面是一些原生JS获取元素位置和尺寸的方法。在示例中,我们将使用一个html文档和一个div元素作为示例。
获取元素位置和尺寸信息是web开发中经常需要面对的问题。下面是一些原生JS获取元素位置和尺寸的方法。在示例中,我们将使用一个html文档和一个div元素作为示例。
获取元素位置
offsetTop和offsetLeft属性
在HTML文档中,每个元素都有offsetTop和offsetLeft属性,它们表示元素相对于其offsetParent(父元素)的顶部和左侧偏移量。
const div = document.getElementById('myDiv');
const top= div.offsetTop; // 获取div相对于其offsetParent的上偏移量
const left = div.offsetLeft; // 获取div相对于其offsetParent的左偏移量
getBoundingClientRect()方法
getBoundingClientRect()方法返回一个具有四个属性(left、top、right、bottom)的DOMRect对象,它表示元素的位置和大小。其中left和top属性表示元素相对于文档左上角(viewport)的位置。
const div = document.getElementById('myDiv');
const rect = div.getBoundingClientRect();
const top= rect.top; // 获取div相对于文档左上角的上偏移量
const left = rect.left; // 获取div相对于文档左上角的左偏移量
获取元素尺寸
offsetWidth和offsetHeight属性
在HTML文档中,每个元素都有offsetWidth和offsetHeight属性,它们表示元素的宽度和高度(包括元素的边框和滚动条)。
const div = document.getElementById('myDiv');
const width = div.offsetWidth; // 获取div的宽度
const height = div.offsetHeight; // 获取div的高度
scrollWidth和scrollHeight属性
scrollWidth和scrollHeight属性返回元素内容的宽度和高度,它们不包括元素的边框和滚动条。如果元素内容未超过可见区域,则scrollWidth和scrollHeight与offsetWidth和offsetHeight相同。
const div = document.getElementById('myDiv');
const width = div.scrollWidth; // 获取div内容的宽度
const height = div.scrollHeight; // 获取div内容的高度
示例说明
示例1:获取div元素的位置信息和尺寸信息,并在控制台输出
<div id="myDiv" style="width: 100px; height: 100px; position: absolute; top: 50px; left: 50px; border: 1px solid black;">
myDiv
</div>
<button onclick="getPositionAndSize()">获取元素位置和尺寸</button>
<script>
function getPositionAndSize() {
const div = document.getElementById('myDiv');
// 获取位置信息
const top = div.offsetTop;
const left = div.offsetLeft;
const rect = div.getBoundingClientRect();
const viewTop = rect.top;
const viewLeft = rect.left;
// 获取尺寸信息
const width = div.offsetWidth;
const height = div.offsetHeight;
const scrollWidth = div.scrollWidth;
const scrollHeight = div.scrollHeight;
console.log("Position: top: " + top + "px, left: " + left + "px, viewTop: " + viewTop + "px, viewLeft: " + viewLeft + "px");
console.log("Size: width: " + width + "px, height: " + height + "px, scrollWidth: " + scrollWidth + "px, scrollHeight: " + scrollHeight + "px");
}
</script>
点击按钮后,将会在控制台输出div元素的位置信息和尺寸信息。
示例2:使用getBoundingClientRect()方法获取点击位置相对于文档左上角的位置信息
<div id="wrapper" style="width: 300px; height: 300px; position: absolute; top: 100px; left: 100px; border: 1px solid black;">
<p style="font-size: 16px; line-height: 24px; margin: 0;">Click anywhere in the wrapper to get the position.</p>
</div>
<script>
const wrapper = document.getElementById('wrapper');
wrapper.addEventListener('click', function (event) {
const rect = wrapper.getBoundingClientRect();
const x = event.clientX - rect.left;
const y = event.clientY - rect.top;
console.log("Clicked at position x: " + x + "px, y: " + y + "px.");
});
</script>
当点击wrapper元素中的任意位置时,都会在控制台输出点击位置相对于文档左上角 的位置信息。
本文标题为:原生JS获取元素的位置与尺寸实现方法
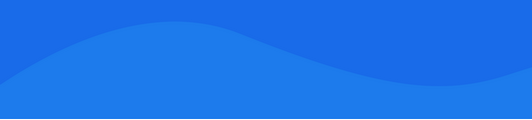
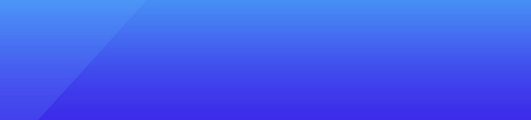
基础教程推荐
- layui表格内可编辑下拉框的实现 2023-11-30
- js关于getImageData跨域问题的解决方法 2023-12-01
- 实例代码讲解ajax实现的无刷新分页 2022-12-15
- 使用postcss-plugin-px2rem和postcss-pxtorem(postcss-px2rem)-px自动转换rem的配置方法-vue-cli3.0 2023-10-08
- 关于 asp.net mvc:如何使用 Razor 语法在 Ext.NET 中指 2022-09-15
- 关于前端ajax请求的优雅方案(http客户端为axios) 2023-02-15
- AJAX实现数据的增删改查操作详解【java后台】 2023-02-23
- Ajax的jsonp方式跨域获取数据的简单实例 2022-12-28
- 浅谈Ajax和JavaScript的区别 2023-01-20
- vue项目结构分析 2023-10-08