JavaScript 可以通过操作 DOM 来实现对 HTML 和 CSS 的操作。下面总结了一些常见的 JavaScript 操作 CSS 的方法。
JavaScript CSS 操作方法集合
概述
JavaScript 可以通过操作 DOM 来实现对 HTML 和 CSS 的操作。下面总结了一些常见的 JavaScript 操作 CSS 的方法。
1. 获取/设置 CSS 样式
获取元素的 CSS 样式
可以通过 getComputedStyle
方法来获取一个元素的样式:
const element = document.getElementById("example");
const style = window.getComputedStyle(element);
console.log(style.backgroundColor); // 等同于 console.log(element.style.backgroundColor);
设置元素的 CSS 样式
可以通过 style
属性的赋值方式实现:
const element = document.getElementById("example");
element.style.backgroundColor = "red";
2. 类名、样式名
添加/删除类名
我们可以通过 classList
对象来操作元素的类名:
const element = document.getElementById("example");
element.classList.add("active");
element.classList.remove("active");
element.classList.toggle("active"); // 如果存在类名 active ,则删除,否则添加
读取/设置样式名
元素的样式名可以通过 className
属性来读取和设置:
const element = document.getElementById("example");
console.log(element.className);
element.className = "test";
3. 尺寸、位置
获取/设置尺寸
可以通过 clientWidth
、clientHeight
、offsetWidth
、offsetHeight
等属性来获取元素的宽度、高度等信息。
const element = document.getElementById("example");
console.log(element.clientWidth);
console.log(element.clientHeight);
console.log(element.offsetWidth);
console.log(element.offsetHeight);
可以通过 style
来设置元素的样式从而改变元素尺寸:
const element = document.getElementById("example");
element.style.width = "200px";
element.style.height = "100px";
获取/设置位置
可以通过 offsetLeft
和 offsetTop
来获取元素相对于定位父元素的位置。
const element = document.getElementById("example");
console.log(element.offsetLeft);
console.log(element.offsetTop);
示例
示例一
下面的例子可以实现鼠标悬浮元素时,改变背景颜色:
HTML:
<div id="example">悬浮我试一试</div>
JavaScript:
const element = document.getElementById("example");
element.addEventListener("mouseover", function() {
this.style.backgroundColor = "red";
});
element.addEventListener("mouseout", function() {
this.style.backgroundColor = "";
});
示例二
下面的例子可以实现滚动到页面底部时,显示一个“返回顶部”的按钮:
HTML:
<button id="back-to-top">返回顶部</button>
JavaScript:
const button = document.getElementById("back-to-top");
window.addEventListener("scroll", function() {
if (window.scrollY > 1000) {
button.style.display = "block";
} else {
button.style.display = "none";
}
});
button.addEventListener("click", function() {
window.scrollTo(0, 0);
});
总结
本篇介绍了常见的操作 CSS 的方法,包括获取/设置 CSS 样式、类名样式名、尺寸和位置。同时也提供了两个示例作为参考。这些方法在实际开发中非常常用,希望读者们可以熟练掌握。
本文标题为:js CSS操作方法集合
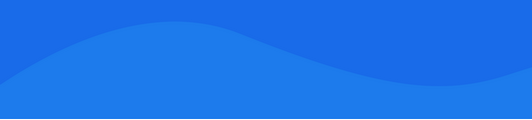
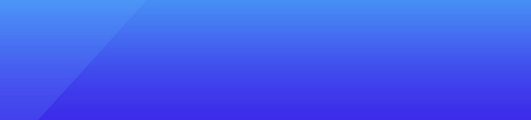
基础教程推荐
- javascript的onchange事件与jQuery的change()方法比较 2023-12-01
- JavaScript股票的动态买卖规划实例分析下篇 2022-10-22
- 23--html(css基础选择器3-id选择器) 2023-10-28
- 解决浏览器记住ajax请求并能前进和后退问题 2023-02-14
- python+selenium 定位到元素,无法点击的解决方法 2024-04-02
- Ajax实现跨域访问最新解决方案 2023-02-15
- DIV+CSS 清除浮动常用方法总结 2024-03-12
- 一文详解Web Audio浏览器采集麦克风音频数据 2024-02-11
- 详解css透明度之rgba和opacity的区别及兼容 2024-04-07
- 如何通过php在mysql中插入特殊字符并在html页面上显示 2023-10-26