Inheritance and templates in C++ - why are inherited members invisible?(C++ 中的继承和模板——为什么继承的成员不可见?)
问题描述
当一个模板从另一个模板公开继承时,是不是应该可以访问基本的公共方法?
When a template publicly inherits from another template, aren't the base public methods supposed to be accessible?
template <int a>
class Test {
public:
Test() {}
int MyMethod1() { return a; }
};
template <int b>
class Another : public Test<b>
{
public:
Another() {}
void MyMethod2() {
MyMethod1();
}
};
int main()
{
Another<5> a;
a.MyMethod1();
a.MyMethod2();
}
好吧,GCC 在这件事上胡说八道……我一定遗漏了一些非常明显的东西(大脑融化).有帮助吗?
Well, GCC craps out on this... I must be missing something totally obvious (brain melt). Help?
推荐答案
这是有关依赖名称的规则的一部分.Method1
不是 Method2
范围内的依赖名称.所以编译器不会在依赖的基类中查找它.
This is part of the rules concerning dependent names. Method1
is not a dependent name in the scope of Method2
. So the compiler doesn't look it up in dependent base classes.
有两种方法可以解决这个问题:使用 this
或指定基本类型.有关此非常近期的帖子的更多详细信息或在 C++ 常见问题解答.另请注意,您错过了 public 关键字和分号.这是您的代码的固定版本.
There two ways to fix that: Using this
or specifying the base type. More details on this very recent post or at the C++ FAQ. Also notice that you missed the public keyword and a semi-colon. Here's a fixed version of your code.
template <int a>
class Test {
public:
Test() {}
int MyMethod1() { return a; }
};
template <int b>
class Another : public Test<b>
{
public:
Another() {}
void MyMethod2() {
Test<b>::MyMethod1();
}
};
int main()
{
Another<5> a;
a.MyMethod1();
a.MyMethod2();
}
这篇关于C++ 中的继承和模板——为什么继承的成员不可见?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:C++ 中的继承和模板——为什么继承的成员不可见
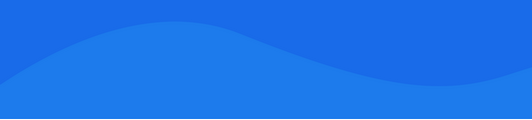
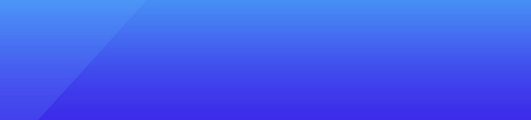
基础教程推荐
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- 从 std::cin 读取密码 2021-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01