Returning non-const reference from a const member function(从 const 成员函数返回非常量引用)
问题描述
为什么返回对指向的成员变量的引用有效,而另一个无效?我知道 const
成员函数应该只返回 const
引用,但为什么对于指针来说这似乎不是真的?
Why does returning the reference to a pointed-to member variable work, but not the other? I know that a const
member function should only return const
references, but why does that not seem true for pointers?
class MyClass
{
private:
int * a;
int b;
public:
MyClass() { a = new int; }
~MyClass() { delete a; }
int & geta(void) const { return *a; } // good?
int & getb(void) const { return b; } // obviously bad
};
int main(void)
{
MyClass m;
m.geta() = 5; //works????
m.getb() = 7; //doesn't compile
return 0;
}
推荐答案
int & geta(void) const { return *a; } // good?
int & getb(void) const { return b; } // obviously bad
在一个 const 函数中,每个数据成员都变成了 const 这样它就不能被修改.int
变为 const int
,int *
变为 int * const
,依此类推.
In a const-function, every data member becomes const in such way that it cannot be modified. int
becomes const int
, int *
becomes int * const
, and so on.
因为在你的第一个函数中 a
的 type 变成了 int * const
,而不是 const int *
>,因此您可以更改数据(可修改):
Since the type of a
in your first function becomes int * const
, as opposed to const int *
, so you can change the data (which is modifiable):
m.geta() = 5; //works, as the data is modifiable
区别:const int*
和 int * const
.
const int*
表示指针是非常量,但指针指向的数据是const.int * const
表示指针是const,但指针指向的数据是非常量.
const int*
means the pointer is non-const, but the data the pointer points to is const.int * const
means the pointer is const, but the data the pointer points to is non-const.
你的第二个函数试图返回 const int &
,因为 b
的 type 变成了 const int
.但是您已经在代码中将实际返回类型提到为 int &
,因此该函数甚至不会 编译(请参阅 this),无论您在 main()
中做什么,因为返回的 type 不匹配.这是修复:
Your second function tries to return const int &
, since the type of b
become const int
. But you've mentioned the actual return type in your code as int &
, so this function would not even compile (see this), irrespective of what you do in main()
, because the return type doesn't match. Here is the fix:
const int & getb(void) const { return b; }
现在它编译正常!.
这篇关于从 const 成员函数返回非常量引用的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:从 const 成员函数返回非常量引用
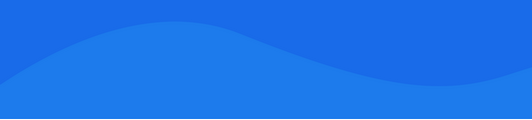
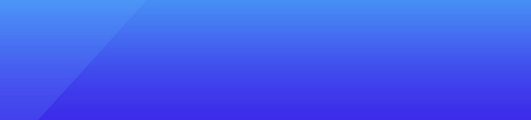
基础教程推荐
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 从 std::cin 读取密码 2021-01-01
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01