What are the operations supported by raw pointer and function pointer in C/C++?(C/C++中的原始指针和函数指针支持哪些操作?)
问题描述
函数指针支持的所有操作与原始指针有何不同?是 > , <, <= , >= 由原始指针支持的运算符,如果有的话有什么用?
What are all operations supported by function pointer differs from raw pointer? Is > , < , <= , >=operators supported by raw pointers if so what is the use?
推荐答案
对于函数指针和对象指针,它们都可以编译,但它们的结果只保证对于同一完整对象的子对象的地址是一致的(您可以比较类或数组的两个成员的地址)以及如果将函数或对象与其自身进行比较.
For both function and object pointers, they compile but their result is only guaranteed to be consistent for addresses to sub-objects of the same complete object (you may compare the addresses of two members of a class or array) and if you compare a function or object against itself.
使用 std::less<>
、std::greater<>
等将适用于任何指针类型,并且会给出一致的结果,即使如果未指定相应内置运算符的结果:
Using std::less<>
, std::greater<>
and so on will work with any pointer type, and will give consistent results, even if the result of the respective built-in operator is unspecified:
void f() { }
void g() { }
int main() {
int a, b;
///// not guaranteed to pass
assert((&a < &b) == (&a < &b));
///// guaranteed to pass
std::less<int*> lss1;
assert(lss1(&a, &b) == lss1(&a, &b));
// note: we don't know whether lss1(&a, &b) is true or false.
// But it's either always true or always false.
////// guaranteed to pass
int c[2];
assert((&c[0] < &c[1]) == (&c[0] < &c[1]));
// in addition, the smaller index compares less:
assert(&c[0] < &c[1]);
///// not guaranteed to pass
assert((&f < &g) == (&f < &g));
///// guaranteed to pass
assert((&g < &g) == (&g < &g));
// in addition, a function compares not less against itself.
assert(!(&g < &g));
///// guaranteed to pass
std::less<void(*)()> lss2;
assert(lss2(&f, &g) == lss2(&f, &g));
// note: same, we don't know whether lss2(&f, &g) is true or false.
///// guaranteed to pass
struct test {
int a;
// no "access:" thing may be between these!
int b;
int c[1];
// likewise here
int d[1];
test() {
assert((&a < &b) == (&a < &b));
assert((&c[0] < &d[0]) == (&c[0] < &d[0]));
// in addition, the previous member compares less:
assert((&a < &b) && (&c[0] < &d[0]));
}
} t;
}
所有这些都应该编译(尽管编译器可以自由地警告它想要的任何代码片段).
Everything of that should compile though (although the compiler is free to warn about any code snippet it wants).
由于函数类型没有sizeof
值,根据指针类型的sizeof
定义的操作将不起作用,包括:
Since function types have no sizeof
value, operations that are defined in terms of sizeof
of the pointee type will not work, these include:
void(*p)() = ...;
// all won't work, since `sizeof (void())` won't work.
// GCC has an extension that treats it as 1 byte, though.
p++; p--; p + n; p - n;
一元 +
适用于任何指针类型,并且只会返回它的值,对于函数指针没有什么特别之处.
The unary +
works on any pointer type, and will just return the value of it, there is nothing special about it for function pointers.
+ p; // works. the result is the address stored in p.
最后注意,指向函数的指针pointer不再是函数指针了:
Finally note that a pointer to a function pointer is not a function pointer anymore:
void (**pp)() = &p;
// all do work, because `sizeof (void(*)())` is defined.
pp++; pp--; pp + n; pp - n;
这篇关于C/C++中的原始指针和函数指针支持哪些操作?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:C/C++中的原始指针和函数指针支持哪些操作?
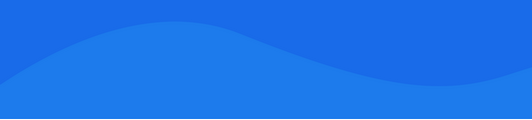
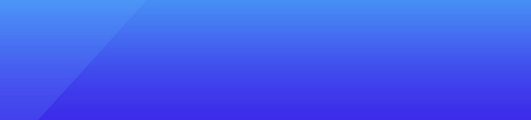
基础教程推荐
- 从 std::cin 读取密码 2021-01-01
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- Windows Media Foundation 录制音频 2021-01-01
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01