SSE reduction of float vector(浮点向量的 SSE 缩减)
问题描述
如何使用 sse 内在函数获取浮点向量的总和元素(减少)?
How can I get sum elements (reduction) of float vector using sse intrinsics?
简单的序列号:
void(float *input, float &result, unsigned int NumElems)
{
result = 0;
for(auto i=0; i<NumElems; ++i)
result += input[i];
}
推荐答案
通常在循环中生成 4 个部分和,然后在循环后对 4 个元素进行水平求和,例如
Typically you generate 4 partial sums in your loop and then just sum horizontally across the 4 elements after the loop, e.g.
#include <cassert>
#include <cstdint>
#include <emmintrin.h>
float vsum(const float *a, int n)
{
float sum;
__m128 vsum = _mm_set1_ps(0.0f);
assert((n & 3) == 0);
assert(((uintptr_t)a & 15) == 0);
for (int i = 0; i < n; i += 4)
{
__m128 v = _mm_load_ps(&a[i]);
vsum = _mm_add_ps(vsum, v);
}
vsum = _mm_hadd_ps(vsum, vsum);
vsum = _mm_hadd_ps(vsum, vsum);
_mm_store_ss(&sum, vsum);
return sum;
}
注意:对于上面的例子,a
必须是16字节对齐,n
必须是4的倍数.如果a
对齐不能保证然后使用 _mm_loadu_ps
代替 _mm_load_ps
.如果 n
不能保证是 4 的倍数,则在函数末尾添加一个标量循环以累积任何剩余元素.
Note: for the above example a
must be 16 byte aligned and n
must be a multiple of 4. If the alignment of a
can not be guaranteed then use _mm_loadu_ps
instead of _mm_load_ps
. If n
is not guaranteed to be a multiple of 4 then add a scalar loop at the end of the function to accumulate any remaining elements.
这篇关于浮点向量的 SSE 缩减的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:浮点向量的 SSE 缩减
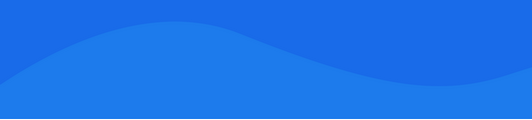
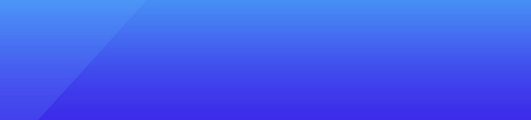
基础教程推荐
- C++,'if' 表达式中的变量声明 2021-01-01
- 如何定义双括号/双迭代器运算符,类似于向量的向量? 2022-01-01
- 运算符重载的基本规则和习语是什么? 2022-10-31
- 如何在 C++ 中处理或避免堆栈溢出 2022-01-01
- C++ 程序在执行 std::string 分配时总是崩溃 2022-01-01
- 您如何将 CreateThread 用于属于类成员的函数? 2021-01-01
- C++ 标准:取消引用 NULL 指针以获取引用? 2021-01-01
- 什么是T&&(双与号)在 C++11 中是什么意思? 2022-11-04
- 设计字符串本地化的最佳方法 2022-01-01
- 调用std::Package_TASK::Get_Future()时可能出现争用情况 2022-12-17