Performance of creating a C++ std::string from an input iterator(从输入迭代器创建 C++ std::string 的性能)
问题描述
我正在做一些非常简单的事情:将整个文本文件从磁盘拖入 std::string
.我当前的代码基本上是这样做的:
I'm doing something really simple: slurping an entire text file from disk into a std::string
. My current code basically does this:
std::ifstream f(filename);
return std::string(std::istreambuf_iterator<char>(f), std::istreambuf_iterator<char>());
这不太可能对程序产生任何性能影响,但我仍然很好奇这是否是一种缓慢的方式.
It's very unlikely that this will ever have any kind of performance impact on the program, but I still got curious whether this is a slow way of doing it.
字符串的构造是否有涉及大量重新分配的风险?使用 seekg()
/tellg()
计算文件大小和 reserve()
会不会更好(也就是更快)> 在读取之前字符串中有那么多空间?
Is there a risk that the construction of the string will involve a lot of reallocations? Would it be better (that is, faster) to use seekg()
/tellg()
to calculate the size of the file and reserve()
that much space in the string before doing the reading?
推荐答案
我对您的实现 (1)、我的 (2) 以及我在 stackoverflow 上找到的其他两个(3 和 4)进行了基准测试.
I benchmarked your implementation(1), mine(2), and two others(3 and 4) that I found on stackoverflow.
结果(100 次运行的平均值;使用 gettimeofday 计时,文件是 40 段 lorem ipsum):
Results (Average of 100 runs; timed using gettimeofday, file was 40 paragraphs of lorem ipsum):
- readFile1:764
- readFile2:104
- readFile3:129
- readFile4:402
实现:
string readFile1(const string &fileName)
{
ifstream f(fileName.c_str());
return string(std::istreambuf_iterator<char>(f),
std::istreambuf_iterator<char>());
}
string readFile2(const string &fileName)
{
ifstream ifs(fileName.c_str(), ios::in | ios::binary | ios::ate);
ifstream::pos_type fileSize = ifs.tellg();
ifs.seekg(0, ios::beg);
vector<char> bytes(fileSize);
ifs.read(&bytes[0], fileSize);
return string(&bytes[0], fileSize);
}
string readFile3(const string &fileName)
{
string data;
ifstream in(fileName.c_str());
getline(in, data, string::traits_type::to_char_type(
string::traits_type::eof()));
return data;
}
string readFile4(const std::string& filename)
{
ifstream file(filename.c_str(), ios::in | ios::binary | ios::ate);
string data;
data.reserve(file.tellg());
file.seekg(0, ios::beg);
data.append(istreambuf_iterator<char>(file.rdbuf()),
istreambuf_iterator<char>());
return data;
}
这篇关于从输入迭代器创建 C++ std::string 的性能的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:从输入迭代器创建 C++ std::string 的性能
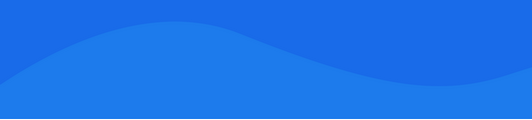
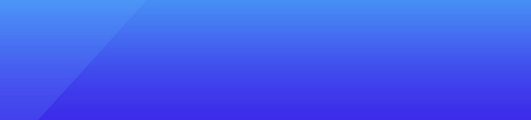
基础教程推荐
- 管理共享内存应该分配多少内存?(助推) 2022-12-07
- Windows Media Foundation 录制音频 2021-01-01
- 使用从字符串中提取的参数调用函数 2022-01-01
- 从 std::cin 读取密码 2021-01-01
- 在 C++ 中循环遍历所有 Lua 全局变量 2021-01-01
- 为 C/C++ 中的项目的 makefile 生成依赖项 2022-01-01
- 如何“在 Finder 中显示"或“在资源管理器中显 2021-01-01
- 如何在不破坏 vtbl 的情况下做相当于 memset(this, ...) 的操作? 2022-01-01
- 为什么语句不能出现在命名空间范围内? 2021-01-01
- 如何使图像调整大小以在 Qt 中缩放? 2021-01-01